DxGridDataColumn.GroupIndex Property
Specifies the column’s index among grouped columns.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v23.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[DefaultValue(-1)]
[Parameter]
public int GroupIndex { get; set; }
Property Value
Type |
Default |
Description |
Int32 |
-1 |
The column’s index (zero-based) among grouped columns. -1 if the grid does not group data by this column.
|
Use the GroupIndex
property to group data by an individual column in code. In this case, grouping is applied regardless of the DxGrid.ShowGroupPanel, DxGrid.AllowGroup, and DxGridDataColumn.AllowGroup property values.
If you group data by multiple columns, this property specifies the group level. The lower the value, the higher a column’s group level. For instance, the grid first groups data by a column with GroupIndex = 0
and then by a column with GroupIndex = 1
. If you set this property to -1
, data is not grouped by the corresponding column.
Note
Only one of SortIndex and GroupIndex
can be set to a non-negative value. Otherwise, the Grid raises an exception.
@using Microsoft.EntityFrameworkCore
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
@implements IDisposable
<DxGrid Data="GridDataSource"
ShowGroupPanel="true"
CustomizeCellDisplayText="OnCustomizeCellDisplayText">
<Columns>
<DxGridDataColumn FieldName="OrderDate"
DisplayFormat="d"
GroupIndex="0"
GroupInterval="GridColumnGroupInterval.DateMonth"/>
<DxGridDataColumn FieldName="Customer"
SortMode="GridColumnSortMode.DisplayText"
GroupIndex="1"
GroupInterval="GridColumnGroupInterval.DisplayText" />
<DxGridDataColumn FieldName="Freight"
DisplayFormat="n2" />
</Columns>
</DxGrid>
@code {
object GridDataSource { get; set; }
NorthwindContext Northwind { get; set; }
protected override void OnInitialized() {
Northwind = NorthwindContextFactory.CreateDbContext();
GridDataSource = Northwind.Orders
.Include(i => i.Customer)
.Include(i => i.OrderDetails)
.Include(i => i.ShipViaNavigation)
.ToList();
}
void OnCustomizeCellDisplayText(GridCustomizeCellDisplayTextEventArgs e) {
if (e.FieldName == "Customer")
{
var customer = (Customer)e.Value;
e.DisplayText = $"{customer.CompanyName} ({customer.Country}, {customer.City})";
}
}
public void Dispose() {
Northwind?.Dispose();
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
public virtual DbSet<Category> Categories { get; set; }
public virtual DbSet<Customer> Customers { get; set; }
public virtual DbSet<Invoice> Invoices { get; set; }
public virtual DbSet<Order> Orders { get; set; }
public virtual DbSet<OrderDetail> OrderDetails { get; set; }
public virtual DbSet<Product> Products { get; set; }
public virtual DbSet<Shipper> Shippers { get; set; }
public virtual DbSet<Supplier> Suppliers { get; set; }
public virtual DbSet<Employee> Employees { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder) {
modelBuilder.HasAnnotation("Relational:Collation", "SQL_Latin1_General_CP1_CI_AS");
modelBuilder.Entity<Category>(entity => {
entity.HasIndex(e => e.CategoryName, "CategoryName");
entity.Property(e => e.CategoryId).HasColumnName("CategoryID");
entity.Property(e => e.CategoryName)
.IsRequired()
.HasMaxLength(15);
entity.Property(e => e.Description).HasColumnType("ntext");
entity.Property(e => e.Picture).HasColumnType("image");
});
modelBuilder.Entity<Customer>(entity => {
entity.HasIndex(e => e.City, "City");
entity.HasIndex(e => e.CompanyName, "CompanyName");
entity.HasIndex(e => e.PostalCode, "PostalCode");
entity.HasIndex(e => e.Region, "Region");
entity.Property(e => e.CustomerId)
.HasMaxLength(5)
.HasColumnName("CustomerID")
.IsFixedLength(true);
entity.Property(e => e.Address).HasMaxLength(60);
entity.Property(e => e.City).HasMaxLength(15);
