Note
You are viewing documentation for the legacy WPF Scheduler control. If you’re starting a new project, we strongly recommend that you use a new control declared in the DevExpress.Xpf.Scheduling namespace. If you decide to upgrade an existing project in order to switch to the updated scheduler control, see the Migration Guidelines document.
This example demonstrates how to use the AppointmentViewInfo.Subject, AppointmentViewInfo.Location, AppointmentViewInfo.Description, AppointmentViewInfo.CustomViewInfo, SchedulerViewBase.AppointmentToolTipContentTemplate properties and SchedulerControl.AppointmentViewInfoCustomizing event to define and apply a custom template for showing an appointment subject, location, description and resource images within appointment tooltips.
using System.IO;
using System.Data;
using System.Windows;
using System.Data.OleDb;
using DevExpress.Xpf.Core.Native;
using System.Collections.Generic;
using System.Windows.Media.Imaging;
using DevExpress.XtraScheduler;
using DevExpress.Xpf.Scheduler;
using DevExpress.Xpf.Scheduler.Drawing;
namespace WpfApplication1 {
public partial class MainWindow : Window {
Dictionary<Resource, BitmapImage> resourceImages = new Dictionary<Resource, BitmapImage>();
public MainWindow() {
InitializeComponent();
// ...
DataTemplate template = (DataTemplate)this.FindResource("AppointmentTooltipContentTemplate");
schedulerControl1.WorkWeekView.AppointmentToolTipContentTemplate = template;
}
private void schedulerControl1_AppointmentViewInfoCustomizing(object sender,
AppointmentViewInfoCustomizingEventArgs e) {
AppointmentViewInfo viewInfo = e.ViewInfo;
Resource resource = schedulerControl1.Storage.Resources.GetResourceById(viewInfo.Appointment.ResourceId);
if (resource == Resource.Empty || resource.Image == null)
viewInfo.CustomViewInfo = null;
else {
if (!this.resourceImages.ContainsKey(resource))
this.resourceImages[resource] = ImageHelper.CreateImageFromStream(ConvertImageToMemoryStream(resource.Image));
viewInfo.CustomViewInfo = this.resourceImages[resource];
}
}
public static MemoryStream ConvertImageToMemoryStream(System.Drawing.Image img) {
var ms = new MemoryStream();
img.Save(ms, System.Drawing.Imaging.ImageFormat.Png);
return ms;
}
}
}
Imports Microsoft.VisualBasic
Imports System.IO
Imports System.Data
Imports System.Windows
Imports System.Data.OleDb
Imports DevExpress.Xpf.Core.Native
Imports System.Collections.Generic
Imports System.Windows.Media.Imaging
Imports DevExpress.XtraScheduler
Imports DevExpress.Xpf.Scheduler
Imports DevExpress.Xpf.Scheduler.Drawing
Namespace WpfApplication1
Partial Public Class MainWindow
Inherits Window
Private resourceImages As Dictionary(Of Resource, BitmapImage) = New Dictionary(Of Resource, _
BitmapImage)()
Public Sub New()
InitializeComponent()
' ...
Dim template As DataTemplate = CType(Me.FindResource("AppointmentTooltipContentTemplate"), _
DataTemplate)
schedulerControl1.WorkWeekView.AppointmentToolTipContentTemplate = template
End Sub
Private Sub schedulerControl1_AppointmentViewInfoCustomizing(ByVal sender As Object, _
ByVal e As AppointmentViewInfoCustomizingEventArgs)
Dim viewInfo As AppointmentViewInfo = e.ViewInfo
Dim resource As Resource = schedulerControl1.Storage.Resources.GetResourceById(viewInfo.Appointment.ResourceId)
If resource Is resource.Empty OrElse resource.Image Is Nothing Then
viewInfo.CustomViewInfo = Nothing
Else
If (Not Me.resourceImages.ContainsKey(resource)) Then
Me.resourceImages(resource) = ImageHelper.CreateImageFromStream(ConvertImageToMemoryStream(resource.Image))
End If
viewInfo.CustomViewInfo = Me.resourceImages(resource)
End If
End Sub
Public Shared Function ConvertImageToMemoryStream(ByVal img As System.Drawing.Image) As MemoryStream
Dim ms = New MemoryStream()
img.Save(ms, System.Drawing.Imaging.ImageFormat.Png)
Return ms
End Function
End Class
End Namespace
<Window x:Class="WpfApplication1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:dxschcore="clr-namespace:DevExpress.XtraScheduler;assembly=DevExpress.XtraScheduler.v10.2.Core"
xmlns:dxsch="http://schemas.devexpress.com/winfx/2008/xaml/scheduler"
Title="MainWindow" Height="350" Width="525">
<Window.Resources>
<!--AppointmentTooltipTemplate-->
<DataTemplate x:Key="AppointmentTooltipContentTemplate">
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="AUTO"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Border Grid.RowSpan="2" SnapsToDevicePixels="True"
Margin="2" BorderThickness="1"
BorderBrush="DarkGray" CornerRadius="2">
<Image SnapsToDevicePixels="True" Stretch="Uniform"
MaxWidth="140" MaxHeight="100"
Source="{Binding ViewInfo, Path=CustomViewInfo}"/>
</Border>
<TextBlock Grid.Column="1" Text="{Binding ViewInfo, Path=Subject}"/>
<TextBlock Grid.Row="1" Grid.Column="1" Text="{Binding ViewInfo, Path=Location}"/>
<TextBlock Grid.Row="2" Grid.ColumnSpan="2" Text="{Binding ViewInfo, Path=Description}"/>
</Grid>
</DataTemplate>
</Window.Resources>
<Grid>
<dxsch:SchedulerControl
Name="schedulerControl1"
HorizontalAlignment="Stretch" VerticalAlignment="Stretch"
ActiveViewType="WorkWeek"
GroupType="None"
AppointmentViewInfoCustomizing="schedulerControl1_AppointmentViewInfoCustomizing">
<dxsch:SchedulerControl.Storage>
<dxsch:SchedulerStorage>
<!---->
</dxsch:SchedulerStorage>
</dxsch:SchedulerControl.Storage>
</dxsch:SchedulerControl>
</Grid>
</Window>
The image below illustrates a custom tooltip shown for an appointment after applying a template.
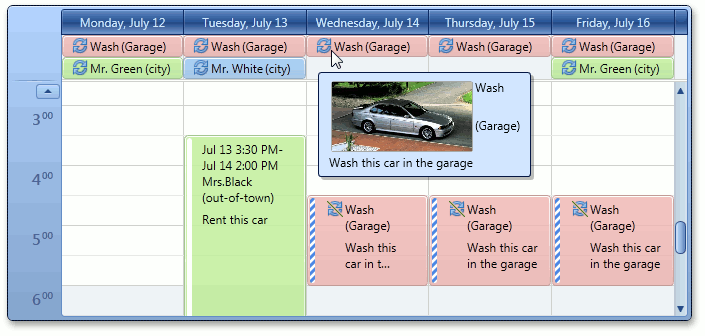