DxGridCommandColumn.DeleteButtonVisible Property
Specifies whether the command column displays Delete buttons.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[DefaultValue(true)]
[Parameter]
public bool DeleteButtonVisible { get; set; }
Property Value
Type |
Default |
Description |
Boolean |
true |
true to display Delete buttons; otherwise, false .
|
The command column displays predefined New, Edit, and Delete command buttons for data rows in display mode. In EditRow
and EditCell
edit modes, this column displays Save and Cancel buttons for the edited row. In the filter row, the column displays the Clear button.
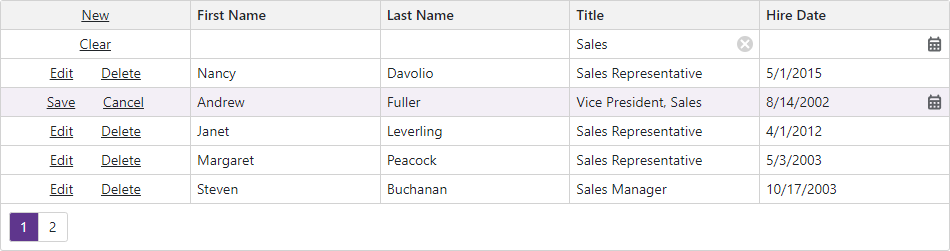
The following example hides New and Delete command buttons:
@using Microsoft.EntityFrameworkCore
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
@implements IDisposable
<DxGrid Data="GridDataSource"
EditModelSaving="OnEditModelSaving"
KeyFieldName="EmployeeId"
ShowFilterRow="true"
EditMode="GridEditMode.EditRow">
<Columns>
<DxGridCommandColumn NewButtonVisible="false" DeleteButtonVisible="false" />
<DxGridDataColumn FieldName="FirstName" />
<DxGridDataColumn FieldName="LastName" />
<DxGridDataColumn FieldName="Title" />
<DxGridDataColumn FieldName="HireDate" />
</Columns>
</DxGrid>
@code {
IEnumerable<object> GridDataSource { get; set; }
NorthwindContext Northwind { get; set; }
protected override async Task OnInitializedAsync() {
Northwind = NorthwindContextFactory.CreateDbContext();
GridDataSource = await Northwind.Employees.ToListAsync();
}
async Task OnEditModelSaving(GridEditModelSavingEventArgs e) {
var editModel = (Employee)e.EditModel;
// Assign changes from the edit model to the data item.
if (e.IsNew)
await Northwind.AddAsync(editModel);
else
e.CopyChangesToDataItem();
// Post changes to the database.
await Northwind.SaveChangesAsync();
// Reload the entire Grid.
GridDataSource = await Northwind.Employees.ToListAsync();
}
public void Dispose() {
Northwind?.Dispose();
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
namespace Grid.Northwind {
public partial class Employee {
public int EmployeeId { get; set; }
[Required]
public string LastName { get; set; }
[Required]
public string FirstName { get; set; }
[Required]
public string Title { get; set; }
public string TitleOfCourtesy { get; set; }
public Nullable<System.DateTime> BirthDate { get; set; }
[Required]
[Range(typeof(DateTime), "1/1/2000", "1/1/2020",
ErrorMessage = "HireDate must be between {1:d} and {2:d}")]
public Nullable<System.DateTime> HireDate { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string HomePhone { get; set; }
public string Extension { get; set; }
public byte[] Photo { get; set; }
public string Notes { get; set; }
public Nullable<int> ReportsTo { get; set; }
public string PhotoPath { get; set; }
public virtual ICollection<Order> Orders { get; set; }
public string Text => $"{FirstName} {LastName} ({Title})";
public string ImageFileName => $"Employees/{EmployeeId}.jpg";
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Employee> Employees { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder) {
modelBuilder.HasAnnotation("Relational:Collation", "SQL_Latin1_General_CP1_CI_AS");
// ...
modelBuilder.Entity<Employee>(entity => {
entity.HasIndex(e => e.EmployeeId, "EmployeeId");
entity.HasIndex(e => e.LastName, "LastName");
entity.HasIndex(e => e.FirstName, "FirstName");
entity.HasIndex(e => e.Title, "Title");
entity.HasIndex(e => e.BirthDate, "BirthDate");
entity.HasIndex(e => e.HireDate, "HireDate");
entity.HasIndex(e => e.Notes, "Notes");
});
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
using Microsoft.EntityFrameworkCore;
// ...
builder.Services.AddDbContextFactory<NorthwindContext>((sp, options) => {
var env = sp.GetRequiredService<IWebHostEnvironment>();
var dbPath = Path.Combine(env.ContentRootPath, "Northwind-5e44b51f.mdf");
options.UseSqlServer("Server=(localdb)\\MSSQLLocalDB;Integrated Security=true;AttachDbFileName=" + dbPath);
});
For information on how to enable data editing, refer to the following topic: Editing and Validation in Blazor Grid.
See Also