entity.Property(e => e.CompanyName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.ContactName).HasMaxLength(30);
entity.Property(e => e.ContactTitle).HasMaxLength(30);
entity.Property(e => e.Country).HasMaxLength(15);
entity.Property(e => e.Fax).HasMaxLength(24);
entity.Property(e => e.Phone).HasMaxLength(24);
entity.Property(e => e.PostalCode).HasMaxLength(10);
entity.Property(e => e.Region).HasMaxLength(15);
});
modelBuilder.Entity<Invoice>(entity => {
entity.HasNoKey();
entity.ToView("Invoices");
entity.Property(e => e.Address).HasMaxLength(60);
entity.Property(e => e.City).HasMaxLength(15);
entity.Property(e => e.Country).HasMaxLength(15);
entity.Property(e => e.CustomerId)
.HasMaxLength(5)
.HasColumnName("CustomerID")
.IsFixedLength(true);
entity.Property(e => e.CustomerName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.ExtendedPrice).HasColumnType("money");
entity.Property(e => e.Freight).HasColumnType("money");
entity.Property(e => e.OrderDate).HasColumnType("datetime");
entity.Property(e => e.OrderId).HasColumnName("OrderID");
entity.Property(e => e.PostalCode).HasMaxLength(10);
entity.Property(e => e.ProductId).HasColumnName("ProductID");
entity.Property(e => e.ProductName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.Region).HasMaxLength(15);
entity.Property(e => e.RequiredDate).HasColumnType("datetime");
entity.Property(e => e.Salesperson)
.IsRequired()
.HasMaxLength(31);
entity.Property(e => e.ShipAddress).HasMaxLength(60);
entity.Property(e => e.ShipCity).HasMaxLength(15);
entity.Property(e => e.ShipCountry).HasMaxLength(15);
entity.Property(e => e.ShipName).HasMaxLength(40);
entity.Property(e => e.ShipPostalCode).HasMaxLength(10);
entity.Property(e => e.ShipRegion).HasMaxLength(15);
entity.Property(e => e.ShippedDate).HasColumnType("datetime");
entity.Property(e => e.ShipperName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.UnitPrice).HasColumnType("money");
});
modelBuilder.Entity<Order>(entity => {
entity.HasIndex(e => e.CustomerId, "CustomerID");
entity.HasIndex(e => e.CustomerId, "CustomersOrders");
entity.HasIndex(e => e.EmployeeId, "EmployeeID");
entity.HasIndex(e => e.EmployeeId, "EmployeesOrders");
entity.HasIndex(e => e.OrderDate, "OrderDate");
entity.HasIndex(e => e.ShipPostalCode, "ShipPostalCode");
entity.HasIndex(e => e.ShippedDate, "ShippedDate");
entity.HasIndex(e => e.ShipVia, "ShippersOrders");
entity.Property(e => e.OrderId).HasColumnName("OrderID");
entity.Property(e => e.CustomerId)
.HasMaxLength(5)
.HasColumnName("CustomerID")
.IsFixedLength(true);
entity.Property(e => e.EmployeeId).HasColumnName("EmployeeID");
entity.Property(e => e.Freight)
.HasColumnType("money")
.HasDefaultValueSql("((0))");
entity.Property(e => e.OrderDate).HasColumnType("datetime");
entity.Property(e => e.RequiredDate).HasColumnType("datetime");
entity.Property(e => e.ShipAddress).HasMaxLength(60);
entity.Property(e => e.ShipCity).HasMaxLength(15);
entity.Property(e => e.ShipCountry).HasMaxLength(15);
entity.Property(e => e.ShipName).HasMaxLength(40);
entity.Property(e => e.ShipPostalCode).HasMaxLength(10);
entity.Property(e => e.ShipRegion).HasMaxLength(15);
entity.Property(e => e.ShippedDate).HasColumnType("datetime");
entity.HasOne(d => d.Customer)
.WithMany(p => p.Orders)
.HasForeignKey(d => d.CustomerId)
.HasConstraintName("FK_Orders_Customers");
entity.HasOne(d => d.ShipViaNavigation)
.WithMany(p => p.Orders)
.HasForeignKey(d => d.ShipVia)
.HasConstraintName("FK_Orders_Shippers");
});
modelBuilder.Entity<OrderDetail>(entity => {
entity.HasKey(e => new { e.OrderId, e.ProductId })
.HasName("PK_Order_Details");
entity.ToTable("Order Details");
entity.HasIndex(e => e.OrderId, "OrderID");
entity.HasIndex(e => e.OrderId, "OrdersOrder_Details");
entity.HasIndex(e => e.ProductId, "ProductID");
entity.HasIndex(e => e.ProductId, "ProductsOrder_Details");
