SunburstHierarchicalDataAdapter Class
Namespace: DevExpress.Xpf.TreeMap
Assembly:
DevExpress.Xpf.TreeMap.v24.1.dll
NuGet Package:
DevExpress.Wpf.TreeMap
Declaration
public class SunburstHierarchicalDataAdapter :
SunburstDataAdapterBase
Public Class SunburstHierarchicalDataAdapter
Inherits SunburstDataAdapterBase
Follow the steps below to load data from hierarchical data sources to a Sunburst chart:
<dxtm:SunburstControl>
<dxtm:SunburstControl.DataAdapter>
<dxtm:SunburstHierarchicalDataAdapter
x:Name="dataAdapter"
DataSource="{Binding}">
<dxtm:SunburstHierarchicalDataAdapter.Mappings>
<dxtm:SunburstDataMapping
LabelDataMember="CountryName"
ChildrenDataMember="CityInfos"
Type="{x:Type local:CountryInfo}"/>
<dxtm:SunburstDataMapping
LabelDataMember="CityName"
ChildrenDataMember="SaleInfos"
Type="{x:Type local:CityInfo}"/>
<dxtm:SunburstDataMapping
ValueDataMember="Total"
LabelDataMember="Category"
Type="{x:Type local:ProductInfo}"/>
</dxtm:SunburstHierarchicalDataAdapter.Mappings>
</dxtm:SunburstHierarchicalDataAdapter>
</dxtm:SunburstControl.DataAdapter>
</dxtm:SunburstControl>
using System.Collections.Generic;
using System.Windows;
namespace HierarchicalSunburst {
public partial class MainWindow : Window {
public MainWindow() {
InitializeComponent();
DataContext = CreateInfos();
}
List<CountryInfo> CreateInfos() {
List<CountryInfo> infos = new List<CountryInfo>();
CountryInfo germanyInfo = new CountryInfo { CountryName = "Germany" };
CityInfo leipzigInfo = new CityInfo { CityName = "Leipzig" };
leipzigInfo.SaleInfos.AddRange(new List<ProductInfo> {
new ProductInfo { Category = "Beverages", Total = 2634.5 },
new ProductInfo { Category = "Baked Goods", Total = 4523.98 },
new ProductInfo { Category = "Grains and Cereals", Total = 913.85 },
new ProductInfo { Category = "Milk and Dairy", Total = 4219.98 },
});
CityInfo berlinInfo = new CityInfo { CityName = "Berlin" };
berlinInfo.SaleInfos.AddRange(new List<ProductInfo> {
new ProductInfo { Category = "Frozen Foods", Total = 900 }
});
germanyInfo.CityInfos.AddRange(new List<CityInfo> { leipzigInfo, berlinInfo });
CountryInfo spainInfo = new CountryInfo { CountryName = "Spain" };
CityInfo barcelonaInfo = new CityInfo { CityName = "Barcelona" };
barcelonaInfo.SaleInfos.AddRange(new List<ProductInfo> {
new ProductInfo { Category = "Baked Goods", Total = 1239.2 },
new ProductInfo { Category = "Fruits", Total = 450.41 },
new ProductInfo { Category = "Milk and Dairy", Total = 692.5 },
});
CityInfo madridInfo = new CityInfo { CityName = "Madrid" };
madridInfo.SaleInfos.AddRange(new List<ProductInfo> {
new ProductInfo { Category = "Spices", Total = 1010.30 },
new ProductInfo { Category = "Vegetables", Total = 2078 }
});
spainInfo.CityInfos.AddRange(new List<CityInfo> { barcelonaInfo, madridInfo });
infos.AddRange(new List<CountryInfo> { germanyInfo, spainInfo });
return infos;
}
}
