PivotGridControl.PopupMenuShowing Event
Allows you to customize the context menus.
Namespace: DevExpress.Xpf.PivotGrid
Assembly:
DevExpress.Xpf.PivotGrid.v24.2.dll
NuGet Package:
DevExpress.Wpf.PivotGrid
Declaration
Event Data
The PopupMenuShowing event's data class is PopupMenuShowingEventArgs.
The following properties provide information specific to this event:
Property |
Description |
Customizations |
Allows you to customize the context menu by adding new menu items or removing existing items.
|
Handled |
Gets or sets a value that indicates the present state of the event handling for a routed event as it travels the route.
|
Items |
Gets a collection of items contained within the context menu.
|
MenuType |
Gets what kind of pivot grid element invokes the menu.
|
OriginalSource |
Gets the original reporting source as determined by pure hit testing, before any possible Source adjustment by a parent class.
|
RoutedEvent |
Gets or sets the RoutedEvent associated with this RoutedEventArgs instance.
|
Source |
Gets the PivotGridControl that raised the event.
|
TargetElement |
Gets the UI element for which the context menu is shown.
|
The event data class exposes the following methods:
Method |
Description |
GetCellInfo() |
Returns information about the cell for which the menu is invoked.
|
GetFieldInfo() |
Returns information about the field for whose header the menu is invoked.
|
GetFieldValueInfo() |
Returns information about the field value for which the menu is invoked.
|
InvokeEventHandler(Delegate, Object) |
When overridden in a derived class, provides a way to invoke event handlers in a type-specific way, which can increase efficiency over the base implementation.
|
OnSetSource(Object) |
When overridden in a derived class, provides a notification callback entry point whenever the value of the Source property of an instance changes.
|
The PopupMenuShowing event occurs when an end-user invokes a context menu.
Use the event parameter’s PopupMenuShowingEventArgs.Customizations property to add new and remove existing menu items. This property provides access to a collection of actions that allow you to customize the context menu. To learn more, see Context Menus, Items and Links and Bar Actions.
To determine for what kind of pivot grid element (a cell, a field value, a field header or the field header area) the menu is invoked, use the PopupMenuShowingEventArgs.MenuType property. To gwt the actual visual element for which the menu is invoked, use the PopupMenuShowingEventArgs.TargetElement property.
Use the following methods to get more information about the visual element.
Example
This example demonstrates how to use the PivotGridControl.PopupMenuShowing
event to add custom items to the built-in context menu.
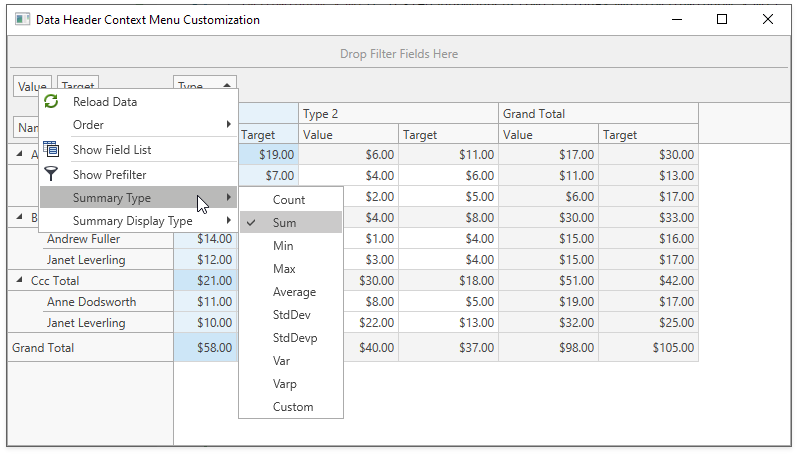
The event is handled automatically if the field’s AllowFieldSummaryTypeChanging or AllowFieldSummaryDisplayTypeChanging attached properties are true. The properties are defined in the HeaderMenuHelper class.
