DxGrid.Data Property
Specifies an object that supplies Grid data.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v21.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[Parameter]
public object Data { get; set; }
Property Value
Type |
Description |
Object |
Supplies Grid data.
|
Use the Data property to bind the Grid to data. To display data within the Grid, declare DxGridDataColumn objects in the Columns template and use each object’s FieldName property to assign data fields.
Bind to Data Synchronously
If your data collection is loaded synchronously, bind the Data property to a model field and initialize this field with data in the OnInitialized lifecycle method.
@inject WeatherForecastService ForecastService
<DxGrid Data="@Data">
<Columns>
<DxGridDataColumn FieldName="Date" DisplayFormat="D" />
<DxGridDataColumn FieldName="TemperatureC" Caption="@("Temp. (\x2103)")" Width="120px" />
<DxGridDataColumn FieldName="TemperatureF" Caption="@("Temp. (\x2109)")" Width="120px" />
<DxGridDataColumn FieldName="Forecast" />
<DxGridDataColumn FieldName="CloudCover" />
</Columns>
</DxGrid>
@code {
object Data { get; set; }
protected override void OnInitialized() {
Data = ForecastService.GetForecast();
}
}
using System;
public class WeatherForecast {
public DateTime Date { get; set; }
public int TemperatureC { get; set; }
public double TemperatureF => Math.Round((TemperatureC * 1.8 + 32), 2);
public string Forecast { get; set; }
public string CloudCover { get; set; }
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading;
using System.Threading.Tasks;
public class WeatherForecastService {
private List<WeatherForecast> Forecast { get; set; }
private static string[] CloudCover = new[] {
"Sunny", "Partly cloudy", "Cloudy", "Storm"
};
Tuple<int, string>[] ConditionsForForecast = new Tuple<int, string>[] {
Tuple.Create( 22 , "Hot"),
Tuple.Create( 13 , "Warm"),
Tuple.Create( 0 , "Cold"),
Tuple.Create( -10 , "Freezing")
};
public WeatherForecastService() {
Forecast = CreateForecast();
}
private List<WeatherForecast> CreateForecast() {
var rng = new Random();
DateTime startDate = DateTime.Now;
return Enumerable.Range(1, 15).Select(index => {
var temperatureC = rng.Next(-10, 30);
return new WeatherForecast {
Date = startDate.AddDays(index),
TemperatureC = temperatureC,
CloudCover = CloudCover[rng.Next(0, 4)],
Forecast = ConditionsForForecast.First(c => c.Item1 <= temperatureC).Item2
};
}).ToList();
}
public IEnumerable<WeatherForecast> GetForecast() {
return Forecast.ToArray();
}
}
public class Startup {
// ...
public void ConfigureServices(IServiceCollection services) {
// ...
services.AddSingleton<WeatherForecastService>();
// ...
}
// ...
}
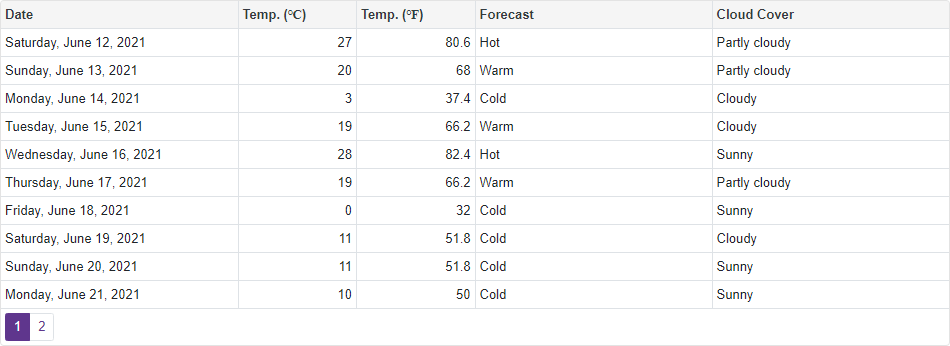
Run Demo: Grid - Data Binding
Bind to Data Asynchronously
If your data collection is loaded asynchronously, bind the Data property to a model field and initialize this field with data in the OnInitializedAsync lifecycle method.
