The start-up application screen contains a Page container (page1) with a GridControl. Double-clicking a grid row takes a user to page2, which contains a separate Grid with detailed employee information. Both containers’ documents contain user controls (ucGeneral and ucDetails, respectively).
In the Designer’s “Navigation Tree” tab, drag Page2 to the Page1 “Children” line. By doing so you assign Page1 to the Page2’s BaseContentContainer.Parent property and make it possible for end-users to navigate back from the detailed employee information page.
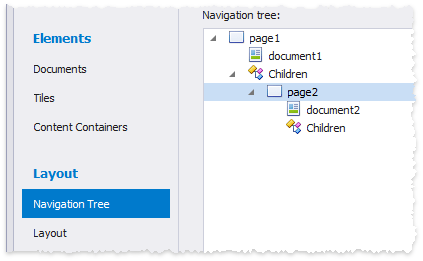
Handle the WindowsUIView.NavigatedTo event, and assign the details Page to the NavigationEventArgs.Parameter property.
private void windowsUIView1_NavigatedTo(object sender, DevExpress.XtraBars.Docking2010.Views.WindowsUI.NavigationEventArgs e) {
if (e.Target == page1) e.Parameter = page2;
}
Private Sub windowsUIView1_NavigatedTo(ByVal sender As Object, ByVal e As DevExpress.XtraBars.Docking2010.Views.WindowsUI.NavigationEventArgs) Handles windowsUIView1.NavigatedTo
If e.Target Is page1 Then
e.Parameter = page2
End If
End Sub
Implement the ISupportNavigation interface and expose its ISupportNavigation.OnNavigatedTo and ISupportNavigation.OnNavigatedFrom methods for both UserControls. At application start-up, the OnNavigatedTo method for the ucGeneral UserControl receives the details page as a parameter from the NavigatedTo event that was handled in the previous step.
//ucGeneral.cs
public partial class ucGeneral : UserControl, ISupportNavigation {
WindowsUIView generalView;
Page detailsPage;
public void OnNavigatedFrom(INavigationArgs args) {
}
public void OnNavigatedTo(INavigationArgs args) {
generalView = args.View;
detailsPage = args.Parameter as Page;
}
}
'ucGeneral.vb
Public Partial Class ucGeneral
Inherits UserControl
Implements ISupportNavigation
Private generalView As WindowsUIView
Private detailsPage As Page
Public Sub OnNavigatedFrom(ByVal args As INavigationArgs) Implements ISupportNavigation.OnNavigatedFrom
End Sub
Public Sub OnNavigatedTo(ByVal args As INavigationArgs) Implements ISupportNavigation.OnNavigatedTo
generalView = args.View
detailsPage = TryCast(args.Parameter, Page)
End Sub
End Class
- Set the ColumnViewOptionsBehavior.Editable property to false, so that you can handle the double-click event. This property is located in the GridView.OptionsBehavior section.
Handle the GridView’s double click event and call the IBaseViewController.Activate method to navigate to the details page.
//ucGeneral.cs
private void gridView1_DoubleClick(object sender, EventArgs e) {
generalView.Controller.Activate(detailsPage);
}
'ucGeneral.vb
Private Sub gridView1_DoubleClick(ByVal sender As Object, ByVal e As EventArgs) Handles gridView1.DoubleClick
generalView.Controller.Activate(detailsPage)
End Sub
Handle the OnNavigatedFrom method for the general page and assign the focused row to the Parameter property. This parameter is passed to the OnNavigatedTo method of the details page, and you are able to retrieve any required data and fill the details page. In this example, a simple filter criteria is applied.