<Window x:Class="HeaderMenuCustomizationExample.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:dxpg="http://schemas.devexpress.com/winfx/2008/xaml/pivotgrid"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:HeaderMenuCustomizationExample"
Title="Data Header Context Menu Customization" Height="450" Width="800"
Loaded="Window_Loaded">
<Grid>
<dxpg:PivotGridControl Name="pivotGridControl1"
local:HeaderMenuHelper.AllowPopupMenuCustomization="True"
RowTreeWidth="130">
<dxpg:PivotGridControl.Fields>
<dxpg:PivotGridField Area="RowArea" FieldName="Name" />
<dxpg:PivotGridField Area="RowArea" FieldName="Owner" />
<dxpg:PivotGridField Area="ColumnArea" FieldName="Type" />
<dxpg:PivotGridField Area="DataArea" FieldName="Value" Name="fieldValue"
local:HeaderMenuHelper.AllowFieldSummaryTypeChanging="True"
local:HeaderMenuHelper.AllowFieldSummaryDisplayTypeChanging="True" />
<dxpg:PivotGridField Area="DataArea" FieldName="Target" Name="fieldTarget"
local:HeaderMenuHelper.AllowFieldSummaryTypeChanging="True"
local:HeaderMenuHelper.AllowFieldSummaryDisplayTypeChanging="True" />
</dxpg:PivotGridControl.Fields>
</dxpg:PivotGridControl>
</Grid>
</Window>
using DevExpress.Xpf.Bars;
using DevExpress.Xpf.PivotGrid;
using System;
using System.Reflection;
using System.Windows;
namespace HeaderMenuCustomizationExample
{
class HeaderMenuHelper
{
#region AttachedProperties
public static readonly DependencyProperty AllowFieldSummaryTypeChangingProperty =
DependencyProperty.RegisterAttached("AllowFieldSummaryTypeChanging", typeof(Boolean), typeof(HeaderMenuHelper));
public static void SetAllowFieldSummaryTypeChanging(DependencyObject element, Boolean value)
{
element.SetValue(AllowFieldSummaryTypeChangingProperty, value);
}
public static Boolean GetAllowFieldSummaryTypeChanging(DependencyObject element)
{
return (Boolean)element.GetValue(AllowFieldSummaryTypeChangingProperty);
}
public static readonly DependencyProperty AllowFieldSummaryDisplayTypeChangingProperty =
DependencyProperty.RegisterAttached("AllowFieldSummaryDisplayTypeChanging", typeof(Boolean), typeof(HeaderMenuHelper));
public static void SetAllowFieldSummaryDisplayTypeChanging(DependencyObject element, Boolean value)
{
element.SetValue(AllowFieldSummaryDisplayTypeChangingProperty, value);
}
public static Boolean GetAllowFieldSummaryDisplayTypeChanging(DependencyObject element)
{
return (Boolean)element.GetValue(AllowFieldSummaryDisplayTypeChangingProperty);
}
public static readonly DependencyProperty AllowPopupMenuCustomizationProperty =
DependencyProperty.RegisterAttached("AllowPopupMenuCustomization", typeof(Boolean), typeof(HeaderMenuHelper),
new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnAllowPopupMenuCustomization)));
public static void SetAllowPopupMenuCustomization(DependencyObject element, Boolean value)
{
element.SetValue(AllowPopupMenuCustomizationProperty, value);
}
public static Boolean GetAllowPopupMenuCustomization(DependencyObject element)
{
return (Boolean)element.GetValue(AllowPopupMenuCustomizationProperty);
}
#endregion AttachedProperties
#region PivotPopupMenuCustomization
public static void OnAllowPopupMenuCustomization(DependencyObject o, DependencyPropertyChangedEventArgs args)
{
PivotGridControl pivotGrid = o as PivotGridControl;
if (pivotGrid == null) return;
if( (Boolean)args.NewValue == true && (Boolean)args.OldValue == false )
pivotGrid.PopupMenuShowing += pivotGrid_PopupMenuShowing;
else if ( (Boolean)args.NewValue == false && (Boolean)args.OldValue == true )
pivotGrid.PopupMenuShowing -= pivotGrid_PopupMenuShowing;