@inject WeatherForecastService ForecastService
<DxGrid Data="@Data">
<Columns>
<DxGridDataColumn FieldName="Date" DisplayFormat="D" />
<DxGridDataColumn FieldName="TemperatureC" Caption="@("Temp. (\x2103)")" Width="120px" />
<DxGridDataColumn FieldName="TemperatureF" Caption="@("Temp. (\x2109)")" Width="120px" />
<DxGridDataColumn FieldName="Forecast" />
<DxGridDataColumn FieldName="CloudCover" />
</Columns>
</DxGrid>
@code {
object Data { get; set; }
protected override async Task OnInitializedAsync() {
Data = await ForecastService.GetForecastAsync();
}
}
using System;
public class WeatherForecast {
public DateTime Date { get; set; }
public int TemperatureC { get; set; }
public double TemperatureF => Math.Round((TemperatureC * 1.8 + 32), 2);
public string Forecast { get; set; }
public string CloudCover { get; set; }
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading;
using System.Threading.Tasks;
public class WeatherForecastService {
private List<WeatherForecast> Forecast { get; set; }
private static string[] CloudCover = new[] {
"Sunny", "Partly cloudy", "Cloudy", "Storm"
};
Tuple<int, string>[] ConditionsForForecast = new Tuple<int, string>[] {
Tuple.Create( 22 , "Hot"),
Tuple.Create( 13 , "Warm"),
Tuple.Create( 0 , "Cold"),
Tuple.Create( -10 , "Freezing")
};
public WeatherForecastService() {
Forecast = CreateForecast();
}
private List<WeatherForecast> CreateForecast() {
var rng = new Random();
DateTime startDate = DateTime.Now;
return Enumerable.Range(1, 15).Select(index => {
var temperatureC = rng.Next(-10, 30);
return new WeatherForecast {
Date = startDate.AddDays(index),
TemperatureC = temperatureC,
CloudCover = CloudCover[rng.Next(0, 4)],
Forecast = ConditionsForForecast.First(c => c.Item1 <= temperatureC).Item2
};
}).ToList();
}
public Task<WeatherForecast[]> GetForecastAsync(CancellationToken ct = default) {
return Task.FromResult(Forecast.ToArray());
}
}
public class Startup {
// ...
public void ConfigureServices(IServiceCollection services) {
// ...
services.AddSingleton<WeatherForecastService>();
// ...
}
// ...
}
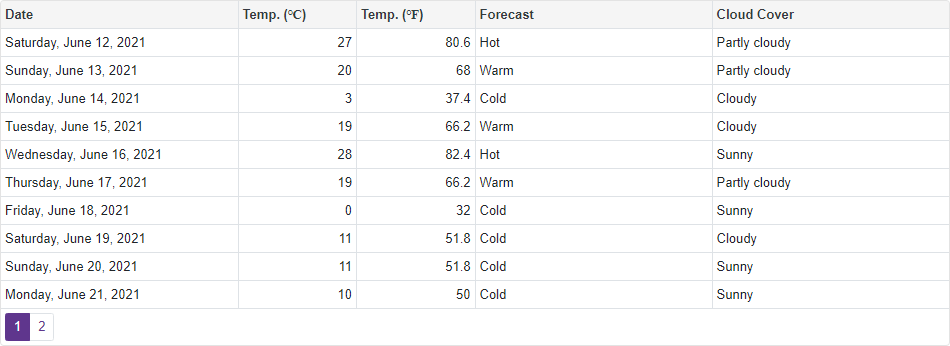
Run Demo: Grid - Asynchronous Data Binding
Bind to Large Data
You can use the Blazor GridDevExtremeDataSource<T> to bind the Grid to a large IQueryable<T> data collection stored locally or published as an HTTP service. Refer to the GridDevExtremeDataSource<T> class description for more information and examples.
Run Demo: Grid - Large Data(Queryable)
Run Demo: Large Data (Queryable as HTTP Service)
Unbound Columns
The Data Grid allows you to add unbound columns whose values are not stored in the assigned data collection. You can calculate column values in two ways:
- Specify the UnboundExpression that calculates an unbound column’s values based on other column values.
- Handle the UnboundColumnData event to supply unique column values based on custom logic.
Fore more information and examples, refer to the following topic: DxGridDataColumn.
See Also