entity.Property(e => e.OrderId).HasColumnName("OrderID");
entity.Property(e => e.ProductId).HasColumnName("ProductID");
entity.Property(e => e.Quantity).HasDefaultValueSql("((1))");
entity.Property(e => e.UnitPrice).HasColumnType("money");
entity.HasOne(d => d.Order)
.WithMany(p => p.OrderDetails)
.HasForeignKey(d => d.OrderId)
.OnDelete(DeleteBehavior.ClientSetNull)
.HasConstraintName("FK_Order_Details_Orders");
entity.HasOne(d => d.Product)
.WithMany(p => p.OrderDetails)
.HasForeignKey(d => d.ProductId)
.OnDelete(DeleteBehavior.ClientSetNull)
.HasConstraintName("FK_Order_Details_Products");
});
modelBuilder.Entity<Product>(entity => {
entity.HasIndex(e => e.CategoryId, "CategoriesProducts");
entity.HasIndex(e => e.CategoryId, "CategoryID");
entity.HasIndex(e => e.ProductName, "ProductName");
entity.HasIndex(e => e.SupplierId, "SupplierID");
entity.HasIndex(e => e.SupplierId, "SuppliersProducts");
entity.Property(e => e.ProductId).HasColumnName("ProductID");
entity.Property(e => e.CategoryId).HasColumnName("CategoryID");
entity.Property(e => e.ProductName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.QuantityPerUnit).HasMaxLength(20);
entity.Property(e => e.ReorderLevel).HasDefaultValueSql("((0))");
entity.Property(e => e.SupplierId).HasColumnName("SupplierID");
entity.Property(e => e.UnitPrice)
.HasColumnType("money")
.HasDefaultValueSql("((0))");
entity.Property(e => e.UnitsInStock).HasDefaultValueSql("((0))");
entity.Property(e => e.UnitsOnOrder).HasDefaultValueSql("((0))");
entity.HasOne(d => d.Category)
.WithMany(p => p.Products)
.HasForeignKey(d => d.CategoryId)
.HasConstraintName("FK_Products_Categories");
entity.HasOne(d => d.Supplier)
.WithMany(p => p.Products)
.HasForeignKey(d => d.SupplierId)
.HasConstraintName("FK_Products_Suppliers");
});
modelBuilder.Entity<Shipper>(entity => {
entity.Property(e => e.ShipperId).HasColumnName("ShipperID");
entity.Property(e => e.CompanyName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.Phone).HasMaxLength(24);
});
modelBuilder.Entity<Supplier>(entity => {
entity.HasIndex(e => e.CompanyName, "CompanyName");
entity.HasIndex(e => e.PostalCode, "PostalCode");
entity.Property(e => e.SupplierId).HasColumnName("SupplierID");
entity.Property(e => e.Address).HasMaxLength(60);
entity.Property(e => e.City).HasMaxLength(15);
entity.Property(e => e.CompanyName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.ContactName).HasMaxLength(30);
entity.Property(e => e.ContactTitle).HasMaxLength(30);
entity.Property(e => e.Country).HasMaxLength(15);
entity.Property(e => e.Fax).HasMaxLength(24);
entity.Property(e => e.HomePage).HasColumnType("ntext");
entity.Property(e => e.Phone).HasMaxLength(24);
entity.Property(e => e.PostalCode).HasMaxLength(10);
entity.Property(e => e.Region).HasMaxLength(15);
});
modelBuilder.Entity<Employee>(entity => {
entity.HasIndex(e => e.EmployeeId, "EmployeeId");
entity.HasIndex(e => e.LastName, "LastName");
entity.HasIndex(e => e.FirstName, "FirstName");
entity.HasIndex(e => e.Title, "Title");
entity.HasIndex(e => e.BirthDate, "BirthDate");
entity.HasIndex(e => e.HireDate, "HireDate");
entity.HasIndex(e => e.Notes, "Notes");
});
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
using Microsoft.EntityFrameworkCore;
// ...
builder.Services.AddDbContextFactory<NorthwindContext>((sp, options) => {
var env = sp.GetRequiredService<IWebHostEnvironment>();
var dbPath = Path.Combine(env.ContentRootPath, "Northwind-5e44b51f.mdf");
options.UseSqlServer("Server=(localdb)\\MSSQLLocalDB;Integrated Security=true;AttachDbFileName=" + dbPath);
});
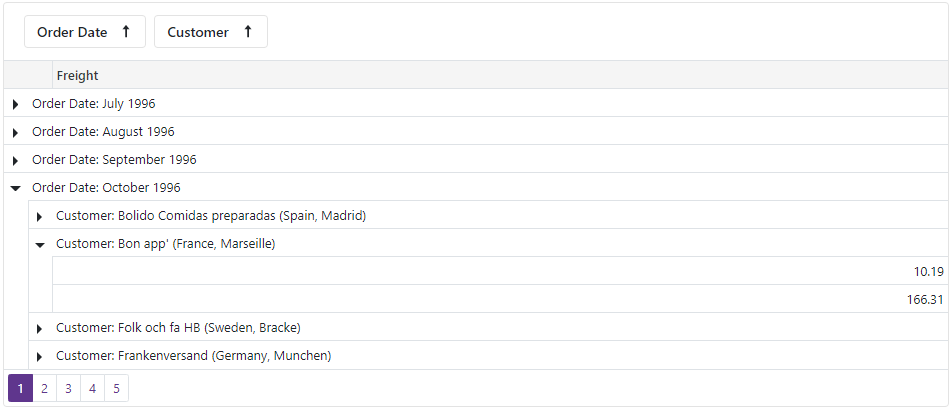
Run Demo: Grid - Group Data
For more information about data grouping in the Grid component, refer to the following topic: Group Data in Blazor Grid.
See Also