Assume that you have a PageGroup containing 2 Documents and a Page that can display any of these Documents separately. The objective is to display different Buttons within these Containers depending on the currently activated Document. Documents are generated based on the User Controls included in the project (see the Documents topic for details). To add a Document Action to this Document, implement the ISupportDocumentActions interface for the related User Control, as demonstrated in the code below.
public partial class UserControl1 : UserControl, ISupportDocumentActions {
}
Public Partial Class UserControl1
Inherits UserControl
Implements ISupportDocumentActions
End Class
To implement an interface’s methods, use the corresponding item from the Visual Studio pop-up menu.
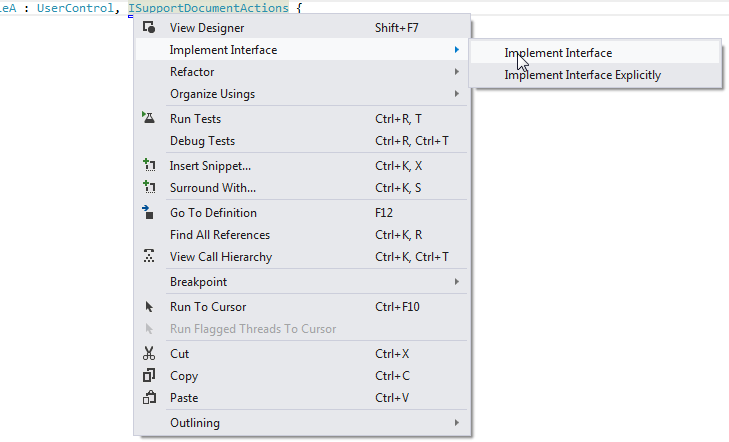
The only ISupportDocumentActions interface method is ISupportDocumentActions.OnQueryDocumentActions. This method is called automatically, each time a related Document is activated. In this method, you can add custom DocumentAction objects to the IDocumentActionsArgs.DocumentActions collection. Add two Document Actions to the first Document.
public void OnQueryDocumentActions(IDocumentActionsArgs args) {
args.DocumentActions.Add(new DocumentAction(DoAction1) { Caption = "Action 1" });
args.DocumentActions.Add(new DocumentAction(CanDoAction2, DoAction2) { Caption = "Action 2" });
}
Public Sub OnQueryDocumentActions(args As IDocumentActionsArgs) Implements ISupportDocumentActions.OnQueryDocumentActions
args.DocumentActions.Add(New DocumentAction(Sub(doc) DoAction1(args.Document)) With { _
.Caption = "Action 1" _
})
args.DocumentActions.Add(New DocumentAction(Function(doc) CanDoAction2(args.Document), Sub(doc) DoAction2(args.Document)) With { _
.Caption = "Action 2" _
})
End Sub
Document Actions act like standard Windows Forms buttons. When creating an Action, you need to specify the execute method that implements the Action’s functionality, and (optionally) the canExecute method, which specifies the set of criteria and checks whether or not the current Document meets these criteria. You can specify these methods as shown in the code below.
void DoAction1(Document document) {
. . .
}
bool CanDoAction2(Document document) {
. . .
}
void DoAction2(Document document) {
. . .
}
Private Sub DoAction1(ByVal document As Document)
. . .
End Sub
Private Function CanDoAction2(ByVal document As Document) As Boolean
. . .
End Function
Private Sub DoAction2(ByVal document As Document)
. . .
End Sub
You now have two Document Actions that act like simple “push” buttons in your first Document. Actions can also act like check buttons that have two states - checked and unchecked. To create these Actions in your second Document, implement the ISupportDocumentActions interface (as you did before) and add two DocumentCheckAction objects to the IDocumentActionsArgs.DocumentActions collection.
public void OnQueryDocumentActions(IDocumentActionsArgs args) {
args.DocumentActions.Add(new DocumentCheckAction(() => flag, OnToggle) { Caption = "Flag" });
args.DocumentActions.Add(new DocumentCheckAction(() => isSpecificState, OnCheck, OnUncheck) { Caption = "Specific State" });
}
Public Sub OnQueryDocumentActions(args As IDocumentActionsArgs) Implements ISupportDocumentActions.OnQueryDocumentActions
args.DocumentActions.Add(New DocumentCheckAction(Function() viewModel.Flag, Sub() OnToggle(args.Document)) With { _
.Caption = "Flag" _
})
args.DocumentActions.Add(New DocumentCheckAction(Function() viewModel.IsSpecificState, Sub() OnCheck(args.Document), Sub() OnUncheck(args.Document)) With { _
.Caption = "Specific State" _
})
End Sub
In the code above, two different overloads are used to initialize DocumentCheckAction objects that act differently. Both Actions receive a Boolean variable as the first getState parameter. These variables store a Boolean value depending on the current Action’s check state.