}
static void pivotGrid_PopupMenuShowing(object sender, PopupMenuShowingEventArgs e)
{
if (e.MenuType == PivotGridMenuType.Header)
{
PivotGridControl pivot = (PivotGridControl)sender;
PivotGridField field = e.GetFieldInfo().Field;
if (Convert.ToBoolean(field.GetValue(HeaderMenuHelper.AllowFieldSummaryTypeChangingProperty)))
e.Customizations.Add(CreateBarSubItem("Summary Type", "SummaryType", field));
if (Convert.ToBoolean(field.GetValue(HeaderMenuHelper.AllowFieldSummaryDisplayTypeChangingProperty)))
e.Customizations.Add(CreateBarSubItem("Summary Display Type", "SummaryDisplayType", field));
}
}
#endregion
#region CustomBarItemsCreation
private static BarSubItem CreateBarSubItem(string displayText, string propertyName, PivotGridField field)
{
BarSubItem barSubItem = new BarSubItem();
barSubItem.Name = "bsi" + propertyName;
barSubItem.Content = displayText;
PropertyInfo property = typeof(PivotGridField).GetProperty(propertyName);
foreach (object enumValue in Enum.GetValues(property.PropertyType))
{
BarCheckItem checkItem = new BarCheckItem();
checkItem.Name = "bci" + propertyName + enumValue;
checkItem.Content = enumValue.ToString();
checkItem.IsChecked = Object.Equals(property.GetValue(field, new object[0]), enumValue);
checkItem.Tag = new object[] { field, property, enumValue };
checkItem.ItemClick += itemClickEventHandler;
barSubItem.ItemLinks.Add(checkItem);
}
return barSubItem;
}
static void itemClickEventHandler(object sender, ItemClickEventArgs e)
{
BarItem barItem = sender as BarItem;
object[] barItemInfo = (object[])barItem.Tag;
PivotGridField field = (PivotGridField)barItemInfo[0];
PropertyInfo property = (PropertyInfo)barItemInfo[1];
object newValue = barItemInfo[2];
property.SetValue(field, newValue, new object[0]);
}
#endregion CommonMethods
}
}
Imports DevExpress.Xpf.Bars
Imports DevExpress.Xpf.PivotGrid
Imports System
Imports System.Reflection
Imports System.Windows
Namespace HeaderMenuCustomizationExample
Friend Class HeaderMenuHelper
#Region "AttachedProperties"
Public Shared ReadOnly AllowFieldSummaryTypeChangingProperty As DependencyProperty = DependencyProperty.RegisterAttached("AllowFieldSummaryTypeChanging", GetType(Boolean), GetType(HeaderMenuHelper))
Public Shared Sub SetAllowFieldSummaryTypeChanging(ByVal element As DependencyObject, ByVal value As Boolean)
element.SetValue(AllowFieldSummaryTypeChangingProperty, value)
End Sub
Public Shared Function GetAllowFieldSummaryTypeChanging(ByVal element As DependencyObject) As Boolean
Return DirectCast(element.GetValue(AllowFieldSummaryTypeChangingProperty), Boolean)
End Function
Public Shared ReadOnly AllowFieldSummaryDisplayTypeChangingProperty As DependencyProperty = DependencyProperty.RegisterAttached("AllowFieldSummaryDisplayTypeChanging", GetType(Boolean), GetType(HeaderMenuHelper))
Public Shared Sub SetAllowFieldSummaryDisplayTypeChanging(ByVal element As DependencyObject, ByVal value As Boolean)
element.SetValue(AllowFieldSummaryDisplayTypeChangingProperty, value)
End Sub
Public Shared Function GetAllowFieldSummaryDisplayTypeChanging(ByVal element As DependencyObject) As Boolean
Return DirectCast(element.GetValue(AllowFieldSummaryDisplayTypeChangingProperty), Boolean)
End Function
Public Shared ReadOnly AllowPopupMenuCustomizationProperty As DependencyProperty = DependencyProperty.RegisterAttached("AllowPopupMenuCustomization", GetType(Boolean), GetType(HeaderMenuHelper), New FrameworkPropertyMetadata(False, New PropertyChangedCallback(AddressOf OnAllowPopupMenuCustomization)))
