DxListEditorColumn Class
A multi-column editor’s column.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.2.dll
NuGet Package:
DevExpress.Blazor
Declaration
public class DxListEditorColumn :
ParameterTrackerSettingsComponent,
IListEditorColumnComponent,
IListEditorColumn
The following editors can display data across multiple columns:
Follow the steps below to create columns:
- Add
<Columns>...</Columns>
to the component’s markup to define the Columns
collection.
Fill the Columns
collection with DxListEditorColumn
objects. These objects include the following customization options:
- FieldName - Specifies the data source field that populates column items.
- Caption - Specifies the column header.
- Visible - Specifies the column visibility.
- VisibleIndex - Specifies the column display order.
- Width - Specifies the column width.
The following code snippet adds three columns to the List Box component.
@using StaffData
<DxListBox Data="@Staff.DataSource"
@bind-Values="@Values">
<Columns>
<DxListEditorColumn FieldName="Id" Width="50px" />
<DxListEditorColumn FieldName="FirstName" Caption="Name"/>
<DxListEditorColumn FieldName="LastName" Caption="Surname"/>
</Columns>
</DxListBox>
@code {
IEnumerable<Person> Values { get; set; } = Staff.DataSource.Take(1);
}
namespace StaffData {
public static class Staff {
private static readonly Lazy<List<Person>> dataSource = new Lazy<List<Person>>(() => {
var dataSource = new List<Person>() {
new Person() { Id= 0 , FirstName="John", LastName="Heart", Department=Department.Electronics },
new Person() { Id= 1 , FirstName="Samantha", LastName="Bright", Department=Department.Motors },
new Person() { Id= 2 , FirstName="Arthur", LastName="Miller", Department=Department.Software },
new Person() { Id= 3 , FirstName="Robert", LastName="Reagan", Department=Department.Electronics },
new Person() { Id= 4 , FirstName="Greta", LastName="Sims", Department=Department.Motors },
new Person() { Id= 5 , FirstName="Brett", LastName="Wade", Department=Department.Software },
new Person() { Id= 6 , FirstName="Sandra", LastName="Johnson", Department=Department.Electronics },
new Person() { Id= 7 , FirstName="Edward", LastName="Holmes", Department=Department.Motors },
new Person() { Id= 8 , FirstName="Barbara", LastName="Banks", Department=Department.Software },
new Person() { Id= 9 , FirstName="Kevin", LastName="Carter", Department=Department.Electronics },
new Person() { Id= 10, FirstName="Cynthia", LastName="Stanwick", Department=Department.Motors },
new Person() { Id= 11, FirstName="Sam", LastName="Hill", Department=Department.Electronics }};
return dataSource;
});
public static List<Person> DataSource { get { return dataSource.Value; } }
}
public class Person {
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public Department Department { get; set; }
public string Text => $"{FirstName} {LastName} ({Department} Dept.)";
public override bool Equals(object obj) {
if (obj is Person typedObj) {
return (this.Id == typedObj.Id) && (this.FirstName == typedObj.FirstName) && (this.LastName == typedObj.LastName)
&& (this.Department == typedObj.Department);
}
return base.Equals(obj);
}
}
public enum Department { Motors, Electronics, Software }
}
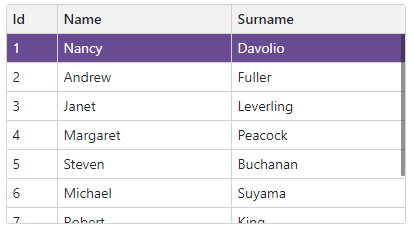
Run Demo: List Box – Multiple Columns
Auto-Generated Multi-Column Editors in Grid
The Grid component generates and configures cell editors for individual columns based on associated data types. The component displays these cell editors in the filter row and edit cells. Refer to the following topics for more information:
You can configure an auto-generated combo box to display multiple columns in the dropdown in the same way as for a standalone editor.
The following code snippet adds three columns to the combo box editor and formats its edit value:
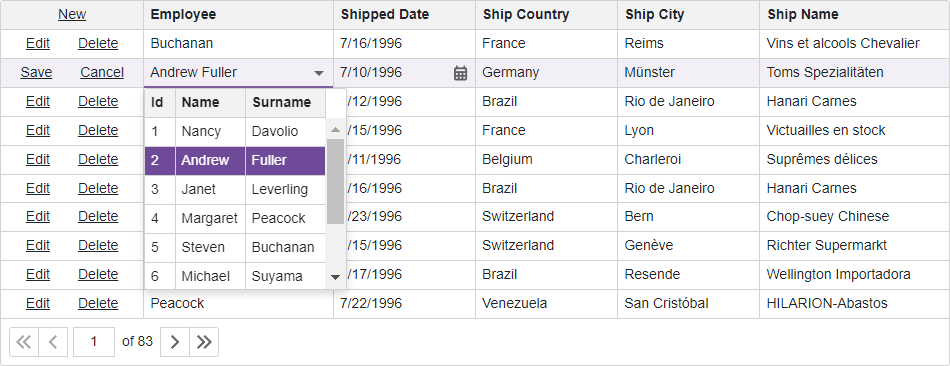
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
<DxGrid Data="Orders"
EditMode="GridEditMode.EditRow">
<Columns>
<DxGridCommandColumn />
<DxGridDataColumn FieldName="EmployeeId" Caption="Employee" Width="20%">
<EditSettings>
<DxComboBoxSettings Data="Employees"
TextFieldName="LastName"
ValueFieldName="EmployeeId"
EditFormat="{1} {2}" >
<Columns>
<DxListEditorColumn FieldName="EmployeeId" Caption="Id" Width="30px" />
<DxListEditorColumn FieldName="FirstName" Caption="Name" />
<DxListEditorColumn FieldName="LastName" Caption="Surname" />
</Columns>
</DxComboBoxSettings>
</EditSettings>
</DxGridDataColumn>
<DxGridDataColumn FieldName="ShippedDate" />
<DxGridDataColumn FieldName="ShipCountry" />
<DxGridDataColumn FieldName="ShipCity" />
<DxGridDataColumn FieldName="ShipName" Width="20%" />
</Columns>
</DxGrid>
@code {
NorthwindContext Northwind { get; set; }
List<Order> Orders { get; set; }
List<Employee> Employees { get; set; }
protected override async Task OnInitializedAsync() {
Northwind = NorthwindContextFactory.CreateDbContext();
Orders = await Northwind.Orders.ToListAsync();
Employees = await Northwind.Employees.ToListAsync();
}
public void Dispose() {
Northwind?.Dispose();
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Order> Orders { get; set; }
public virtual DbSet<Employee> Employees { get; set; }
// ...