public bool flag;
public bool isSpecificState;
Public flag As Boolean
Public isSpecificState As Boolean
The first Check Action receives the OnToggle method as the last parameter. This method takes a Document as the parameter and is executed each time the Action’s check state changes.
void OnToggle(Document document) {
//do something
}
Private Sub OnToggle(ByVal document As Document)
'do something
End Sub
The ‘Specific State’ Check Action receives two methods - the OnCheck method is called when the Action is checked, and the OnUncheck method is called when the Action is unchecked. This allows you to perform different actions depending on the Action’s check state. Compare this to the first Check Action, which performs the same actions whenever its check state changes.
void OnCheck(Document document) {
//First actions set
}
void OnUncheck(Document document) {
//Second actions set
}
Private Sub OnCheck(ByVal document As Document)
'First actions set
End Sub
Private Sub OnUncheck(ByVal document As Document)
'Second actions set
End Sub
To display the Actions you have added, hide the default PageGroup header. To do so, set the IPageGroupDefaultProperties.ShowPageHeaders property to DefaultBoolean.False.
pageGroup1.Properties.ShowPageHeaders = DevExpress.Utils.DefaultBoolean.False;
pageGroup1.Properties.ShowPageHeaders = DevExpress.Utils.DefaultBoolean.False
The problem is that from now on, end-users can no longer navigate through PageGroup items because there are no page headers. To resolve this issue, add two Document Actions that will implement the navigation functionality. For these Actions, you will handle the WindowsUIView.QueryDocumentActions event. Both Document Actions are added at once, but since only one Document within a PageGroup can be activated, the canExecute method for only one corresponding Action will return true. Thus, both Actions will not be simultaneously visible.
void windowsUIView1_QueryDocumentActions(object sender, QueryDocumentActionsEventArgs e) {
WindowsUIView view = sender as WindowsUIView;
if (e.Source == pageGroup1) {
e.DocumentActions.Add(new DocumentAction((doc) => doc == document2,
(doc) => view.ActivateDocument(document1)) { Caption = "Document 1" });
e.DocumentActions.Add(new DocumentAction((doc) => doc == document1,
(doc) => view.ActivateDocument(document2)) { Caption = "Document 2" });
}
}
Private Sub windowsUIView1_QueryDocumentActions(sender As Object, e As QueryDocumentActionsEventArgs)
Dim view As WindowsUIView = TryCast(sender, WindowsUIView)
If e.Source = pageGroup1 Then
e.DocumentActions.Add(New DocumentAction(Function(doc) doc = document2, Function(doc) view.ActivateDocument(document1)) With {.Caption = "Document 1"})
e.DocumentActions.Add(New DocumentAction(Function(doc) doc = document1, Function(doc) view.ActivateDocument(document2)) With {.Caption = "Document 2"})
End If
End Sub
Finally, the code below illustrates how to add the ‘Hello’ Document Action. This is an example of an Action that does not have a canExecute method, and thus is displayed within each Content Container.
void windowsUIView1_QueryDocumentActions(object sender, QueryDocumentActionsEventArgs e) {
e.DocumentActions.Add(new DocumentAction(Hello) { Caption = "Hello" });
}
void Hello(Document document) {
MessageBox.Show("Hello from " + document.Caption);
}
Private Sub windowsUIView1_QueryDocumentActions(sender As Object, e As QueryDocumentActionsEventArgs)
e.DocumentActions.Add(New DocumentAction(Sub(doc) Hello(e.Document)) With { _
.Caption = "Hello" _
})
End Sub
Private Sub Hello(ByVal document As Document)
MessageBox.Show("Hello from " & Convert.ToString(document.Caption))
End Sub
The animation below shows the result. The Page container has different Actions depending on the activated Document (DocumentActions for the first Document and DocumentCheckActions for the second Document). The PageGroup container also changes its Actions when navigating through its items, and displays either ‘Document 1’ or ‘Document 2’ navigation Actions. The ‘Hello’ action is displayed within any Content Container regardless of the currently activated Document.