Public Shared Sub SetAllowPopupMenuCustomization(ByVal element As DependencyObject, ByVal value As Boolean)
element.SetValue(AllowPopupMenuCustomizationProperty, value)
End Sub
Public Shared Function GetAllowPopupMenuCustomization(ByVal element As DependencyObject) As Boolean
Return DirectCast(element.GetValue(AllowPopupMenuCustomizationProperty), Boolean)
End Function
#End Region ' AttachedProperties
#Region "PivotPopupMenuCustomization"
Public Shared Sub OnAllowPopupMenuCustomization(ByVal o As DependencyObject, ByVal args As DependencyPropertyChangedEventArgs)
Dim pivotGrid As PivotGridControl = TryCast(o, PivotGridControl)
If pivotGrid Is Nothing Then
Return
End If
If DirectCast(args.NewValue, Boolean) = True AndAlso DirectCast(args.OldValue, Boolean) = False Then
AddHandler pivotGrid.PopupMenuShowing, AddressOf pivotGrid_PopupMenuShowing
ElseIf DirectCast(args.NewValue, Boolean) = False AndAlso DirectCast(args.OldValue, Boolean) = True Then
RemoveHandler pivotGrid.PopupMenuShowing, AddressOf pivotGrid_PopupMenuShowing
End If
End Sub
Private Shared Sub pivotGrid_PopupMenuShowing(ByVal sender As Object, ByVal e As PopupMenuShowingEventArgs)
If e.MenuType = PivotGridMenuType.Header Then
Dim pivot As PivotGridControl = DirectCast(sender, PivotGridControl)
Dim field As PivotGridField = e.GetFieldInfo().Field
If Convert.ToBoolean(field.GetValue(HeaderMenuHelper.AllowFieldSummaryTypeChangingProperty)) Then
e.Customizations.Add(CreateBarSubItem("Summary Type", "SummaryType", field))
End If
If Convert.ToBoolean(field.GetValue(HeaderMenuHelper.AllowFieldSummaryDisplayTypeChangingProperty)) Then
e.Customizations.Add(CreateBarSubItem("Summary Display Type", "SummaryDisplayType", field))
End If
End If
End Sub
#End Region
#Region "CustomBarItemsCreation"
Private Shared Function CreateBarSubItem(ByVal displayText As String, ByVal propertyName As String, ByVal field As PivotGridField) As BarSubItem
Dim barSubItem As New BarSubItem()
barSubItem.Name = "bsi" & propertyName
barSubItem.Content = displayText
Dim [property] As PropertyInfo = GetType(PivotGridField).GetProperty(propertyName)
For Each enumValue As Object In System.Enum.GetValues([property].PropertyType)
Dim checkItem As New BarCheckItem()
checkItem.Name = "bci" + propertyName.ToString() + enumValue.ToString()
checkItem.Content = enumValue.ToString()
checkItem.IsChecked = Object.Equals([property].GetValue(field, New Object(){}), enumValue)
checkItem.Tag = New Object() { field, [property], enumValue }
AddHandler checkItem.ItemClick, AddressOf itemClickEventHandler
barSubItem.ItemLinks.Add(checkItem)
Next enumValue
Return barSubItem
End Function
Private Shared Sub itemClickEventHandler(ByVal sender As Object, ByVal e As ItemClickEventArgs)
Dim barItem As BarItem = TryCast(sender, BarItem)
Dim barItemInfo() As Object = DirectCast(barItem.Tag, Object())
Dim field As PivotGridField = DirectCast(barItemInfo(0), PivotGridField)
Dim [property] As PropertyInfo = DirectCast(barItemInfo(1), PropertyInfo)
Dim newValue As Object = barItemInfo(2)
[property].SetValue(field, newValue, New Object(){})
End Sub
#End Region ' CommonMethods
End Class
End Namespace
The following code snippets (auto-collected from DevExpress Examples) contain references to the PopupMenuShowing event.
Note
The algorithm used to collect these code examples remains a work in progress. Accordingly, the links and snippets below may produce inaccurate results. If you encounter an issue with code examples below, please use the feedback form on this page to report the issue.
See Also