//ucGeneral.cs
public void OnNavigatedFrom(INavigationArgs args) {
args.Parameter = gridView1.GetDataRow(gridView1.FocusedRowHandle);
}
//ucDetails.cs
public void OnNavigatedTo(INavigationArgs args) {
detailsID = (args.Parameter as ParamNavigation.nwindDataSet.EmployeesRow).EmployeeID.ToString();
layoutView1.ActiveFilterString = "[EmployeeID] = " + detailsID;
}
'ucGeneral.vb
Public Sub OnNavigatedFrom(ByVal args As INavigationArgs) Implements ISupportNavigation.OnNavigatedFrom
args.Parameter = gridView1.GetDataRow(gridView1.FocusedRowHandle)
End Sub
'ucDetails.vb
Public Sub OnNavigatedTo(ByVal args As INavigationArgs) Implements ISupportNavigation.OnNavigatedTo
detailsID = (TryCast(args.Parameter, ParamNavigation.nwindDataSet.EmployeesRow)).EmployeeID.ToString()
layoutView1.ActiveFilterString = "[EmployeeID] = " & detailsID
End Sub
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using DevExpress.XtraBars.Docking2010.Views.WindowsUI;
namespace ParamNavigation {
public partial class ucGeneral : UserControl, ISupportNavigation {
WindowsUIView generalView;
Page detailsPage;
public ucGeneral() {
InitializeComponent();
employeesTableAdapter.Fill(nwindDataSet.Employees);
}
public void OnNavigatedFrom(INavigationArgs args) {
args.Parameter = gridView1.GetDataRow(gridView1.FocusedRowHandle);
}
public void OnNavigatedTo(INavigationArgs args) {
generalView = args.View;
detailsPage = args.Parameter as Page;
}
private void gridView1_DoubleClick(object sender, EventArgs e) {
generalView.Controller.Activate(detailsPage);
}
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using DevExpress.XtraBars.Docking2010.Views.WindowsUI;
namespace ParamNavigation {
public partial class ucDetails : UserControl, ISupportNavigation {
string detailsID;
public ucDetails() {
InitializeComponent();
employeesTableAdapter.Fill(nwindDataSet.Employees);
}
public void OnNavigatedFrom(INavigationArgs args) {
}
public void OnNavigatedTo(INavigationArgs args) {
detailsID = (args.Parameter as ParamNavigation.nwindDataSet.EmployeesRow).EmployeeID.ToString();
layoutView1.ActiveFilterString = "[EmployeeID] = " + detailsID;
}
}
}
Imports Microsoft.VisualBasic
Imports System
Imports System.Collections.Generic
Imports System.ComponentModel
Imports System.Drawing
Imports System.Data
Imports System.Linq
Imports System.Text
Imports System.Threading.Tasks
Imports System.Windows.Forms
Imports DevExpress.XtraBars.Docking2010.Views.WindowsUI
Namespace ParamNavigation
Public Partial Class ucGeneral
Inherits UserControl
Implements ISupportNavigation
Private generalView As WindowsUIView
Private detailsPage As Page
Public Sub New()
InitializeComponent()
employeesTableAdapter.Fill(nwindDataSet.Employees)
End Sub
Public Sub OnNavigatedFrom(ByVal args As INavigationArgs) Implements ISupportNavigation.OnNavigatedFrom
args.Parameter = gridView1.GetDataRow(gridView1.FocusedRowHandle)
End Sub
Public Sub OnNavigatedTo(ByVal args As INavigationArgs) Implements ISupportNavigation.OnNavigatedTo
generalView = args.View
detailsPage = TryCast(args.Parameter, Page)
End Sub
Private Sub gridView1_DoubleClick(ByVal sender As Object, ByVal e As EventArgs) Handles gridView1.DoubleClick
generalView.Controller.Activate(detailsPage)
End Sub
End Class
End Namespace
Imports Microsoft.VisualBasic
Imports System
Imports System.Collections.Generic
Imports System.ComponentModel
Imports System.Drawing
Imports System.Data
Imports System.Linq
Imports System.Text
Imports System.Threading.Tasks
Imports System.Windows.Forms
Imports DevExpress.XtraBars.Docking2010.Views.WindowsUI
Namespace ParamNavigation
Public Partial Class ucDetails
Inherits UserControl
Implements ISupportNavigation
Private detailsID As String
Public Sub New()
InitializeComponent()
employeesTableAdapter.Fill(nwindDataSet.Employees)
End Sub
Public Sub OnNavigatedFrom(ByVal args As INavigationArgs) Implements ISupportNavigation.OnNavigatedFrom
End Sub
Public Sub OnNavigatedTo(ByVal args As INavigationArgs) Implements ISupportNavigation.OnNavigatedTo
detailsID = (TryCast(args.Parameter, ParamNavigation.nwindDataSet.EmployeesRow)).EmployeeID.ToString()
layoutView1.ActiveFilterString = "[EmployeeID] = " & detailsID
End Sub
End Class
End Namespace