DxGrid.DetailExpandButtonDisplayMode Property
Specifies when to display master-detail expand buttons.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[DefaultValue(GridDetailExpandButtonDisplayMode.Auto)]
[Parameter]
public GridDetailExpandButtonDisplayMode DetailExpandButtonDisplayMode { get; set; }
Property Value
Available values:
Name |
Description |
Auto
|
Expand buttons are displayed when the DetailRowTemplate is specified.
|
Never
|
Expand buttons are always hidden.
|
The Grid allows users to create master-detail layouts of any complexity. To enable this functionality, specify the DetailRowTemplate.
A user can click a data row’s expand/collapse button to change a detail row’s expansion state and show/hide detail data.
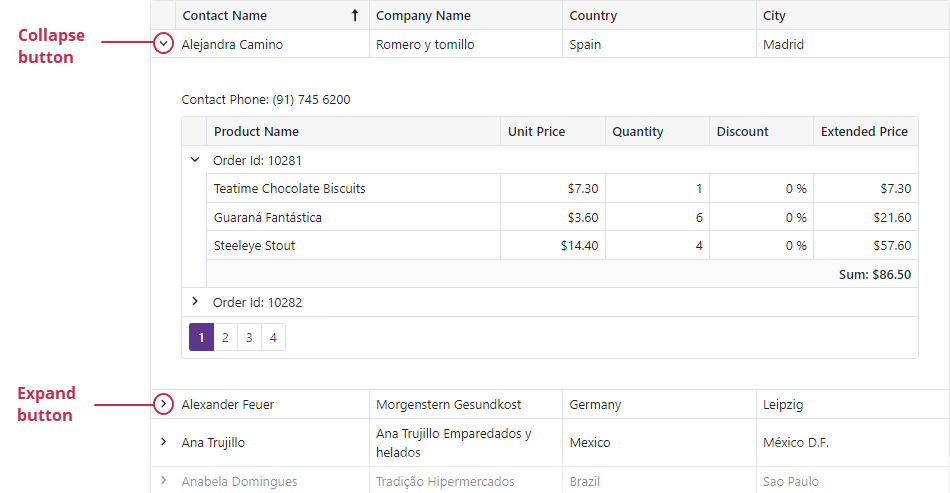
Use the DetailExpandButtonDisplayMode
property to specify when to display master-detail expand buttons in the Grid. The default property value is Auto
. The Grid shows expand buttons if the DetailRowTemplate is specified and the DetailRowDisplayMode is set to Auto
. Otherwise, expand buttons are hidden.
The following example changes DetailExpandButtonDisplayMode
to Never
:
@inject NwindDataService NwindDataService
<DxGrid @ref="Grid"
Data="MasterGridData"
DetailExpandButtonDisplayMode="GridDetailExpandButtonDisplayMode.Never">
<Columns>
<DxGridDataColumn FieldName="ContactName" SortIndex="0" />
<DxGridDataColumn FieldName="CompanyName" />
<DxGridDataColumn FieldName="Country" />
<DxGridDataColumn FieldName="City" />
</Columns>
<DetailRowTemplate>
<Grid_MasterDetail_NestedGrid_DetailContent Customer="(Customer)context.DataItem" />
</DetailRowTemplate>
</DxGrid>
@code {
IGrid Grid { get; set; }
object MasterGridData { get; set; }
protected override async Task OnInitializedAsync() {
MasterGridData = await NwindDataService.GetCustomersAsync();
}
protected override void OnAfterRender(bool firstRender) {
if(firstRender) {
Grid.ExpandDetailRow(0);
}
}
}
@inject NwindDataService NwindDataService
<div class="mb-2">
Contact Phone: @Customer.Phone
</div>
<DxGrid Data="DetailGridData"
PageSize="5"
AutoExpandAllGroupRows="true">
<Columns>
<DxGridDataColumn FieldName="OrderId" DisplayFormat="d" GroupIndex="0" />
<DxGridDataColumn FieldName="ProductName" Width="40%" />
