SunburstFlatDataAdapter.LabelDataMember Property
Gets or sets the data member that is a source of Sunburst sector labels.
Namespace: DevExpress.Xpf.TreeMap
Assembly:
DevExpress.Xpf.TreeMap.v24.2.dll
NuGet Package:
DevExpress.Wpf.TreeMap
Declaration
public string LabelDataMember { get; set; }
Public Property LabelDataMember As String
Property Value
Type |
Description |
String |
The name of a data member that is a source of Sunburst sector labels.
|
Example
This example creates a Sunburst chart based on an XML data file with flat structure. To do this, follow the steps below:
<dxtm:SunburstControl>
<dxtm:SunburstControl.DataAdapter>
<dxtm:SunburstFlatDataAdapter
x:Name="dataAdapter"
LabelDataMember="Symbol"
ValueDataMember="Value"
DataSource="{Binding}"
GroupDataMembers="Block, Family"/>
</dxtm:SunburstControl.DataAdapter>
</dxtm:SunburstControl>
using System;
using System.Collections.Generic;
using System.IO;
using System.Windows;
using System.Xml;
using System.Xml.Linq;
namespace SunburstSample {
public partial class MainWindow : Window {
public MainWindow() {
InitializeComponent();
DataContext = LoadDataFromXML();
}
List<ChemicalElement> LoadDataFromXML() {
XDocument document = XDocument.Load(@"D:\ChemicalElements.xml");
List<ChemicalElement> infos = new List<ChemicalElement>();
if (document != null) {
foreach (XElement element in document.Element("ArrayOfElement").Elements()) {
ChemicalElement chemicalElement = new ChemicalElement();
chemicalElement.Name = element.Element("Name").Value;
chemicalElement.AtomicMass = element.Element("AtomicMass").Value;
chemicalElement.AtomicNumber = element.Element("AtomicNumber").Value;
chemicalElement.Density = element.Element("Density").Value;
chemicalElement.MeltingPoint = element.Element("MeltingPoint").Value;
chemicalElement.BoilingPoint = element.Element("BoilingPoint").Value;
chemicalElement.Block = element.Element("Block").Value;
chemicalElement.Family = element.Element("Family").Value;
chemicalElement.Symbol = element.Element("Symbol").Value;
infos.Add(chemicalElement);
}
}
return infos;
}
}
public static class DataLoader {
static Stream GetStream(string fileName) {
Uri uri = GetResourceUri(fileName);
return Application.GetResourceStream(uri).Stream;
}
public static Uri GetResourceUri(string fileName) {
return new Uri(fileName, UriKind.RelativeOrAbsolute);
}
public static XDocument LoadXDocumentFromResources(string fileName) {
try {
return XDocument.Load(GetStream(fileName));
}
catch {
return null;
}
}
}
public class ChemicalElement {
public string AtomicNumber { get; set; }
public string AtomicMass { get; set; }
public string Density { get; set; }
public string MeltingPoint { get; set; }
public string BoilingPoint { get; set; }
public string Name { get; set; }
public string Block { get; set; }
public string Family { get; set; }
public string Symbol { get; set; }
public int Value { get { return 1; } }
}
}
Imports System
Imports System.Collections.Generic
Imports System.IO
Imports System.Xml.Linq
Namespace SunburstSample
Public Partial Class MainWindow
Inherits Window
Public Sub New()
InitializeComponent()
DataContext = LoadDataFromXML()
End Sub
Private Function LoadDataFromXML() As List(Of ChemicalElement)
Dim document As XDocument = XDocument.Load("D:\ChemicalElements.xml")
Dim infos As List(Of ChemicalElement) = New List(Of ChemicalElement)()
If document IsNot Nothing Then
For Each element As XElement In document.Element("ArrayOfElement").Elements()
Dim chemicalElement As ChemicalElement = New ChemicalElement()
chemicalElement.Name = element.Element("Name").Value
chemicalElement.AtomicMass = element.Element("AtomicMass").Value
chemicalElement.AtomicNumber = element.Element("AtomicNumber").Value
chemicalElement.Density = element.Element("Density").Value
chemicalElement.MeltingPoint = element.Element("MeltingPoint").Value
chemicalElement.BoilingPoint = element.Element("BoilingPoint").Value
chemicalElement.Block = element.Element("Block").Value
chemicalElement.Family = element.Element("Family").Value
chemicalElement.Symbol = element.Element("Symbol").Value
infos.Add(chemicalElement)
Next
End If
Return infos
End Function
End Class
Public Module DataLoader
Private Function GetStream(ByVal fileName As String) As Stream
Dim uri As Uri = GetResourceUri(fileName)
Return Application.GetResourceStream(uri).Stream
End Function
Public Function GetResourceUri(ByVal fileName As String) As Uri
Return New Uri(fileName, UriKind.RelativeOrAbsolute)
End Function
Public Function LoadXDocumentFromResources(ByVal fileName As String) As XDocument
Try
Return XDocument.Load(GetStream(fileName))
Catch
Return Nothing
End Try
End Function
End Module
Public Class ChemicalElement
Public Property AtomicNumber As String
Public Property AtomicMass As String
Public Property Density As String
Public Property MeltingPoint As String
Public Property BoilingPoint As String
Public Property Name As String
Public Property Block As String
Public Property Family As String
Public Property Symbol As String
Public ReadOnly Property Value As Integer
Get
Return 1
End Get
End Property
End Class
End Namespace
The data structure looks as follows:
<?xml version="1.0"?>
<ArrayOfElement xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<Element>
<FakeValue>1</FakeValue>
<AtomicNumber>1</AtomicNumber>
<Group>1</Group>
<Period>1</Period>
<Symbol>H</Symbol>
<Name>Hydrogen</Name>
<AtomicMass>1.008</AtomicMass>
<Density>0.00008988</Density>
<MeltingPoint>14.01</MeltingPoint>
<BoilingPoint>20.28</BoilingPoint>
<Family>Nonmetal</Family>
<DiscoveryPeriod>Middle Ages–1799</DiscoveryPeriod>
<Block>S-block</Block>
</Element>
<!--...-->
</ArrayOfElement>
The following image shows the results:
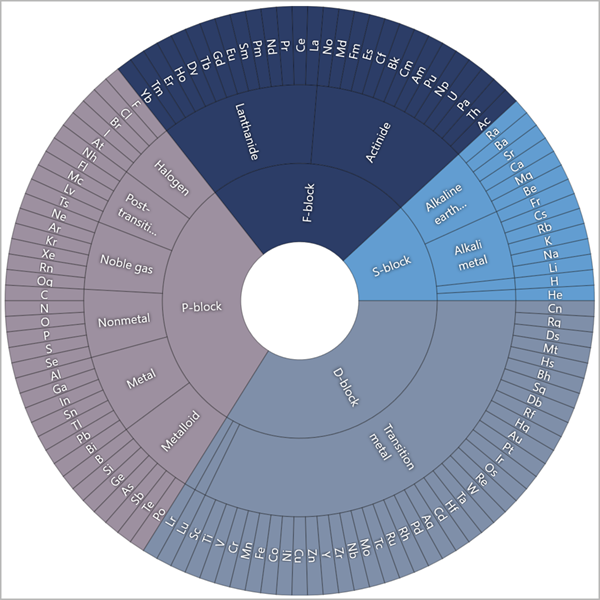
See Also