DxGrid.PageSizeChanged Event
Fires when the page size changes.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[Parameter]
public EventCallback<int> PageSizeChanged { get; set; }
Parameters
The PageSizeChanged
event fires each time the PageSize property value changes. The event is handled automatically when you use two-way data binding for the PageSize
property (@bind-PageSize
).
You can also handle this event to create a custom response to page size changes.
The following example displays an informational message when a user selects a new value in the page size selector.
@using Microsoft.EntityFrameworkCore
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
@implements IDisposable
<div><b>@PageSizeInfo</b></div>
<DxGrid Data="@Data"
PageSize="@PageSize"
PageSizeSelectorVisible="true"
PageSizeSelectorItems="@(new int[] { 5, 10, 15 })"
PageSizeChanged="OnPageSizeChanged">
<Columns>
<DxGridDataColumn FieldName="ShipName" />
<DxGridDataColumn FieldName="ShipCity" />
<DxGridDataColumn FieldName="ShipCountry" />
<DxGridDataColumn FieldName="Freight" />
<DxGridDataColumn FieldName="OrderDate" />
<DxGridDataColumn FieldName="ShippedDate" />
</Columns>
</DxGrid>
@code {
object Data { get; set; }
NorthwindContext Northwind { get; set; }
int PageSize { get; set; } = 10;
string PageSizeInfo { get; set; } = "Page size is 10";
protected override void OnInitialized() {
Northwind = NorthwindContextFactory.CreateDbContext();
Data = Northwind.Orders.ToList();
}
void OnPageSizeChanged(int newPageSize) {
PageSizeInfo = "Page size was changed from " + PageSize + " to " + newPageSize;
PageSize = newPageSize;
}
public void Dispose() {
Northwind?.Dispose();
}
}
using System;
using System.Collections.Generic;
#nullable disable
namespace Grid.Northwind {
public partial class Order {
public Order() {
OrderDetails = new HashSet<OrderDetail>();
}
public int OrderId { get; set; }
public string CustomerId { get; set; }
public int? EmployeeId { get; set; }
public DateTime? OrderDate { get; set; }
public DateTime? RequiredDate { get; set; }
public DateTime? ShippedDate { get; set; }
public int? ShipVia { get; set; }
public decimal? Freight { get; set; }
public string ShipName { get; set; }
public string ShipAddress { get; set; }
public string ShipCity { get; set; }
public string ShipRegion { get; set; }
public string ShipPostalCode { get; set; }
public string ShipCountry { get; set; }
public virtual Customer Customer { get; set; }
public virtual Shipper ShipViaNavigation { get; set; }
public virtual ICollection<OrderDetail> OrderDetails { get; set; }
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Order> Orders { get; set; }
// ...
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder) {
modelBuilder.HasAnnotation("Relational:Collation", "SQL_Latin1_General_CP1_CI_AS");
// ...
modelBuilder.Entity<Order>(entity => {
entity.HasIndex(e => e.CustomerId, "CustomerID");
entity.HasIndex(e => e.CustomerId, "CustomersOrders");
entity.HasIndex(e => e.EmployeeId, "EmployeeID");
entity.HasIndex(e => e.EmployeeId, "EmployeesOrders");
entity.HasIndex(e => e.OrderDate, "OrderDate");
entity.HasIndex(e => e.ShipPostalCode, "ShipPostalCode");
entity.HasIndex(e => e.ShippedDate, "ShippedDate");
entity.HasIndex(e => e.ShipVia, "ShippersOrders");
entity.Property(e => e.OrderId).HasColumnName("OrderID");
entity.Property(e => e.CustomerId)
.HasMaxLength(5)
.HasColumnName("CustomerID")
.IsFixedLength(true);
entity.Property(e => e.EmployeeId).HasColumnName("EmployeeID");
entity.Property(e => e.Freight)
.HasColumnType("money")
.HasDefaultValueSql("((0))");
entity.Property(e => e.OrderDate).HasColumnType("datetime");
entity.Property(e => e.RequiredDate).HasColumnType("datetime");
entity.Property(e => e.ShipAddress).HasMaxLength(60);
entity.Property(e => e.ShipCity).HasMaxLength(15);
entity.Property(e => e.ShipCountry).HasMaxLength(15);
entity.Property(e => e.ShipName).HasMaxLength(40);
entity.Property(e => e.ShipPostalCode).HasMaxLength(10);
entity.Property(e => e.ShipRegion).HasMaxLength(15);
entity.Property(e => e.ShippedDate).HasColumnType("datetime");
entity.HasOne(d => d.Customer)
.WithMany(p => p.Orders)
.HasForeignKey(d => d.CustomerId)
.HasConstraintName("FK_Orders_Customers");
entity.HasOne(d => d.ShipViaNavigation)
.WithMany(p => p.Orders)
.HasForeignKey(d => d.ShipVia)
.HasConstraintName("FK_Orders_Shippers");
});
// ...
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
using Microsoft.EntityFrameworkCore;
// ...
builder.Services.AddDbContextFactory<NorthwindContext>((sp, options) => {
var env = sp.GetRequiredService<IWebHostEnvironment>();
var dbPath = Path.Combine(env.ContentRootPath, "Northwind-5e44b51f.mdf");
options.UseSqlServer("Server=(localdb)\\MSSQLLocalDB;Integrated Security=true;AttachDbFileName=" + dbPath);
});
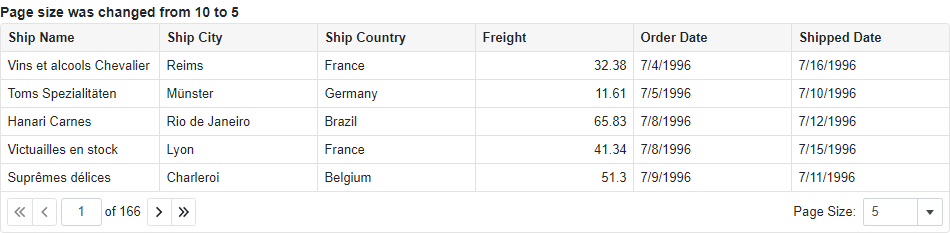
For more information about paging in the Grid component, refer to the following topic: Paging in Blazor Grid.
See Also