IGrid Interface
An interface that defines the DxGrid component’s API members (properties and methods).
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v23.2.dll
NuGet Package:
DevExpress.Blazor
Declaration
public interface IGrid
The following members return IGrid objects:
We recommend that you use the IGrid
interface when you access Grid API members in any of the following cases:
- You use the
@ref
attribute to reference a Grid object. For example, you set or change values of the Grid parameters outside the component’s markup.
- You access a
Grid
object from templates or event handlers.
In all other cases, bind your data to Grid parameters.
The following code snippet changes multiple sort options in the Grid. Note that when you change a Grid parameter that causes the Grid to update or you need to prevent excessive component redraw operations, enclose your code between the BeginUpdate() and EndUpdate() method calls.
@using Microsoft.EntityFrameworkCore
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
@implements IDisposable
<DxGrid Data="@Data" @ref="MyGrid">
<Columns>
<DxGridDataColumn FieldName="Country" SortIndex="0" />
<DxGridDataColumn FieldName="City" SortIndex="1" />
<DxGridDataColumn FieldName="OrderDate" />
<DxGridDataColumn FieldName="UnitPrice" DisplayFormat="c" />
<DxGridDataColumn FieldName="Quantity" />
</Columns>
</DxGrid>
<br />
<DxButton Click="@(() => ChangeSortInfo())">Change Sort Options</DxButton>
@code {
object Data { get; set; }
NorthwindContext Northwind { get; set; }
IGrid MyGrid { get; set; }
protected override void OnInitialized() {
Northwind = NorthwindContextFactory.CreateDbContext();
Data = Northwind.Invoices
.ToList();
}
void ChangeSortInfo() {
MyGrid.BeginUpdate();
MyGrid.ClearSort();
MyGrid.SortBy("OrderDate");
MyGrid.SortBy("UnitPrice", GridColumnSortOrder.Descending);
MyGrid.EndUpdate();
}
public void Dispose() {
Northwind?.Dispose();
}
}
using System;
#nullable disable
namespace Grid.Northwind {
public partial class Invoice {
public string ShipName { get; set; }
public string ShipAddress { get; set; }
public string ShipCity { get; set; }
public string ShipRegion { get; set; }
public string ShipPostalCode { get; set; }
public string ShipCountry { get; set; }
public string CustomerId { get; set; }
public string CustomerName { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string Salesperson { get; set; }
public int OrderId { get; set; }
public DateTime? OrderDate { get; set; }
public DateTime? RequiredDate { get; set; }
public DateTime? ShippedDate { get; set; }
public string ShipperName { get; set; }
public int ProductId { get; set; }
public string ProductName { get; set; }
public decimal UnitPrice { get; set; }
public short Quantity { get; set; }
public float Discount { get; set; }
public decimal? ExtendedPrice { get; set; }
public decimal? Freight { get; set; }
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Invoice> Invoices { get; set; }
// ...
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
// ...
protected override void OnModelCreating(ModelBuilder modelBuilder) {
// ...
modelBuilder.Entity<Invoice>(entity => {
entity.HasNoKey();
entity.ToView("Invoices");
entity.Property(e => e.Address).HasMaxLength(60);
entity.Property(e => e.City).HasMaxLength(15);
entity.Property(e => e.Country).HasMaxLength(15);
entity.Property(e => e.CustomerId)
.HasMaxLength(5)
.HasColumnName("CustomerID")
.IsFixedLength(true);
entity.Property(e => e.CustomerName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.ExtendedPrice).HasColumnType("money");
entity.Property(e => e.Freight).HasColumnType("money");
entity.Property(e => e.OrderDate).HasColumnType("datetime");
entity.Property(e => e.OrderId).HasColumnName("OrderID");
entity.Property(e => e.PostalCode).HasMaxLength(10);
entity.Property(e => e.ProductId).HasColumnName("ProductID");
entity.Property(e => e.ProductName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.Region).HasMaxLength(15);
entity.Property(e => e.RequiredDate).HasColumnType("datetime");
entity.Property(e => e.Salesperson)
.IsRequired()
.HasMaxLength(31);
entity.Property(e => e.ShipAddress).HasMaxLength(60);
entity.Property(e => e.ShipCity).HasMaxLength(15);
entity.Property(e => e.ShipCountry).HasMaxLength(15);
entity.Property(e => e.ShipName).HasMaxLength(40);
entity.Property(e => e.ShipPostalCode).HasMaxLength(10);
entity.Property(e => e.ShipRegion).HasMaxLength(15);
entity.Property(e => e.ShippedDate).HasColumnType("datetime");
entity.Property(e => e.ShipperName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.UnitPrice).HasColumnType("money");
});
// ...
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
using Microsoft.EntityFrameworkCore;
// ...
builder.Services.AddDbContextFactory<NorthwindContext>((sp, options) => {
var env = sp.GetRequiredService<IWebHostEnvironment>();
var dbPath = Path.Combine(env.ContentRootPath, "Northwind-5e44b51f.mdf");
options.UseSqlServer("Server=(localdb)\\MSSQLLocalDB;Integrated Security=true;AttachDbFileName=" + dbPath);
});
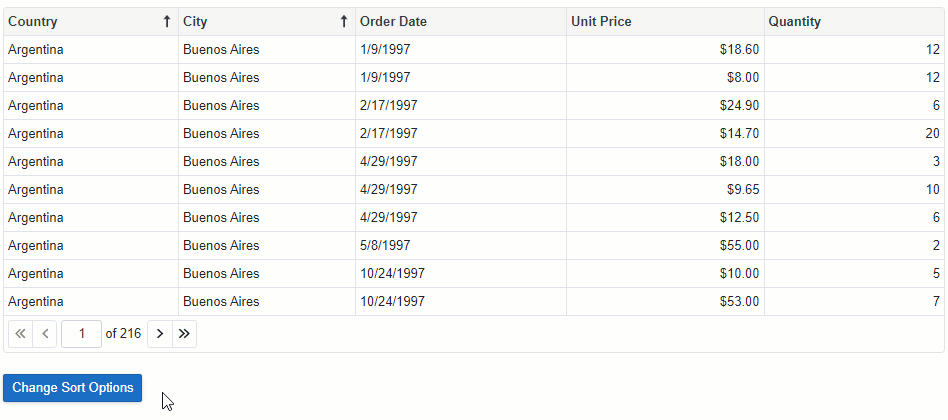
See Also