<DxGridDataColumn FieldName="UnitPrice" DisplayFormat="c" />
<DxGridDataColumn FieldName="Quantity" />
<DxGridDataColumn FieldName="Discount" DisplayFormat="p0" />
<DxGridDataColumn FieldName="ExtendedPrice" DisplayFormat="c" />
</Columns>
<GroupSummary>
<DxGridSummaryItem SummaryType="GridSummaryItemType.Sum"
FieldName="ExtendedPrice"
FooterColumnName="ExtendedPrice" />
</GroupSummary>
</DxGrid>
@code {
[Parameter]
public Customer Customer { get; set; }
object DetailGridData { get; set; }
protected override async Task OnInitializedAsync() {
var invoices = await NwindDataService.GetInvoicesAsync();
DetailGridData = invoices
.Where(i => i.CustomerId == Customer.CustomerId)
.ToArray();
}
}
using System;
using System.Collections.Generic;
using System.Runtime.InteropServices;
namespace BlazorDemo.Data.Northwind {
public partial class Customer {
public Customer() {
Orders = new HashSet<Order>();
}
public string CustomerId { get; set; }
public string CompanyName { get; set; }
public string ContactName { get; set; }
public string ContactTitle { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string Phone { get; set; }
public string Fax { get; set; }
public virtual ICollection<Order> Orders { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.Runtime.InteropServices;
namespace BlazorDemo.Data.Northwind {
public class Invoice {
public string ShipName { get; set; }
public string ShipAddress { get; set; }
public string ShipCity { get; set; }
public string ShipRegion { get; set; }
public string ShipPostalCode { get; set; }
public string ShipCountry { get; set; }
public string CustomerId { get; set; }
public string CustomerName { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string Salesperson { get; set; }
public int OrderId { get; set; }
public DateTime? OrderDate { get; set; }
public DateTime? RequiredDate { get; set; }
public DateTime? ShippedDate { get; set; }
public string ShipperName { get; set; }
public int ProductId { get; set; }
public string ProductName { get; set; }
public decimal UnitPrice { get; set; }
public short Quantity { get; set; }
public float Discount { get; set; }
public decimal? ExtendedPrice { get; set; }
public decimal? Freight { get; set; }
}
}
// ...
builder.Services.AddScoped<NwindDataService>();
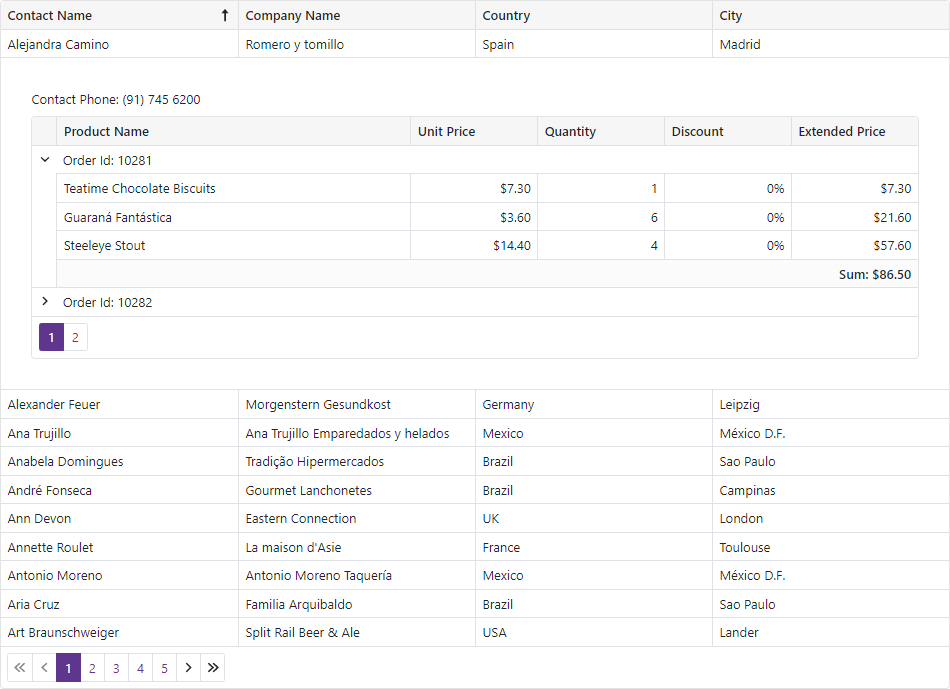
See Also