This example shows how to modify context menu for the Field, Data and Header areas in the PivotGridControl.
- Field Value context menu contains two new items. One command enables the end-user to exclude all fields but the one which is hovered over. Another command copies the filed name to the clipboard.
- Data Area context menu contains a command which enables the end-user to copy cell content to the clipboard.
- Field Header context menu context menu is modified to remove a command which reorders fields.
- Header Area context menu is modified to remove all built-in commands and add a drop-down menu with a single item which enables the end-user to show/hide column grand totals.
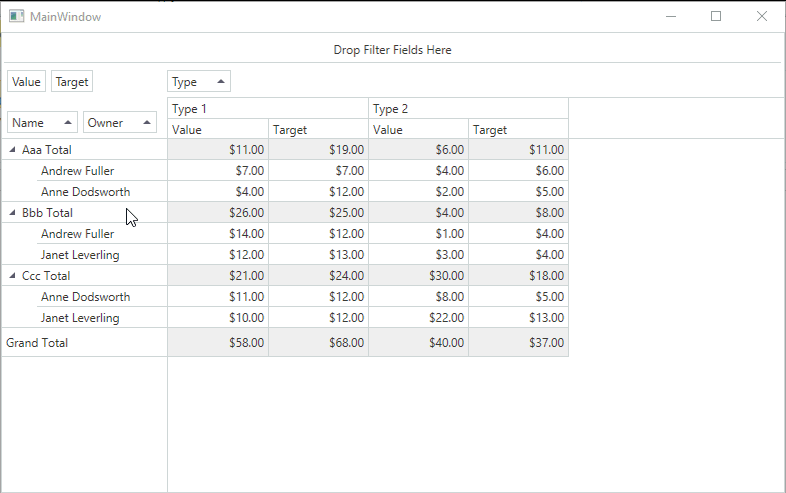
?using DevExpress.Xpf.PivotGrid;
using System.Windows;
namespace WpfPivotGrid_CustomMenu
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
pivotGrid.DataSource = DataHelper.CreatePivotDataSource();
}
private void CopyFieldElementData_ItemClick(object sender, DevExpress.Xpf.Bars.ItemClickEventArgs e)
{
PivotGridFieldValueMenuInfo menuInfo = pivotGrid.GridMenu.MenuInfo as PivotGridFieldValueMenuInfo;
if (menuInfo != null && menuInfo.FieldValueElementData != null &&
menuInfo.FieldValueElementData.Value.ToString() != string.Empty)
{
Clipboard.SetDataObject(menuInfo.FieldValueElementData.Value);
}
}
private void FilterFieldElementData_ItemClick(object sender, DevExpress.Xpf.Bars.ItemClickEventArgs e)
{
PivotGridFieldValueMenuInfo menuInfo = pivotGrid.GridMenu.MenuInfo as PivotGridFieldValueMenuInfo;
if (menuInfo != null && menuInfo.FieldValueElementData != null &&
menuInfo.FieldValueElementData.Value != null &&
menuInfo.FieldValueElementData.Field != null)
{
PivotGridField field = menuInfo.FieldValueElementData.Field;
object value = menuInfo.FieldValueElementData.Value;
field.FilterValues.FilterType = FieldFilterType.Included;
field.FilterValues.Add(value);
}
}
private void CopyCellElementData_ItemClick(object sender, DevExpress.Xpf.Bars.ItemClickEventArgs e)
{
PivotGridCellMenuInfo menuInfo = pivotGrid.GridMenu.MenuInfo as PivotGridCellMenuInfo;
if (menuInfo != null && menuInfo.CellElementData != null &&
menuInfo.CellElementData.Value.ToString() != string.Empty)
{
Clipboard.SetDataObject(menuInfo.CellElementData.DisplayText);
}
}
}
}
?Imports DevExpress.Xpf.PivotGrid
Imports System.Windows
Namespace WpfPivotGrid_CustomMenu
''' <summary>
''' Interaction logic for MainWindow.xaml
''' </summary>
Partial Public Class MainWindow
Inherits Window
Public Sub New()
InitializeComponent()
End Sub
Private Sub Window_Loaded(ByVal sender As Object, ByVal e As RoutedEventArgs)
pivotGrid.DataSource = DataHelper.CreatePivotDataSource()
End Sub
Private Sub CopyFieldElementData_ItemClick(ByVal sender As Object, ByVal e As DevExpress.Xpf.Bars.ItemClickEventArgs)
Dim menuInfo As PivotGridFieldValueMenuInfo = TryCast(pivotGrid.GridMenu.MenuInfo, PivotGridFieldValueMenuInfo)
If menuInfo IsNot Nothing AndAlso menuInfo.FieldValueElementData IsNot Nothing AndAlso menuInfo.FieldValueElementData.Value.ToString() <> String.Empty Then
Clipboard.SetDataObject(menuInfo.FieldValueElementData.Value)
End If
End Sub
Private Sub FilterFieldElementData_ItemClick(ByVal sender As Object, ByVal e As DevExpress.Xpf.Bars.ItemClickEventArgs)
Dim menuInfo As PivotGridFieldValueMenuInfo = TryCast(pivotGrid.GridMenu.MenuInfo, PivotGridFieldValueMenuInfo)