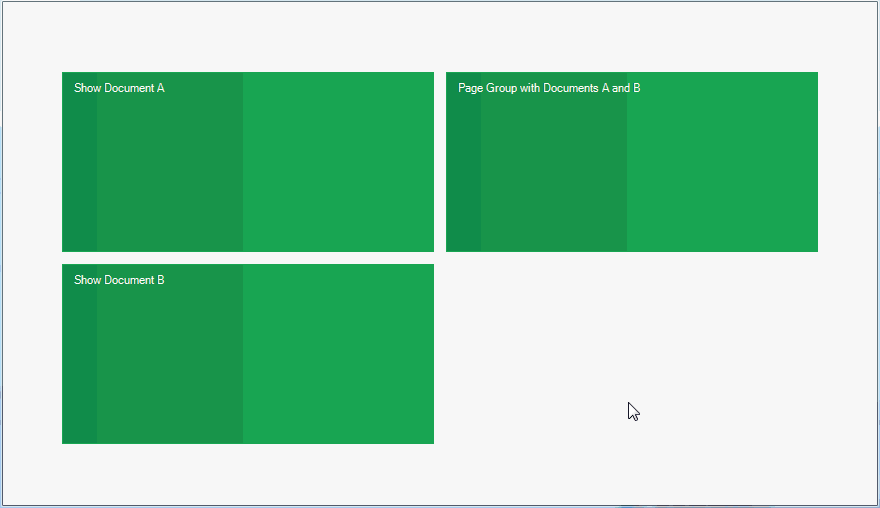
using System.Windows.Forms;
using DevExpress.XtraBars.Docking2010.Views.WindowsUI;
using WindowsFormsApplication1.ViewModels;
namespace WindowsFormsApplication1.Views {
public partial class ucModuleA : UserControl, ISupportDocumentActions {
ViewModelModuleA viewModel;
public ucModuleA(ViewModelModuleA viewModel) {
this.viewModel = viewModel;
InitializeComponent();
}
#region ISupportDocumentActions Members
public void OnQueryDocumentActions(IDocumentActionsArgs args) {
args.DocumentActions.Add(new DocumentAction(DoAction1) { Caption = "Action 1" });
args.DocumentActions.Add(new DocumentAction(CanDoAction2, DoAction2) { Caption = "Action 2" });
}
#endregion
void DoAction1(Document document) {
bool prev = viewModel.AllowAnotherAction;
viewModel.DoSomeAction();
MessageBox.Show("Some Action");
if(!prev && prev != viewModel.AllowAnotherAction)
MessageBox.Show("Action 2 enabled");
}
bool CanDoAction2(Document document) {
return viewModel.AllowAnotherAction;
}
void DoAction2(Document document) {
MessageBox.Show("Action 2 disabled");
viewModel.ResetSomeAction();
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace WindowsFormsApplication1.ViewModels {
public class ViewModelModuleB {
public bool Flag { get; set; }
public bool IsSpecificState { get; set; }
public void SetSpecicState() {
IsSpecificState = true;
}
public void ResetSpecificState() {
IsSpecificState = false;
}
}
}
using System.Windows.Forms;
using DevExpress.XtraBars.Docking2010.Views.WindowsUI;
using DevExpress.XtraBars.Docking2010.Views.WindowsUI;
using WindowsFormsApplication1.ViewModels;
namespace WindowsFormsApplication1.Views {
public partial class ucModuleB : UserControl, ISupportDocumentActions {
ViewModelModuleB viewModel;
public ucModuleB(ViewModelModuleB viewModel) {
this.viewModel = viewModel;
InitializeComponent();
}
#region ISupportDocumentActions Members
public void OnQueryDocumentActions(IDocumentActionsArgs args) {
args.DocumentActions.Add(new DocumentCheckAction(() => viewModel.Flag, OnToggle) { Caption = "Flag" });
args.DocumentActions.Add(new DocumentCheckAction(() => viewModel.IsSpecificState, OnCheck, OnUncheck) { Caption = "Specific State" });
}
#endregion
void OnToggle(Document document) {
viewModel.Flag = !viewModel.Flag;
MessageBox.Show("Flag is " + (viewModel.Flag).ToString());
}
void OnCheck(Document document) {
viewModel.SetSpecicState();
MessageBox.Show("Specific state enabled");
}
void OnUncheck(Document document) {
viewModel.ResetSpecificState();
MessageBox.Show("Specific state disabled");
}
}
}
namespace WindowsFormsApplication1.ViewModels {
public class ViewModelModuleA {
public bool AllowAnotherAction { get; private set; }
internal void DoSomeAction() {
AllowAnotherAction = true;
}
internal void ResetSomeAction() {
AllowAnotherAction = false;
}
}
}
Imports Microsoft.VisualBasic
Imports System.Windows.Forms
Imports DevExpress.XtraBars.Docking2010.Views.WindowsUI
Imports WindowsFormsApplication1.ViewModels
Namespace WindowsFormsApplication1.Views
Public Partial Class ucModuleB
Inherits UserControl
Implements ISupportDocumentActions
Private viewModel As ViewModelModuleB
Public Sub New(ByVal viewModel As ViewModelModuleB)
Me.viewModel = viewModel
InitializeComponent()
End Sub
#Region "ISupportDocumentActions Members"
Public Sub OnQueryDocumentActions(args As IDocumentActionsArgs) Implements ISupportDocumentActions.OnQueryDocumentActions
