The following example demonstrates how to specify time scales for the Timeline View using the MVVM architectural pattern.
Use the TimelineView.TimeScalesSource property to bind the view to a collection of objects containing time scale settings described in the ViewModel. The Timeline View generates time scales based on templates. Create multiple templates, one template for each time scale type. In this example, there are three time scale templates: WorkDayScaleTemplate, WorkHourScaleTemplate, and FixedTimeScaleTemplate.
To choose the required template based on the time scale type, create a template selector and assign it to the TimelineView.TimeScaleTemplateSelector property.
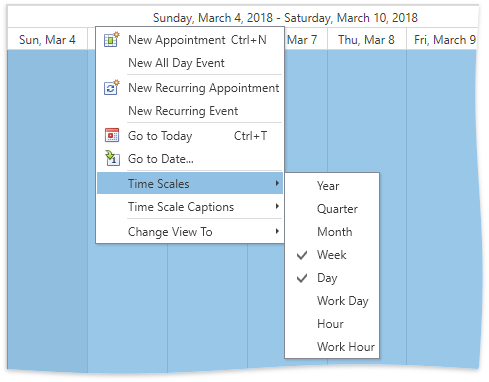
View Example
Imports System.Windows
Imports System.Windows.Controls
Imports System
Namespace WpfSchedulerTimelineScalesTemplate
Public Class TimeScaleTemplateSelector
Inherits DataTemplateSelector
Public Property WorkDayScaleTemplate() As DataTemplate
Public Property WorkHourScaleTemplate() As DataTemplate
Public Property FixedTimeScaleTemplate() As DataTemplate
Public Overrides Function SelectTemplate(ByVal item As Object, ByVal container As DependencyObject) As DataTemplate
Dim timeScale As TimeScaleViewModel = TryCast(item, TimeScaleViewModel)
Select Case timeScale.Type
Case ScaleType.WorkDay
Return WorkDayScaleTemplate
Case ScaleType.WorkHour
Return WorkHourScaleTemplate
Case ScaleType.FixedTime
Return FixedTimeScaleTemplate
Case Else
Throw New NotImplementedException()
End Select
End Function
End Class
End Namespace
Imports DevExpress.Mvvm.POCO
Imports System
Imports System.Collections.Generic
Imports System.Collections.ObjectModel
Namespace WpfSchedulerTimelineScalesTemplate
Public Class MainViewModel
Public Overridable Property Start() As Date
Private privateCalendars As IEnumerable(Of TeamCalendar)
Public Overridable Property Calendars() As IEnumerable(Of TeamCalendar)
Get
Return privateCalendars
End Get
Protected Set(ByVal value As IEnumerable(Of TeamCalendar))
privateCalendars = value
End Set
End Property
Private privateAppointments As IEnumerable(Of TeamAppointment)
Public Overridable Property Appointments() As IEnumerable(Of TeamAppointment)
Get
Return privateAppointments
End Get
Protected Set(ByVal value As IEnumerable(Of TeamAppointment))
privateAppointments = value
End Set
End Property
Private privateTimeScales As ObservableCollection(Of TimeScaleViewModel)
Public Overridable Property TimeScales() As ObservableCollection(Of TimeScaleViewModel)
Get
Return privateTimeScales
End Get
Protected Set(ByVal value As ObservableCollection(Of TimeScaleViewModel))
privateTimeScales = value
End Set
End Property
Protected Sub New()
Start = TeamData.Start
Calendars = New ObservableCollection(Of TeamCalendar)(TeamData.Calendars)
Appointments = New ObservableCollection(Of TeamAppointment)(TeamData.AllAppointments)
CreateTimeScales()
End Sub
Private Sub CreateTimeScales()
TimeScales = New ObservableCollection(Of TimeScaleViewModel)()
AddTimeScale("Work Day", True, ScaleType.WorkDay)
AddTimeScale("Work Hour", True, ScaleType.WorkHour)
AddFixedTimeScale("Half Hour", False, New TimeSpan(0, 30, 0))
End Sub