If menuInfo IsNot Nothing AndAlso menuInfo.FieldValueElementData IsNot Nothing AndAlso menuInfo.FieldValueElementData.Value IsNot Nothing AndAlso menuInfo.FieldValueElementData.Field IsNot Nothing Then
Dim field As PivotGridField = menuInfo.FieldValueElementData.Field
Dim value As Object = menuInfo.FieldValueElementData.Value
field.FilterValues.FilterType = FieldFilterType.Included
field.FilterValues.Add(value)
End If
End Sub
Private Sub CopyCellElementData_ItemClick(ByVal sender As Object, ByVal e As DevExpress.Xpf.Bars.ItemClickEventArgs)
Dim menuInfo As PivotGridCellMenuInfo = TryCast(pivotGrid.GridMenu.MenuInfo, PivotGridCellMenuInfo)
If menuInfo IsNot Nothing AndAlso menuInfo.CellElementData IsNot Nothing AndAlso menuInfo.CellElementData.Value.ToString() <> String.Empty Then
Clipboard.SetDataObject(menuInfo.CellElementData.DisplayText)
End If
End Sub
End Class
End Namespace
?<Window
x:Class="WpfPivotGrid_CustomMenu.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:dxpg="http://schemas.devexpress.com/winfx/2008/xaml/pivotgrid"
xmlns:local="clr-namespace:WpfPivotGrid_CustomMenu"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:dxb="http://schemas.devexpress.com/winfx/2008/xaml/bars"
Width="800"
Height="500"
mc:Ignorable="d"
Loaded="Window_Loaded"
Title="MainWindow">
<Grid>
<dxb:BarManager Name="barManager">
<dxb:BarManager.Items>
<dxb:BarCheckItem Name="ShowColumnGrandTotals"
IsChecked="{Binding ElementName=pivotGrid, Path=ShowColumnGrandTotals, Mode=TwoWay}"
Content="Show Column Grand Totals" />
</dxb:BarManager.Items>
<dxpg:PivotGridControl Name="pivotGrid" RowTreeWidth="130">
<dxpg:PivotGridControl.Fields>
<dxpg:PivotGridField Area="RowArea" FieldName="Name" />
<dxpg:PivotGridField Area="RowArea" FieldName="Owner" />
<dxpg:PivotGridField Area="ColumnArea" FieldName="Type" />
<dxpg:PivotGridField Area="DataArea" FieldName="Value" />
<dxpg:PivotGridField Area="DataArea" FieldName="Target" />
</dxpg:PivotGridControl.Fields>
<dxpg:PivotGridControl.FieldValueMenuCustomizations>
<dxb:BarButtonItem Name="CopyFieldElementData" Content="Copy Field Element Value"
ItemClick="CopyFieldElementData_ItemClick"/>
<dxb:BarButtonItem Name="FilterFieldElementData" Content="Filter By Field Element"
ItemClick="FilterFieldElementData_ItemClick"/>
</dxpg:PivotGridControl.FieldValueMenuCustomizations>
<dxpg:PivotGridControl.CellMenuCustomizations>
<dxb:BarButtonItem Name="CopyCellElementData" Content="Copy Cell Data"
ItemClick="CopyCellElementData_ItemClick"/>
</dxpg:PivotGridControl.CellMenuCustomizations>
<dxpg:PivotGridControl.HeaderMenuCustomizations>
<dxb:RemoveBarItemAndLinkAction
ItemName="{x:Static dxpg:DefaultMenuItemNames.FieldOrder}" />
</dxpg:PivotGridControl.HeaderMenuCustomizations>
<dxpg:PivotGridControl.HeaderAreaMenuCustomizations >
<dxb:BarItemSeparator/>
<dxb:BarSubItem Content="Totals">
<dxb:BarSubItem.ItemLinks>
<dxb:BarCheckItemLink BarItemName="ShowColumnGrandTotals" />
</dxb:BarSubItem.ItemLinks>
</dxb:BarSubItem>
<dxb:RemoveBarItemAndLinkAction ItemName="ItemShowPrefilter" />
<dxb:RemoveBarItemAndLinkAction ItemName="ItemHidePrefilter" />
<dxb:RemoveBarItemAndLinkAction ItemName="ItemRefreshData" />
<dxb:RemoveBarItemAndLinkAction ItemName="ItemShowFieldList" />
</dxpg:PivotGridControl.HeaderAreaMenuCustomizations>
</dxpg:PivotGridControl>
</dxb:BarManager>
</Grid>
</Window>