GridColumnHeaderCaptionTemplateContext Class
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.2.dll
NuGet Package:
DevExpress.Blazor
Declaration
public class GridColumnHeaderCaptionTemplateContext :
GridColumnHeaderCaptionTemplateContextBase
The DxGrid.ColumnHeaderCaptionTemplate and DxGridColumn.HeaderCaptionTemplate accept a GridColumnHeaderCaptionTemplateContext
object as the context
parameter. You can use the parameter’s members to get information about the caption of the column header.
The following example shows the tooltip when a user hovers the mouse pointer over any column caption:
@using Microsoft.EntityFrameworkCore
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
@implements IDisposable
<DxGrid Data="@Data">
<Columns>
<DxGridDataColumn FieldName="FirstName" />
<DxGridDataColumn FieldName="LastName" />
<DxGridDataColumn FieldName="Title" />
<DxGridDataColumn FieldName="BirthDate" />
<DxGridDataColumn FieldName="HireDate" />
</Columns>
<ColumnHeaderCaptionTemplate>
<span title="Click the header to sort data by this column. Drag and drop the header to change the column's position">
@context.Caption</span>
</ColumnHeaderCaptionTemplate>
</DxGrid>
@code {
IEnumerable<Employee> Data { get; set; }
NorthwindContext Northwind { get; set; }
Employee CurrentEmployee { get; set; }
protected override void OnInitialized() {
Northwind = NorthwindContextFactory.CreateDbContext();
Data = Northwind.Employees
.ToList();
}
public void Dispose() {
Northwind?.Dispose();
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
namespace Grid.Northwind {
public partial class Employee {
public int EmployeeId { get; set; }
[Required]
public string LastName { get; set; }
[Required]
public string FirstName { get; set; }
[Required]
public string Title { get; set; }
public string TitleOfCourtesy { get; set; }
public Nullable<System.DateTime> BirthDate { get; set; }
[Required]
[Range(typeof(DateTime), "1/1/2000", "1/1/2020",
ErrorMessage = "HireDate must be between {1:d} and {2:d}")]
public Nullable<System.DateTime> HireDate { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string HomePhone { get; set; }
public string Extension { get; set; }
public byte[] Photo { get; set; }
public string Notes { get; set; }
public Nullable<int> ReportsTo { get; set; }
public string PhotoPath { get; set; }
public virtual ICollection<Order> Orders { get; set; }
public string Text => $"{FirstName} {LastName} ({Title})";
public string ImageFileName => $"Employees/{EmployeeId}.jpg";
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Employee> Employees { get; set; }
// ...
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
// ...
protected override void OnModelCreating(ModelBuilder modelBuilder) {
// ...
modelBuilder.Entity<Employee>(entity => {
entity.HasIndex(e => e.EmployeeId, "EmployeeId");
entity.HasIndex(e => e.LastName, "LastName");
entity.HasIndex(e => e.FirstName, "FirstName");
entity.HasIndex(e => e.Title, "Title");
entity.HasIndex(e => e.BirthDate, "BirthDate");
entity.HasIndex(e => e.HireDate, "HireDate");
entity.HasIndex(e => e.Notes, "Notes");
});
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
using Microsoft.EntityFrameworkCore;
// ...
builder.Services.AddDbContextFactory<NorthwindContext>((sp, options) => {
var env = sp.GetRequiredService<IWebHostEnvironment>();
var dbPath = Path.Combine(env.ContentRootPath, "Northwind-5e44b51f.mdf");
options.UseSqlServer("Server=(localdb)\\MSSQLLocalDB;Integrated Security=true;AttachDbFileName=" + dbPath);
});
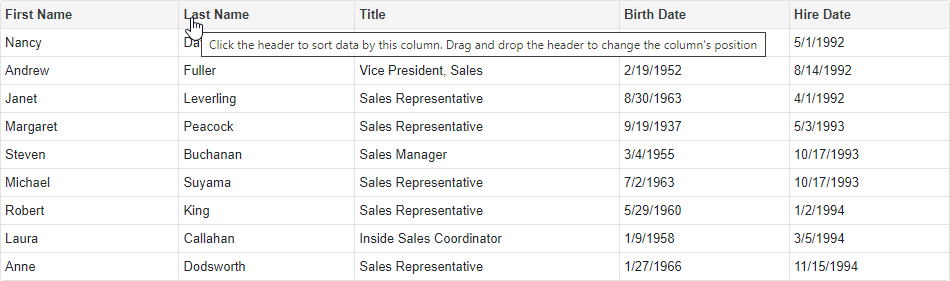
Run Demo: Grid - Column Templates
Inheritance
ObjectDevExpress.Blazor.Internal.GridTemplateContextBaseDevExpress.Blazor.Internal.GridColumnHeaderCaptionTemplateContextBaseGridColumnHeaderCaptionTemplateContext
See Also