DxGrid.DeselectAllOnPage() Method
Deselects all rows on the current visible page.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v23.2.dll
NuGet Package:
DevExpress.Blazor
Declaration
public void DeselectAllOnPage()
The following methods allow you to manage Grid selection:
Call the DeselectAllOnPage
method to remove all rows on the current page from selection. To add these rows to selection, call the SelectAllOnPage method.
Note
The Grid displays all rows on one page if the ShowAllRows or VirtualScrollingEnabled property is enabled. In this case, the DeselectAllOnPage
method deselects all grid rows not hidden by the filter.
To access data items that correspond to selected rows, implement two-way binding for the SelectedDataItems property or handle the SelectedDataItemsChanged event.
@using Microsoft.EntityFrameworkCore
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
@implements IDisposable
<DxGrid Data="GridDataSource"
AllowSelectRowByClick="true"
@bind-SelectedDataItems="@SelectedDataItems"
KeyFieldName="ProductId"
@ref="MyGrid">
<Columns>
<DxGridDataColumn FieldName="ProductName" />
<DxGridDataColumn FieldName="UnitPrice" />
<DxGridDataColumn FieldName="QuantityPerUnit" />
<DxGridDataColumn FieldName="UnitsInStock" />
</Columns>
</DxGrid>
<br />
<DxButton Click="() => MyGrid.SelectAllOnPage()">Select All on Page</DxButton>
<DxButton Click="() => MyGrid.DeselectAllOnPage()">Deselect All on Page</DxButton>
@code {
IEnumerable<object> GridDataSource { get; set; }
NorthwindContext Northwind { get; set; }
IReadOnlyList<object> SelectedDataItems { get; set; }
IGrid MyGrid { get; set; }
protected override void OnInitialized() {
Northwind = NorthwindContextFactory.CreateDbContext();
GridDataSource = Northwind.Products
.ToList();
SelectedDataItems = GridDataSource.Skip(1).Take(2).ToList();
}
public void Dispose() {
Northwind?.Dispose();
}
}
using System;
using System.Collections.Generic;
#nullable disable
namespace Grid.Northwind {
public partial class Product {
public Product() {
OrderDetails = new HashSet<OrderDetail>();
}
public int ProductId { get; set; }
public string ProductName { get; set; }
public int? SupplierId { get; set; }
public int? CategoryId { get; set; }
public string QuantityPerUnit { get; set; }
public decimal? UnitPrice { get; set; }
public short? UnitsInStock { get; set; }
public short? UnitsOnOrder { get; set; }
public short? ReorderLevel { get; set; }
public bool Discontinued { get; set; }
public virtual Category Category { get; set; }
public virtual Supplier Supplier { get; set; }
public virtual ICollection<OrderDetail> OrderDetails { get; set; }
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Product> Products { get; set; }
// ...
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder) {
// ...
modelBuilder.Entity<Product>(entity => {
entity.HasIndex(e => e.CategoryId, "CategoriesProducts");
entity.HasIndex(e => e.CategoryId, "CategoryID");
entity.HasIndex(e => e.ProductName, "ProductName");
entity.HasIndex(e => e.SupplierId, "SupplierID");
entity.HasIndex(e => e.SupplierId, "SuppliersProducts");
entity.Property(e => e.ProductId).HasColumnName("ProductID");
entity.Property(e => e.CategoryId).HasColumnName("CategoryID");
entity.Property(e => e.ProductName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.QuantityPerUnit).HasMaxLength(20);
entity.Property(e => e.ReorderLevel).HasDefaultValueSql("((0))");
entity.Property(e => e.SupplierId).HasColumnName("SupplierID");
entity.Property(e => e.UnitPrice)
.HasColumnType("money")
.HasDefaultValueSql("((0))");
entity.Property(e => e.UnitsInStock).HasDefaultValueSql("((0))");
entity.Property(e => e.UnitsOnOrder).HasDefaultValueSql("((0))");
entity.HasOne(d => d.Category)
.WithMany(p => p.Products)
.HasForeignKey(d => d.CategoryId)
.HasConstraintName("FK_Products_Categories");
entity.HasOne(d => d.Supplier)
.WithMany(p => p.Products)
.HasForeignKey(d => d.SupplierId)
.HasConstraintName("FK_Products_Suppliers");
});
// ...
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
using Microsoft.EntityFrameworkCore;
// ...
builder.Services.AddDbContextFactory<NorthwindContext>((sp, options) => {
var env = sp.GetRequiredService<IWebHostEnvironment>();
var dbPath = Path.Combine(env.ContentRootPath, "Northwind-5e44b51f.mdf");
options.UseSqlServer("Server=(localdb)\\MSSQLLocalDB;Integrated Security=true;AttachDbFileName=" + dbPath);
});
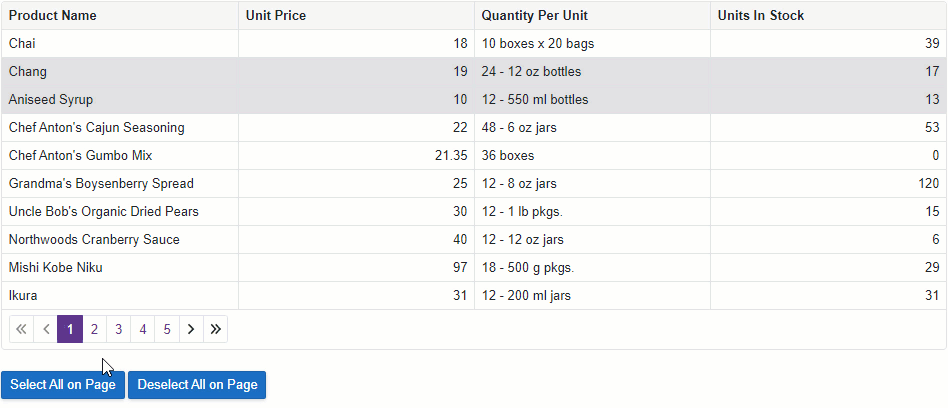
Run Demo: Data Grid - Selection API
For more information about selection in the Grid component, refer to the following topic: Selection and Focus in Blazor Grid.
See Also