DxGridDataColumn.DataRowEditorVisible Property
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[DefaultValue(true)]
[Parameter]
public bool DataRowEditorVisible { get; set; }
Property Value
Type |
Default |
Description |
Boolean |
true |
true to render the column editor; false to hide the editor.
|
The Grid component generates editors for columns based on associated data types and automatically displays these editors in the filter row and in data rows during edit operations. Set the column’s DataRowEditorVisible
property to false
to render display text instead of the editor in the column edit cell. To hide the filter row editor, set the FilterRowEditorVisible property to false
.
The GetEditor(String) method allows you to get a column editor and place it in the edit or pop-up edit form. If the column’s DataRowEditorVisible
property is set to false
, the method returns an empty render fragment instead of the editor.
The following code snippet hides the editor assosiated with the Product ID column:
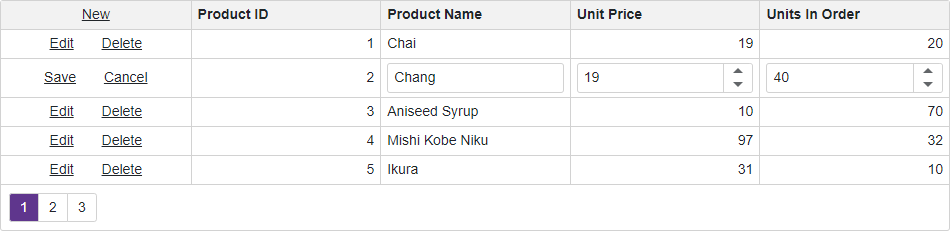
@inject ProductService ProductData
<DxGrid Data="@products"
EditMode="GridEditMode.EditRow"
EditorRenderMode="GridEditorRenderMode.Detached">
<Columns>
<DxGridCommandColumn />
<DxGridDataColumn FieldName="ProductID" DataRowEditorVisible="false" />
<DxGridDataColumn FieldName="ProductName" />
<DxGridDataColumn FieldName="UnitPrice" />
<DxGridDataColumn FieldName="UnitsInOrder" />
</Columns>
</DxGrid>
@code {
private Product[]? products;
protected override async Task OnInitializedAsync() {
products = await ProductData.GetData();
}
}
public class ProductService {
public Task<Product[]> GetData() {
List<Product> products = new List<Product>();
products.Add(new Product() { ProductID = 1, ProductName = "Chai", UnitPrice = 19, UnitsInOrder = 20, Discontinued = true });
products.Add(new Product() { ProductID = 2, ProductName = "Chang", UnitPrice = 19, UnitsInOrder = 40, Discontinued = true });
products.Add(new Product() { ProductID = 3, ProductName = "Aniseed Syrup", UnitPrice = 10, UnitsInOrder = 70, Discontinued = true });
products.Add(new Product() { ProductID = 4, ProductName = "Mishi Kobe Niku", UnitPrice = 97, UnitsInOrder = 32, Discontinued = false });
products.Add(new Product() { ProductID = 5, ProductName = "Ikura", UnitPrice = 31, UnitsInOrder = 10, Discontinued = false });
products.Add(new Product() { ProductID = 6, ProductName = "Chef Anton's Cajun Seasoning", UnitPrice = 22, UnitsInOrder = 12, Discontinued = false });
products.Add(new Product() { ProductID = 7, ProductName = "Chef Anton's Gumbo Mix", UnitPrice = 21.35m, UnitsInOrder = 16, Discontinued = true });
products.Add(new Product() { ProductID = 8, ProductName = "Grandma's Boysenberry Spread", UnitPrice = 25, UnitsInOrder = 20, Discontinued = true });
products.Add(new Product() { ProductID = 9, ProductName = "Uncle Bob's Organic Dried Pears", UnitPrice = 30, UnitsInOrder = 24, Discontinued = false });
products.Add(new Product() { ProductID = 10, ProductName = "Northwoods Cranberry Sauce", UnitPrice = 40, UnitsInOrder = 18, Discontinued = false });
products.Add(new Product() { ProductID = 11, ProductName = "Queso Cabrales", UnitPrice = 21, UnitsInOrder = 30 });
products.Add(new Product() { ProductID = 12, ProductName = "Queso Manchego La Pastora", UnitPrice = 38, UnitsInOrder = 10 });
return Task.FromResult(products.ToArray());
}
}
public class Product {
public int ProductID { get; set; }
public string ProductName { get; set; }
public decimal? UnitPrice { get; set; }
public short? UnitsInOrder { get; set; }
public bool Discontinued { get; set; }
}
See Also