Private Sub AddTimeScale(ByVal caption As String, ByVal showHeaders As Boolean, ByVal type As ScaleType)
Dim timeScale As TimeScaleViewModel = TimeScaleViewModel.Create()
timeScale.Caption = caption
timeScale.ShowHeaders = showHeaders
timeScale.Type = type
timeScale.IsEnabled = True
TimeScales.Add(timeScale)
End Sub
Private Sub AddFixedTimeScale(ByVal caption As String, ByVal showHeaders As Boolean, ByVal scale As TimeSpan)
Dim timeScale As TimeScaleViewModel = TimeScaleViewModel.Create()
timeScale.Caption = caption
timeScale.Scale = scale
timeScale.ShowHeaders = showHeaders
timeScale.Type = ScaleType.FixedTime
timeScale.IsEnabled = True
TimeScales.Add(timeScale)
End Sub
End Class
Public Enum ScaleType
WorkDay
WorkHour
FixedTime
End Enum
Public Class TimeScaleViewModel
Public Shared Function Create() As TimeScaleViewModel
Return ViewModelSource.Create(Function() New TimeScaleViewModel())
End Function
Protected Sub New()
End Sub
Public Overridable Property Caption() As String
Public Overridable Property ShowHeaders() As Boolean
Public Overridable Property IsEnabled() As Boolean
Public Overridable Property Scale() As TimeSpan
Public Property Type() As ScaleType
End Class
End Namespace
<Window
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfSchedulerTimelineScalesTemplate"
xmlns:dx="http://schemas.devexpress.com/winfx/2008/xaml/core"
xmlns:dxmvvm="http://schemas.devexpress.com/winfx/2008/xaml/mvvm"
xmlns:dxsch="http://schemas.devexpress.com/winfx/2008/xaml/scheduling" x:Class="WpfSchedulerTimelineScalesTemplate.MainWindow"
mc:Ignorable="d"
Title="Timeline View" Height="480" Width="640"
DataContext="{dxmvvm:ViewModelSource local:MainViewModel}">
<Window.Resources>
<local:TimeScaleTemplateSelector x:Key="TimeScaleTemplateSelector">
<local:TimeScaleTemplateSelector.WorkDayScaleTemplate>
<DataTemplate>
<ContentControl>
<dxsch:TimeScaleWorkDay Caption="{Binding Caption}" ShowHeaders="{Binding ShowHeaders}" IsEnabled="{Binding IsEnabled}"/>
</ContentControl>
</DataTemplate>
</local:TimeScaleTemplateSelector.WorkDayScaleTemplate>
<local:TimeScaleTemplateSelector.WorkHourScaleTemplate>
<DataTemplate>
<ContentControl>
<dxsch:TimeScaleWorkHour Caption="{Binding Caption}" ShowHeaders="{Binding ShowHeaders}" IsEnabled="{Binding IsEnabled}"/>
</ContentControl>
</DataTemplate>
</local:TimeScaleTemplateSelector.WorkHourScaleTemplate>
<local:TimeScaleTemplateSelector.FixedTimeScaleTemplate>
<DataTemplate>
<ContentControl>
<dxsch:FixedTimeScale Caption="{Binding Caption}" ShowHeaders="{Binding ShowHeaders}" IsEnabled="{Binding IsEnabled}" Scale="{Binding Scale}"/>
</ContentControl>
</DataTemplate>
</local:TimeScaleTemplateSelector.FixedTimeScaleTemplate>
</local:TimeScaleTemplateSelector>
</Window.Resources>
<Grid>
<dxsch:SchedulerControl x:Name="scheduler" GroupType="None" Start="{Binding Start, Mode=TwoWay}" AllowAppointmentConflicts="True">
<dxsch:TimelineView x:Name="timelineView" IntervalCount="8" TimeScalesSource="{Binding TimeScales}" TimeScaleTemplateSelector="{StaticResource TimeScaleTemplateSelector}"/>
<dxsch:SchedulerControl.DataSource>
<dxsch:DataSource AppointmentsSource="{Binding Appointments}" ResourcesSource="{Binding Calendars}">
<dxsch:DataSource.AppointmentMappings>
<dxsch:AppointmentMappings
Id="Id"
