DxGrid.PagerNavigationMode Property
Specifies how users navigate between Grid pages.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[DefaultValue(PagerNavigationMode.Auto)]
[Parameter]
public PagerNavigationMode PagerNavigationMode { get; set; }
Property Value
Available values:
Name |
Description |
The input box is displayed if the total number of pages is greater than or equal to the SwitchToInputBoxButtonCount (for the Pager) or PagerSwitchToInputBoxButtonCount (for the Grid or TreeList), or the pager is shown on small devices. Otherwise, numeric buttons are displayed.
|
Users can type a page number in the displayed Go to Page input box to jump to the corresponding page.
|
Users can click numeric buttons to navigate between pages.
|
The Grid splits a large amount of data rows across multiple pages and displays the pager to enable data navigation. Use the PagerNavigationMode
property to specify how users navigate between pages. The following values are available:
- PagerNavigationMode.InputBox
The pager contains an input box in which a user can type a page number.
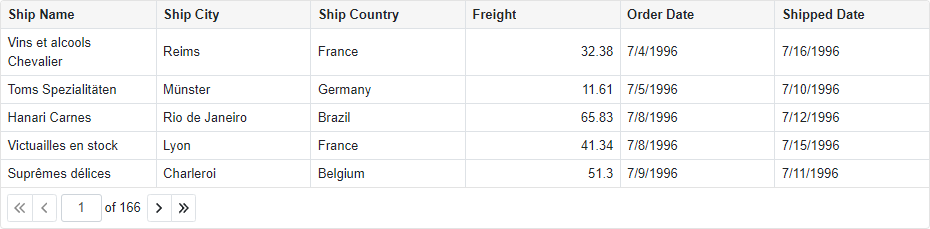
- PagerNavigationMode.NumericButtons
The pager contains numeric buttons.
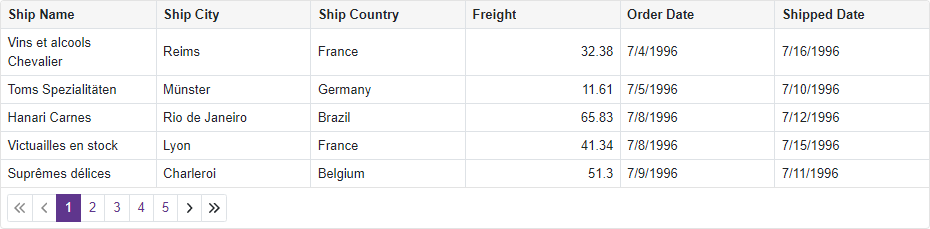
- PagerNavigationMode.Auto (Default)
- The pager switches from numeric buttons to the input box in the following cases:
The following example always displays numeric buttons in the pager:
@using Microsoft.EntityFrameworkCore
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
@implements IDisposable
<DxGrid Data="@Data"
PagerNavigationMode="PagerNavigationMode.NumericButtons">
<Columns>
<DxGridDataColumn FieldName="ShipName" />
<DxGridDataColumn FieldName="ShipCity" />
<DxGridDataColumn FieldName="ShipCountry" />
<DxGridDataColumn FieldName="Freight" />
<DxGridDataColumn FieldName="OrderDate" />
<DxGridDataColumn FieldName="ShippedDate" />
</Columns>
</DxGrid>
@code {
object Data { get; set; }
NorthwindContext Northwind { get; set; }
protected override void OnInitialized() {
Northwind = NorthwindContextFactory.CreateDbContext();
Data = Northwind.Orders.ToList();
}
public void Dispose() {
Northwind?.Dispose();
}
}
using System;
using System.Collections.Generic;
#nullable disable
namespace Grid.Northwind {
public partial class Order {
public Order() {
OrderDetails = new HashSet<OrderDetail>();
}
public int OrderId { get; set; }
public string CustomerId { get; set; }
public int? EmployeeId { get; set; }
public DateTime? OrderDate { get; set; }
public DateTime? RequiredDate { get; set; }
public DateTime? ShippedDate { get; set; }
public int? ShipVia { get; set; }
public decimal? Freight { get; set; }
public string ShipName { get; set; }
public string ShipAddress { get; set; }
public string ShipCity { get; set; }
public string ShipRegion { get; set; }
public string ShipPostalCode { get; set; }
public string ShipCountry { get; set; }
public virtual Customer Customer { get; set; }
public virtual Shipper ShipViaNavigation { get; set; }
public virtual ICollection<OrderDetail> OrderDetails { get; set; }
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Order> Orders { get; set; }
// ...
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder) {
modelBuilder.HasAnnotation("Relational:Collation", "SQL_Latin1_General_CP1_CI_AS");
// ...
modelBuilder.Entity<Order>(entity => {
entity.HasIndex(e => e.CustomerId, "CustomerID");
entity.HasIndex(e => e.CustomerId, "CustomersOrders");
entity.HasIndex(e => e.EmployeeId, "EmployeeID");
entity.HasIndex(e => e.EmployeeId, "EmployeesOrders");
entity.HasIndex(e => e.OrderDate, "OrderDate");
entity.HasIndex(e => e.ShipPostalCode, "ShipPostalCode");
entity.HasIndex(e => e.ShippedDate, "ShippedDate");
entity.HasIndex(e => e.ShipVia, "ShippersOrders");
entity.Property(e => e.OrderId).HasColumnName("OrderID");
entity.Property(e => e.CustomerId)
.HasMaxLength(5)
.HasColumnName("CustomerID")
.IsFixedLength(true);
entity.Property(e => e.EmployeeId).HasColumnName("EmployeeID");
entity.Property(e => e.Freight)
.HasColumnType("money")
.HasDefaultValueSql("((0))");
entity.Property(e => e.OrderDate).HasColumnType("datetime");
entity.Property(e => e.RequiredDate).HasColumnType("datetime");
entity.Property(e => e.ShipAddress).HasMaxLength(60);
entity.Property(e => e.ShipCity).HasMaxLength(15);
entity.Property(e => e.ShipCountry).HasMaxLength(15);
entity.Property(e => e.ShipName).HasMaxLength(40);
entity.Property(e => e.ShipPostalCode).HasMaxLength(10);
entity.Property(e => e.ShipRegion).HasMaxLength(15);
entity.Property(e => e.ShippedDate).HasColumnType("datetime");
entity.HasOne(d => d.Customer)
.WithMany(p => p.Orders)
.HasForeignKey(d => d.CustomerId)
.HasConstraintName("FK_Orders_Customers");
entity.HasOne(d => d.ShipViaNavigation)
.WithMany(p => p.Orders)
.HasForeignKey(d => d.ShipVia)
.HasConstraintName("FK_Orders_Shippers");
});
// ...
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
using Microsoft.EntityFrameworkCore;
// ...
builder.Services.AddDbContextFactory<NorthwindContext>((sp, options) => {
var env = sp.GetRequiredService<IWebHostEnvironment>();
var dbPath = Path.Combine(env.ContentRootPath, "Northwind-5e44b51f.mdf");
options.UseSqlServer("Server=(localdb)\\MSSQLLocalDB;Integrated Security=true;AttachDbFileName=" + dbPath);
});
When the pager contains numeric buttons, you can also define the following properties:
- PagerVisibleNumericButtonCount
- Specifies the maximum number of numeric buttons displayed in the pager.
- PagerAutoHideNavButtons
- Specifies whether the navigation buttons are hidden when all numeric buttons are displayed.
The pager can also contain the page size selector.
Run Demo: Data Grid - Paging
For more information about paging in the Grid component, refer to the following topic: Paging in Blazor Grid.
See Also