args.DocumentActions.Add(New DocumentCheckAction(Function() viewModel.Flag, Sub() OnToggle(args.Document)) With { _
.Caption = "Flag" _
})
args.DocumentActions.Add(New DocumentCheckAction(Function() viewModel.IsSpecificState, Sub() OnCheck(args.Document), Sub() OnUncheck(args.Document)) With { _
.Caption = "Specific State" _
})
End Sub
#End Region
Private Sub OnToggle(ByVal document As Document)
viewModel.Flag = Not viewModel.Flag
MessageBox.Show("Flag is " & (viewModel.Flag).ToString())
End Sub
Private Sub OnCheck(ByVal document As Document)
viewModel.SetSpecicState()
MessageBox.Show("Specific state enabled")
End Sub
Private Sub OnUncheck(ByVal document As Document)
viewModel.ResetSpecificState()
MessageBox.Show("Specific state disabled")
End Sub
End Class
End Namespace
Imports Microsoft.VisualBasic
Imports System.Windows.Forms
Imports DevExpress.XtraBars.Docking2010.Views.WindowsUI
Imports WindowsFormsApplication1.ViewModels
Namespace WindowsFormsApplication1.Views
Public Partial Class ucModuleA
Inherits UserControl
Implements ISupportDocumentActions
Private viewModel As ViewModelModuleA
Public Sub New(ByVal viewModel As ViewModelModuleA)
Me.viewModel = viewModel
InitializeComponent()
End Sub
#Region "ISupportDocumentActions Members"
Public Sub OnQueryDocumentActions(args As IDocumentActionsArgs) Implements ISupportDocumentActions.OnQueryDocumentActions
args.DocumentActions.Add(New DocumentAction(Sub(doc) DoAction1(args.Document)) With { _
.Caption = "Action 1" _
})
args.DocumentActions.Add(New DocumentAction(Function(doc) CanDoAction2(args.Document), Sub(doc) DoAction2(args.Document)) With { _
.Caption = "Action 2" _
})
End Sub
#End Region
Private Sub DoAction1(ByVal document As Document)
Dim prev As Boolean = viewModel.AllowAnotherAction
viewModel.DoSomeAction()
MessageBox.Show("Some Action")
If (Not prev) AndAlso prev <> viewModel.AllowAnotherAction Then
MessageBox.Show("Action 2 enabled")
End If
End Sub
Private Function CanDoAction2(ByVal document As Document) As Boolean
Return viewModel.AllowAnotherAction
End Function
Private Sub DoAction2(ByVal document As Document)
MessageBox.Show("Action 2 disabled")
viewModel.ResetSomeAction()
End Sub
End Class
End Namespace
Imports Microsoft.VisualBasic
Imports System
Namespace WindowsFormsApplication1.ViewModels
Public Class ViewModelModuleA
Public Property AllowAnotherAction() As Boolean
Get
Return m_AllowAnotherAction
End Get
Private Set(value As Boolean)
m_AllowAnotherAction = Value
End Set
End Property
Private m_AllowAnotherAction As Boolean
Friend Sub DoSomeAction()
AllowAnotherAction = True
End Sub
Friend Sub ResetSomeAction()
AllowAnotherAction = False
End Sub
End Class
End Namespace
Imports Microsoft.VisualBasic
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text
Namespace WindowsFormsApplication1.ViewModels
Public Class ViewModelModuleB
Public Property Flag() As Boolean
Get
Return m_Flag
End Get
Set(value As Boolean)
m_Flag = Value
End Set
End Property
Private m_Flag As Boolean
Public Property IsSpecificState() As Boolean
Get
Return m_IsSpecificState
End Get
Set(value As Boolean)
m_IsSpecificState = Value
End Set
End Property
Private m_IsSpecificState As Boolean
Public Sub SetSpecicState()
IsSpecificState = True
End Sub
Public Sub ResetSpecificState()
IsSpecificState = False
End Sub
End Class
End Namespace