AllDay="AllDay"
Type="AppointmentType"
Start="Start"
End="End"
Subject="Subject"
Description="Description"
Location="Location"
ResourceId="CalendarId"
RecurrenceInfo="RecurrenceInfo"
StatusId="Status"
LabelId="Label"/>
</dxsch:DataSource.AppointmentMappings>
<dxsch:DataSource.ResourceMappings>
<dxsch:ResourceMappings Id="Id" Caption="Name"/>
</dxsch:DataSource.ResourceMappings>
</dxsch:DataSource>
</dxsch:SchedulerControl.DataSource>
</dxsch:SchedulerControl>
</Grid>
</Window>
using System.Windows;
using System.Windows.Controls;
using System;
namespace WpfSchedulerTimelineScalesTemplate {
public class TimeScaleTemplateSelector : DataTemplateSelector {
public DataTemplate WorkDayScaleTemplate { get; set; }
public DataTemplate WorkHourScaleTemplate { get; set; }
public DataTemplate FixedTimeScaleTemplate { get; set; }
public override DataTemplate SelectTemplate(object item, DependencyObject container) {
TimeScaleViewModel timeScale = item as TimeScaleViewModel;
switch (timeScale.Type) {
case ScaleType.WorkDay: return WorkDayScaleTemplate;
case ScaleType.WorkHour: return WorkHourScaleTemplate;
case ScaleType.FixedTime: return FixedTimeScaleTemplate;
default: throw new NotImplementedException();
}
}
}
}
using DevExpress.Mvvm.POCO;
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
namespace WpfSchedulerTimelineScalesTemplate {
public class MainViewModel {
public virtual DateTime Start { get; set; }
public virtual IEnumerable<TeamCalendar> Calendars { get; protected set; }
public virtual IEnumerable<TeamAppointment> Appointments { get; protected set; }
public virtual ObservableCollection<TimeScaleViewModel> TimeScales { get; protected set; }
protected MainViewModel() {
Start = TeamData.Start;
Calendars = new ObservableCollection<TeamCalendar>(TeamData.Calendars);
Appointments = new ObservableCollection<TeamAppointment>(TeamData.AllAppointments);
CreateTimeScales();
}
private void CreateTimeScales() {
TimeScales = new ObservableCollection<TimeScaleViewModel>();
AddTimeScale("Work Day", true, ScaleType.WorkDay);
AddTimeScale("Work Hour", true, ScaleType.WorkHour);
AddFixedTimeScale("Half Hour", false, new TimeSpan(0, 30, 0));
}
private void AddTimeScale(string caption, bool showHeaders, ScaleType type) {
TimeScaleViewModel timeScale = TimeScaleViewModel.Create();
timeScale.Caption = caption;
timeScale.ShowHeaders = showHeaders;
timeScale.Type = type;
timeScale.IsEnabled = true;
TimeScales.Add(timeScale);
}
private void AddFixedTimeScale(string caption, bool showHeaders, TimeSpan scale) {
TimeScaleViewModel timeScale = TimeScaleViewModel.Create();
timeScale.Caption = caption;
timeScale.Scale = scale;
timeScale.ShowHeaders = showHeaders;
timeScale.Type = ScaleType.FixedTime;
timeScale.IsEnabled = true;
TimeScales.Add(timeScale);
}
}
public enum ScaleType { WorkDay, WorkHour, FixedTime }
public class TimeScaleViewModel {
public static TimeScaleViewModel Create() {
return ViewModelSource.Create(() => new TimeScaleViewModel());
}
protected TimeScaleViewModel() { }
public virtual string Caption { get; set; }
public virtual bool ShowHeaders { get; set; }
public virtual bool IsEnabled { get; set; }
public virtual TimeSpan Scale { get; set; }
public ScaleType Type { get; set; }
}
}