DxGrid.ExpandDetailRow(Int32) Method
Expands the specified detail row.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
public void ExpandDetailRow(
int visibleIndex
)
Parameters
Name |
Type |
Description |
visibleIndex |
Int32 |
A zero-based integer visible index that identifies the detail row.
|
Specify the DetailRowTemplate to enable a master-detail relationship in the grid. A user can click a data row’s expand button to expand/collapse the row and access detail data.
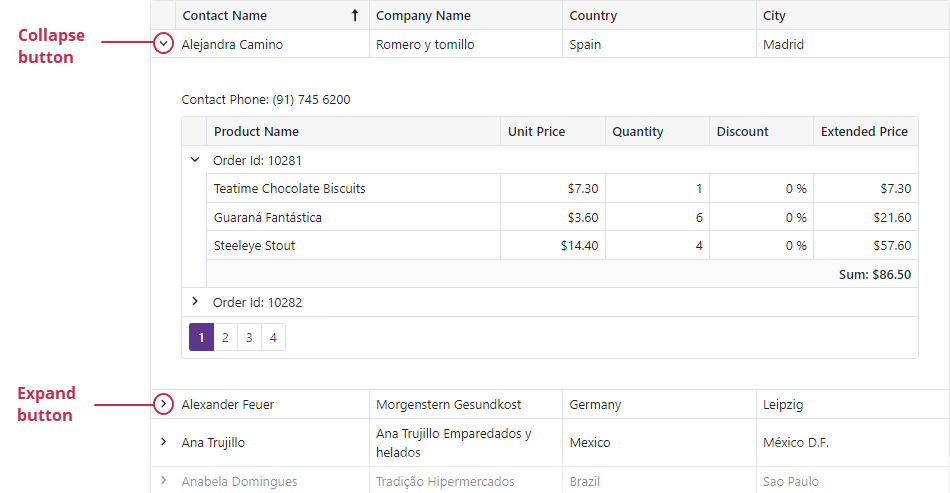
Use the ExpandDetailRow
and CollapseDetailRow(Int32) methods to expand and collapse detail rows in code. To check whether an individual detail row is expanded, use the IsDetailRowExpanded(Int32) method.
The following code snippet checks the first detail row’s expansion state. If the row is expanded, the button click collapses this row and vice versa.
@inject NwindDataService NwindDataService
<DxGrid @ref="Grid"
Data="MasterGridData" >
<Columns>
<DxGridDataColumn FieldName="ContactName" SortIndex="0" />
<DxGridDataColumn FieldName="CompanyName" />
<DxGridDataColumn FieldName="Country" />
<DxGridDataColumn FieldName="City" />
</Columns>
<DetailRowTemplate>
<Grid_MasterDetail_NestedGrid_DetailContent Customer="(Customer)context.DataItem" />
</DetailRowTemplate>
</DxGrid>
<DxButton Click="OnButtonClick" Text="Expand/Collapse the First Detail Row" />
@code {
IGrid Grid { get; set; }
object MasterGridData { get; set; }
protected override async Task OnInitializedAsync() {
MasterGridData = await NwindDataService.GetCustomersAsync();
}
protected override void OnAfterRender(bool firstRender) {
if(firstRender) {
Grid.ExpandDetailRow(0);
}
}
void OnButtonClick() {
if (Grid.IsDetailRowExpanded(0))
Grid.CollapseDetailRow(0);
else
Grid.ExpandDetailRow(0);
}
}
@inject NwindDataService NwindDataService
<div class="mb-2">
Contact Phone: @Customer.Phone
</div>
<DxGrid Data="DetailGridData"
PageSize="5"
AutoExpandAllGroupRows="true">
<Columns>
<DxGridDataColumn FieldName="OrderId" DisplayFormat="d" GroupIndex="0" />
<DxGridDataColumn FieldName="ProductName" Width="40%" />
<DxGridDataColumn FieldName="UnitPrice" DisplayFormat="c" />
<DxGridDataColumn FieldName="Quantity" />
