DxGridCommandColumn.FilterRowCellTemplate Property
Specifies a template used to display the command column’s filter row cell.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[Parameter]
public RenderFragment<GridCommandColumnFilterRowCellTemplateContext> FilterRowCellTemplate { get; set; }
Property Value
The command column displays predefined New, Edit, and Delete command buttons for data rows in display mode. In EditRow
and EditCell
edit modes, this column displays Save and Cancel buttons for the edited row. In the filter row, the column displays the Clear button.
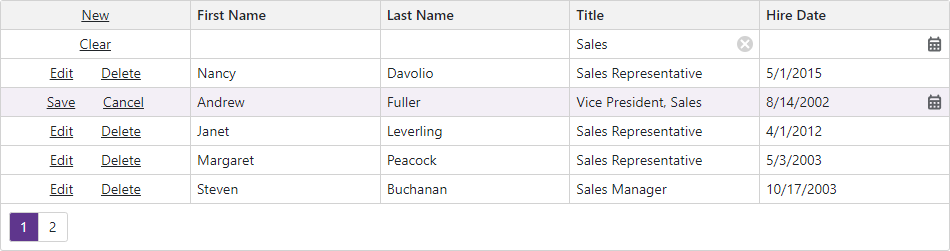
Read Tutorial: Edit Data in Blazor Grid Read Tutorial: Filter Row in Blazor Grid
Define the FilterRowCellTemplate
to display custom content in the command column’s filter row cell. The template accepts a GridDataColumnFilterRowCellTemplateContext object as the context
parameter. You can use this parameter to access CommandColumn and Grid objects and obtain information about their states.
The following example displays the custom Clear button in the command column’s filter row cell. The button’s click handler calls the ClearFilter() method.
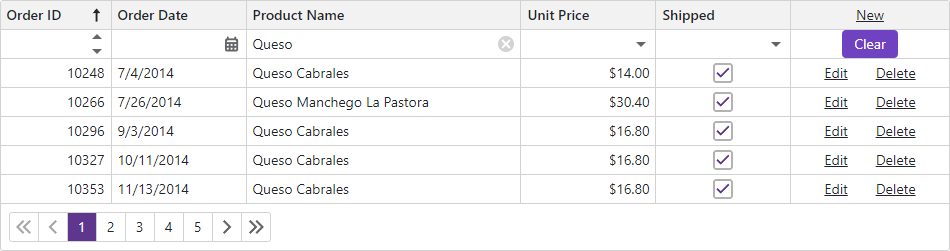
@using Microsoft.EntityFrameworkCore
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
@implements IDisposable
<DxGrid Data="@Data"
ShowFilterRow="true"
@ref="MyGrid">
<Columns>
<DxGridDataColumn FieldName="OrderId" Caption="Order ID" DisplayFormat="d"/>
<DxGridDataColumn FieldName="OrderDate" DisplayFormat="d" />
<DxGridDataColumn FieldName="ProductName" FilterRowValue='"Queso"'
FilterRowOperatorType="GridFilterRowOperatorType.Contains" />
<DxGridDataColumn FieldName="UnitPrice" DisplayFormat="c2" />
<DxGridDataColumn FieldName="Shipped" UnboundType="GridUnboundColumnType.Boolean"
UnboundExpression="[ShippedDate] <> Null" />
<DxGridCommandColumn>
<FilterRowCellTemplate>
<DxButton Text="Clear" Click="ClearFilter"></DxButton>
</FilterRowCellTemplate>
</DxGridCommandColumn>
</Columns>
</DxGrid>
@code {
object Data { get; set; }
NorthwindContext Northwind { get; set; }
IGrid MyGrid { get; set; }
protected override void OnInitialized() {
Northwind = NorthwindContextFactory.CreateDbContext();
Data = Northwind.Invoices
.ToList();
}
void ClearFilter() {
MyGrid.ClearFilter();
}
public void Dispose() {
Northwind?.Dispose();
}
}
using System;
#nullable disable
namespace Grid.Northwind {
public partial class Invoice {
public string ShipName { get; set; }
public string ShipAddress { get; set; }
public string ShipCity { get; set; }
public string ShipRegion { get; set; }
public string ShipPostalCode { get; set; }
public string ShipCountry { get; set; }
public string CustomerId { get; set; }
public string CustomerName { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string Salesperson { get; set; }
public int OrderId { get; set; }
public DateTime? OrderDate { get; set; }
public DateTime? RequiredDate { get; set; }
public DateTime? ShippedDate { get; set; }
public string ShipperName { get; set; }
public int ProductId { get; set; }
public string ProductName { get; set; }
public decimal UnitPrice { get; set; }
public short Quantity { get; set; }
public float Discount { get; set; }
public decimal? ExtendedPrice { get; set; }
public decimal? Freight { get; set; }
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Invoice> Invoices { get; set; }
// ...
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder) {
modelBuilder.HasAnnotation("Relational:Collation", "SQL_Latin1_General_CP1_CI_AS");
// ...
modelBuilder.Entity<Invoice>(entity => {
entity.HasNoKey();
entity.ToView("Invoices");
entity.Property(e => e.Address).HasMaxLength(60);
entity.Property(e => e.City).HasMaxLength(15);
entity.Property(e => e.Country).HasMaxLength(15);
entity.Property(e => e.CustomerId)
.HasMaxLength(5)
.HasColumnName("CustomerID")
.IsFixedLength(true);
entity.Property(e => e.CustomerName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.ExtendedPrice).HasColumnType("money");
entity.Property(e => e.Freight).HasColumnType("money");
entity.Property(e => e.OrderDate).HasColumnType("datetime");
entity.Property(e => e.OrderId).HasColumnName("OrderID");
entity.Property(e => e.PostalCode).HasMaxLength(10);
entity.Property(e => e.ProductId).HasColumnName("ProductID");
entity.Property(e => e.ProductName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.Region).HasMaxLength(15);
entity.Property(e => e.RequiredDate).HasColumnType("datetime");
entity.Property(e => e.Salesperson)
.IsRequired()
.HasMaxLength(31);
entity.Property(e => e.ShipAddress).HasMaxLength(60);
entity.Property(e => e.ShipCity).HasMaxLength(15);
entity.Property(e => e.ShipCountry).HasMaxLength(15);
entity.Property(e => e.ShipName).HasMaxLength(40);
entity.Property(e => e.ShipPostalCode).HasMaxLength(10);
entity.Property(e => e.ShipRegion).HasMaxLength(15);
entity.Property(e => e.ShippedDate).HasColumnType("datetime");
entity.Property(e => e.ShipperName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.UnitPrice).HasColumnType("money");
});
// ...
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
using Microsoft.EntityFrameworkCore;
// ...
builder.Services.AddDbContextFactory<NorthwindContext>((sp, options) => {
var env = sp.GetRequiredService<IWebHostEnvironment>();
var dbPath = Path.Combine(env.ContentRootPath, "Northwind-5e44b51f.mdf");
options.UseSqlServer("Server=(localdb)\\MSSQLLocalDB;Integrated Security=true;AttachDbFileName=" + dbPath);
});
Run Demo: Data Grid - Filter Row View Example: How to implement filter operator selector
See Also