public class CountryInfo {
public string CountryName { get; set; }
public List<CityInfo> CityInfos { get; set; } = new List<CityInfo>();
}
public class CityInfo {
public string CityName { get; set; }
public List<ProductInfo> SaleInfos { get; set; } = new List<ProductInfo>();
}
public class ProductInfo {
public string Category { get; set; }
public double Total { get; set; }
}
}
Imports System.Collections.Generic
Namespace HierarchicalSunburst
Public Partial Class MainWindow
Inherits Window
Public Sub New()
InitializeComponent()
DataContext = CreateInfos()
End Sub
Private Function CreateInfos() As List(Of CountryInfo)
Dim infos As List(Of CountryInfo) = New List(Of CountryInfo)()
Dim germanyInfo As CountryInfo = New CountryInfo With {
.CountryName = "Germany"
}
Dim leipzigInfo As CityInfo = New CityInfo With {
.CityName = "Leipzig"
}
leipzigInfo.SaleInfos.AddRange(New List(Of ProductInfo) From {
New ProductInfo With {
.Category = "Beverages",
.Total = 2634.5
},
New ProductInfo With {
.Category = "Baked Goods",
.Total = 4523.98
},
New ProductInfo With {
.Category = "Grains and Cereals",
.Total = 913.85
},
New ProductInfo With {
.Category = "Milk and Dairy",
.Total = 4219.98
}
})
Dim berlinInfo As CityInfo = New CityInfo With {
.CityName = "Berlin"
}
berlinInfo.SaleInfos.AddRange(New List(Of ProductInfo) From {
New ProductInfo With {
.Category = "Frozen Foods",
.Total = 900
}
})
germanyInfo.CityInfos.AddRange(New List(Of CityInfo) From {
leipzigInfo,
berlinInfo
})
Dim spainInfo As CountryInfo = New CountryInfo With {
.CountryName = "Spain"
}
Dim barcelonaInfo As CityInfo = New CityInfo With {
.CityName = "Barcelona"
}
barcelonaInfo.SaleInfos.AddRange(New List(Of ProductInfo) From {
New ProductInfo With {
.Category = "Baked Goods",
.Total = 1239.2
},
New ProductInfo With {
.Category = "Fruits",
.Total = 450.41
},
New ProductInfo With {
.Category = "Milk and Dairy",
.Total = 692.5
}
})
Dim madridInfo As CityInfo = New CityInfo With {
.CityName = "Madrid"
}
madridInfo.SaleInfos.AddRange(New List(Of ProductInfo) From {
New ProductInfo With {
.Category = "Spices",
.Total = 1010.30
},
New ProductInfo With {
.Category = "Vegetables",
.Total = 2078
}
})
spainInfo.CityInfos.AddRange(New List(Of CityInfo) From {
barcelonaInfo,
madridInfo
})
infos.AddRange(New List(Of CountryInfo) From {
germanyInfo,
spainInfo
})
Return infos
End Function
End Class
Public Class CountryInfo
Public Property CountryName As String
Public Property CityInfos As List(Of CityInfo) = New List(Of CityInfo)()
End Class
Public Class CityInfo
Public Property CityName As String
Public Property SaleInfos As List(Of ProductInfo) = New List(Of ProductInfo)()
End Class
Public Class ProductInfo
Public Property Category As String
Public Property Total As Double
End Class
End Namespace
The image below shows the results:
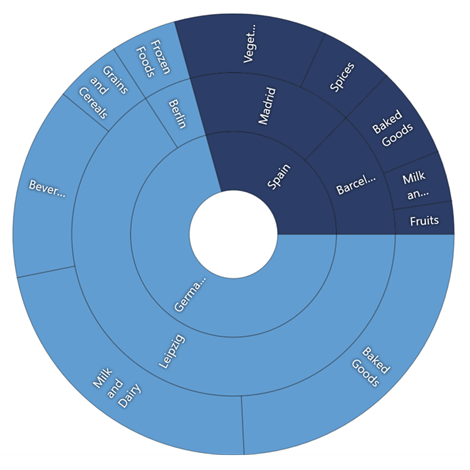
See Also