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder) {
modelBuilder.HasAnnotation("Relational:Collation", "SQL_Latin1_General_CP1_CI_AS");
// ...
modelBuilder.Entity<Order>(entity => {
entity.HasIndex(e => e.CustomerId, "CustomerID");
entity.HasIndex(e => e.CustomerId, "CustomersOrders");
entity.HasIndex(e => e.EmployeeId, "EmployeeID");
entity.HasIndex(e => e.EmployeeId, "EmployeesOrders");
entity.HasIndex(e => e.OrderDate, "OrderDate");
entity.HasIndex(e => e.ShipPostalCode, "ShipPostalCode");
entity.HasIndex(e => e.ShippedDate, "ShippedDate");
entity.HasIndex(e => e.ShipVia, "ShippersOrders");
entity.Property(e => e.OrderId).HasColumnName("OrderID");
entity.Property(e => e.CustomerId)
.HasMaxLength(5)
.HasColumnName("CustomerID")
.IsFixedLength(true);
entity.Property(e => e.EmployeeId).HasColumnName("EmployeeID");
entity.Property(e => e.Freight)
.HasColumnType("money")
.HasDefaultValueSql("((0))");
entity.Property(e => e.OrderDate).HasColumnType("datetime");
entity.Property(e => e.RequiredDate).HasColumnType("datetime");
entity.Property(e => e.ShipAddress).HasMaxLength(60);
entity.Property(e => e.ShipCity).HasMaxLength(15);
entity.Property(e => e.ShipCountry).HasMaxLength(15);
entity.Property(e => e.ShipName).HasMaxLength(40);
entity.Property(e => e.ShipPostalCode).HasMaxLength(10);
entity.Property(e => e.ShipRegion).HasMaxLength(15);
entity.Property(e => e.ShippedDate).HasColumnType("datetime");
});
modelBuilder.Entity<Employee>(entity => {
entity.HasIndex(e => e.EmployeeId, "EmployeeId");
entity.HasIndex(e => e.LastName, "LastName");
entity.HasIndex(e => e.FirstName, "FirstName");
entity.HasIndex(e => e.Title, "Title");
entity.HasIndex(e => e.BirthDate, "BirthDate");
entity.HasIndex(e => e.HireDate, "HireDate");
entity.HasIndex(e => e.Notes, "Notes");
});
// ...
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
public partial class Order {
public Order() {
OrderDetails = new HashSet<OrderDetail>();
}
public int OrderId { get; set; }
public string CustomerId { get; set; }
public int? EmployeeId { get; set; }
public DateTime? OrderDate { get; set; }
public DateTime? RequiredDate { get; set; }
public DateTime? ShippedDate { get; set; }
public int? ShipVia { get; set; }
public decimal? Freight { get; set; }
public string ShipName { get; set; }
public string ShipAddress { get; set; }
public string ShipCity { get; set; }
public string ShipRegion { get; set; }
public string ShipPostalCode { get; set; }
public string ShipCountry { get; set; }
}
public partial class Employee {
public int EmployeeId { get; set; }
public string LastName { get; set; }
public string FirstName { get; set; }
public string Title { get; set; }
public string TitleOfCourtesy { get; set; }
public Nullable<System.DateTime> BirthDate { get; set; }
public Nullable<System.DateTime> HireDate { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string HomePhone { get; set; }
public string Extension { get; set; }
public byte[] Photo { get; set; }
public string Notes { get; set; }
public Nullable<int> ReportsTo { get; set; }
public string PhotoPath { get; set; }
public virtual ICollection<Order> Orders { get; set; }
public string Text => $"{FirstName} {LastName} ({Title})";
public string ImageFileName => $"Employees/{EmployeeId}.jpg";
}
Inheritance
ObjectComponentBaseDevExpress.Blazor.Internal.BranchedRenderComponentDevExpress.Blazor.Internal.ParameterTrackerSettingsComponentDxListEditorColumn
See Also