DxGrid.HighlightRowOnHover Property
Specifies whether to highlight a data row when a user hovers the mouse pointer over it.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[DefaultValue(false)]
[Parameter]
public bool HighlightRowOnHover { get; set; }
Property Value
Type |
Default |
Description |
Boolean |
false |
true to highlight data rows on hover; otherwise, false .
|
The following example highlights data rows in the Grid component when a user hovers the mouse pointer over them:
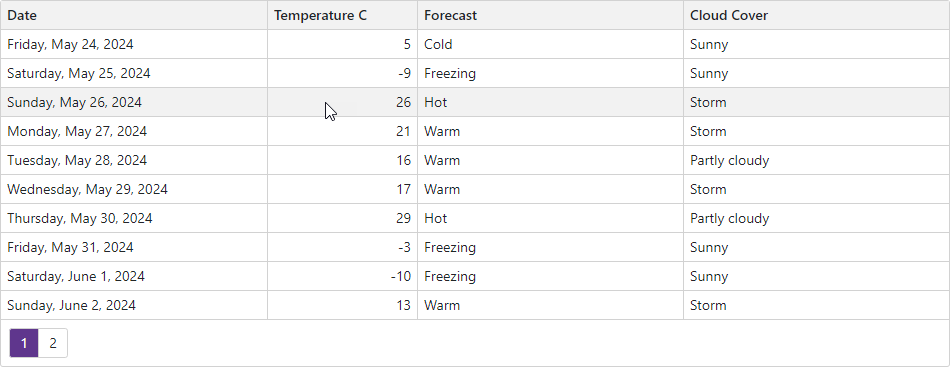
@inject WeatherForecastService ForecastService
<DxGrid Data="@Data" HighlightRowOnHover="true">
<Columns>
<DxGridDataColumn FieldName="Date" DisplayFormat="D" />
<DxGridDataColumn FieldName="TemperatureC" Width="150px" />
<DxGridDataColumn FieldName="Forecast" />
<DxGridDataColumn FieldName="CloudCover" />
</Columns>
</DxGrid>
@code {
object Data { get; set; }
protected override void OnInitialized() {
Data = ForecastService.GetForecast();
}
}
using System;
public class WeatherForecast {
public DateTime Date { get; set; }
public int TemperatureC { get; set; }
public double TemperatureF => Math.Round((TemperatureC * 1.8 + 32), 2);
public string Forecast { get; set; }
public string CloudCover { get; set; }
public bool Precipitation { get; set; }
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading;
using System.Threading.Tasks;
public class WeatherForecastService {
private List<WeatherForecast> Forecast { get; set; }
private static string[] CloudCover = new[] {
"Sunny", "Partly cloudy", "Cloudy", "Storm"
};
Tuple<int, string>[] ConditionsForForecast = new Tuple<int, string>[] {
Tuple.Create( 22 , "Hot"),
Tuple.Create( 13 , "Warm"),
Tuple.Create( 0 , "Cold"),
Tuple.Create( -10 , "Freezing")
};
public WeatherForecastService() {
Forecast = CreateForecast();
}
private List<WeatherForecast> CreateForecast() {
var rng = new Random();
DateTime startDate = DateTime.Now;
return Enumerable.Range(1, 15).Select(index => {
var temperatureC = rng.Next(-10, 30);
return new WeatherForecast {
Date = startDate.AddDays(index),
TemperatureC = temperatureC,
CloudCover = CloudCover[rng.Next(0, 4)],
Precipitation = Convert.ToBoolean(rng.Next(0, 2)),
Forecast = ConditionsForForecast.First(c => c.Item1 <= temperatureC).Item2
};
}).ToList();
}
public IEnumerable<WeatherForecast> GetForecast() {
return Forecast.ToArray();
}
// ...
}
// ...
builder.Services.AddSingleton<WeatherForecastService>();
See Also