<DxGridDataColumn FieldName="Discount" DisplayFormat="p0" />
<DxGridDataColumn FieldName="ExtendedPrice" DisplayFormat="c" />
</Columns>
<GroupSummary>
<DxGridSummaryItem SummaryType="GridSummaryItemType.Sum"
FieldName="ExtendedPrice"
FooterColumnName="ExtendedPrice" />
</GroupSummary>
</DxGrid>
@code {
[Parameter]
public Customer Customer { get; set; }
object DetailGridData { get; set; }
protected override async Task OnInitializedAsync() {
var invoices = await NwindDataService.GetInvoicesAsync();
DetailGridData = invoices
.Where(i => i.CustomerId == Customer.CustomerId)
.ToArray();
}
}
using System;
using System.Collections.Generic;
using System.Runtime.InteropServices;
namespace BlazorDemo.Data.Northwind {
public partial class Customer {
public Customer() {
Orders = new HashSet<Order>();
}
public string CustomerId { get; set; }
public string CompanyName { get; set; }
public string ContactName { get; set; }
public string ContactTitle { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string Phone { get; set; }
public string Fax { get; set; }
public virtual ICollection<Order> Orders { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.Runtime.InteropServices;
namespace BlazorDemo.Data.Northwind {
public class Invoice {
public string ShipName { get; set; }
public string ShipAddress { get; set; }
public string ShipCity { get; set; }
public string ShipRegion { get; set; }
public string ShipPostalCode { get; set; }
public string ShipCountry { get; set; }
public string CustomerId { get; set; }
public string CustomerName { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string Salesperson { get; set; }
public int OrderId { get; set; }
public DateTime? OrderDate { get; set; }
public DateTime? RequiredDate { get; set; }
public DateTime? ShippedDate { get; set; }
public string ShipperName { get; set; }
public int ProductId { get; set; }
public string ProductName { get; set; }
public decimal UnitPrice { get; set; }
public short Quantity { get; set; }
public float Discount { get; set; }
public decimal? ExtendedPrice { get; set; }
public decimal? Freight { get; set; }
}
}
// ...
builder.Services.AddScoped<NwindDataService>();
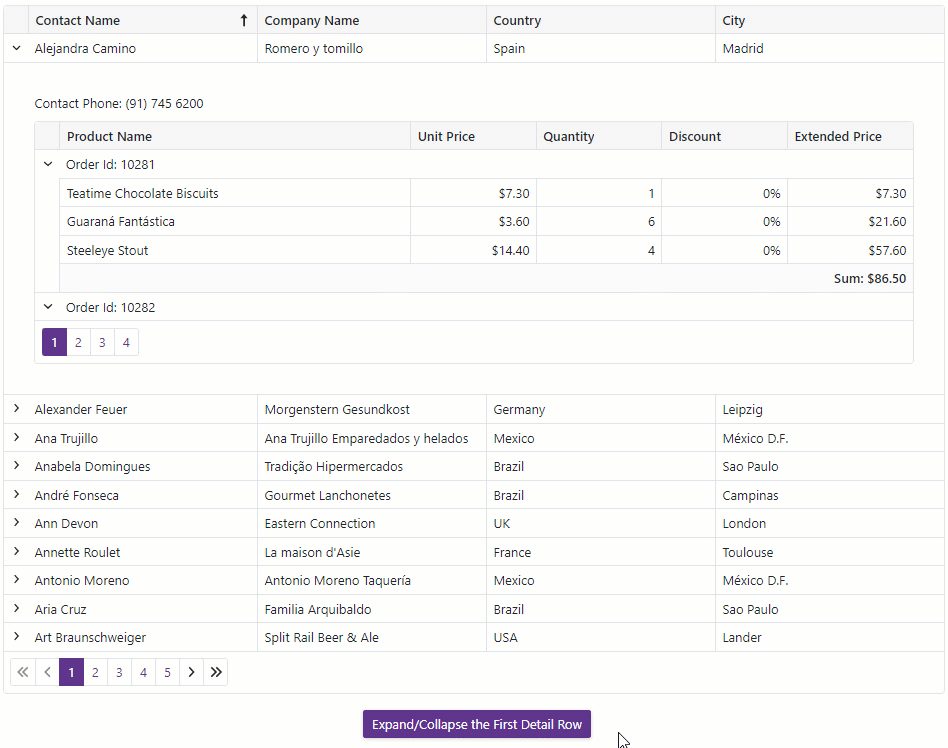
See Also