DxListBox<TData, TValue>.SearchTextParseMode Property
Specifies how the list box treats search words.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.2.dll
NuGet Package:
DevExpress.Blazor
Declaration
[DefaultValue(ListSearchTextParseMode.Default)]
[Parameter]
public ListSearchTextParseMode SearchTextParseMode { get; set; }
Property Value
Available values:
Name |
Description |
Default
|
The ExactMatch is used by default. The search words are not treated separately. Only records that match the search text exactly are shown.
|
GroupWordsByAnd
|
The search words are grouped as individual conditions by the AND logical operator. Only records that match all the conditions are shown.
|
GroupWordsByOr
|
The search words are treated as individual conditions grouped by the OR logical operator. Records that match at least one of these conditions are shown.
|
ExactMatch
|
The search words are not treated separately. Only records that match the search text exactly are shown.
|
The search feature allows users to locate and highlight the search text in List Box data. If the search text contains multiple words separated by space characters, the words can be treated as a single condition or as individual conditions grouped by the Or
or And
operator.
Use the SearchTextParseMode
property to specify how the List Box component treats search words.
The following code snippet sets the SearchTextParseMode
property to the GroupWordsByOr
value:
<DxListBox TData=Person TValue=Person Data="Staff.DataSource"
ShowCheckboxes="true"
SearchTextParseMode="ListSearchTextParseMode.GroupWordsByOr"
SearchText="sandra electronics"
SelectionMode="@ListBoxSelectionMode.Multiple">
<Columns>
<DxListEditorColumn FieldName="FirstName"></DxListEditorColumn>
<DxListEditorColumn FieldName="LastName"></DxListEditorColumn>
<DxListEditorColumn FieldName="Department"></DxListEditorColumn>
</Columns>
</DxListBox>
namespace StaffData {
public static class Staff {
private static readonly Lazy<List<Person>> dataSource = new Lazy<List<Person>>(() => {
var dataSource = new List<Person>() {
new Person() { Id= 0 , FirstName="John", LastName="Heart", Department=Department.Electronics },
new Person() { Id= 1 , FirstName="Samantha", LastName="Bright", Department=Department.Motors },
new Person() { Id= 2 , FirstName="Arthur", LastName="Miller", Department=Department.Software },
new Person() { Id= 3 , FirstName="Robert", LastName="Reagan", Department=Department.Electronics },
new Person() { Id= 4 , FirstName="Greta", LastName="Sims", Department=Department.Motors },
new Person() { Id= 5 , FirstName="Brett", LastName="Wade", Department=Department.Software },
new Person() { Id= 6 , FirstName="Sandra", LastName="Johnson", Department=Department.Electronics },
new Person() { Id= 7 , FirstName="Edward", LastName="Holmes", Department=Department.Motors },
new Person() { Id= 8 , FirstName="Barbara", LastName="Banks", Department=Department.Software },
new Person() { Id= 9 , FirstName="Kevin", LastName="Carter", Department=Department.Electronics },
new Person() { Id= 10, FirstName="Cynthia", LastName="Stanwick", Department=Department.Motors },
new Person() { Id= 11, FirstName="Sam", LastName="Hill", Department=Department.Electronics }};
return dataSource;
});
public static List<Person> DataSource { get { return dataSource.Value; } }
}
public class Person {
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public Department Department { get; set; }
public string Text => $"{FirstName} {LastName} ({Department} Dept.)";
public override bool Equals(object obj) {
if (obj is Person typedObj) {
return (this.Id == typedObj.Id) && (this.FirstName == typedObj.FirstName) && (this.LastName == typedObj.LastName)
&& (this.Department == typedObj.Department);
}
return base.Equals(obj);
}
}
public enum Department { Motors, Electronics, Software }
}
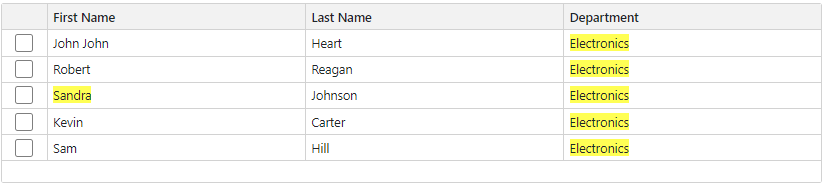
Search Syntax
Search text can include special characters that allow users to create composite criteria. Refer to the following section for additional information: Search Syntax
Implements
DevExpress.Blazor.IListBox<TData, TValue>.SearchTextParseMode
See Also