DxGrid.DetailRowDisplayMode Property
Specifies when to display detail rows in the Grid.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[DefaultValue(GridDetailRowDisplayMode.Auto)]
[Parameter]
public GridDetailRowDisplayMode DetailRowDisplayMode { get; set; }
Property Value
Available values:
Name |
Description |
Auto
|
Detail rows are visible if the corresponding master row is expanded.
|
Never
|
Detail rows are always hidden. Users cannot expand these rows.
|
Always
|
Detail rows display preview sections under each Grid data row across all columns. These sections can display any content, including tables, values from data source fields, custom text, etc. Users cannot collapse detail rows.
|
The Grid allows users to create master-detail layouts of any complexity. To enable this functionality, specify the DetailRowTemplate.
Use the DetailRowDisplayMode
property to specify when to display detail rows in the Grid. The default property value is Auto
. The Grid shows detail rows if the corresponding master rows are expanded. Otherwise, detail rows are hidden.
Run Demo: Grid Master-Detail View - Nested Grid
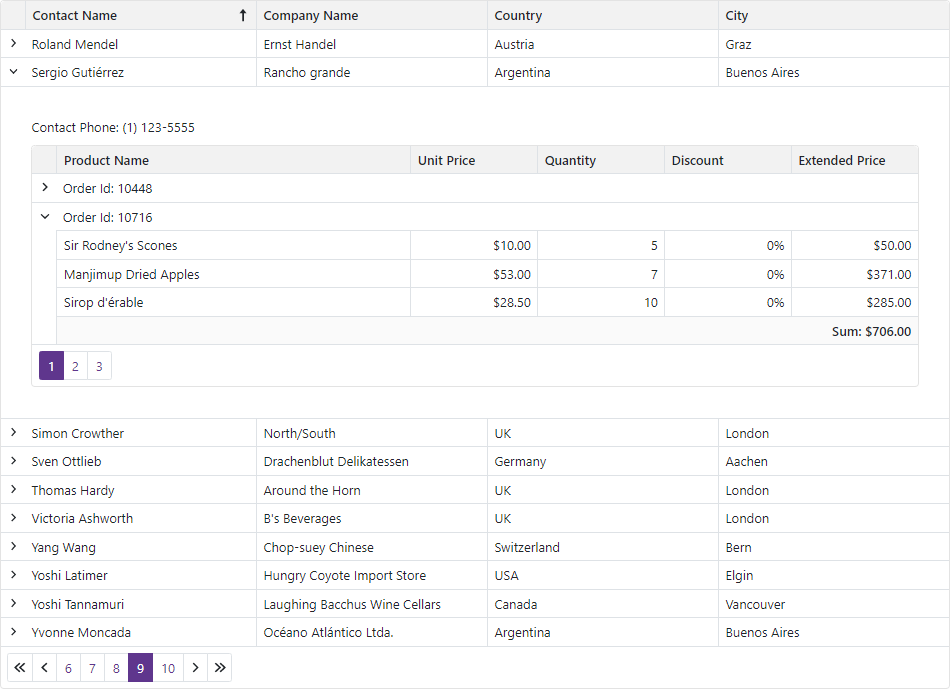
The following example changes DetailRowDisplayMode
to Always
:
@inject NwindDataService NwindDataService
<DxGrid @ref="Grid" Data="MasterGridData" DetailRowDisplayMode="GridDetailRowDisplayMode.Always">
<Columns>
<DxGridDataColumn FieldName="ContactName" SortIndex="0" />
<DxGridDataColumn FieldName="CompanyName" />
<DxGridDataColumn FieldName="Country" />
<DxGridDataColumn FieldName="City" />
</Columns>
<DetailRowTemplate>
<Grid_MasterDetail_NestedGrid_DetailContent Customer="(Customer)context.DataItem" />
</DetailRowTemplate>
</DxGrid>
@code {
IGrid Grid { get; set; }
object MasterGridData { get; set; }
protected override async Task OnInitializedAsync() {
MasterGridData = await NwindDataService.GetCustomersAsync();
}
protected override void OnAfterRender(bool firstRender) {
if(firstRender) {
Grid.ExpandDetailRow(0);
}
}
}
@inject NwindDataService NwindDataService
<div class="mb-2">
Contact Phone: @Customer.Phone
</div>
<DxGrid Data="DetailGridData"
PageSize="5"
AutoExpandAllGroupRows="true">
<Columns>
<DxGridDataColumn FieldName="OrderId" DisplayFormat="d" GroupIndex="0" />
<DxGridDataColumn FieldName="ProductName" Width="40%" />
<DxGridDataColumn FieldName="UnitPrice" DisplayFormat="c" />
<DxGridDataColumn FieldName="Quantity" />
<DxGridDataColumn FieldName="Discount" DisplayFormat="p0" />
<DxGridDataColumn FieldName="ExtendedPrice" DisplayFormat="c" />
</Columns>
<GroupSummary>
<DxGridSummaryItem SummaryType="GridSummaryItemType.Sum"
FieldName="ExtendedPrice"
FooterColumnName="ExtendedPrice" />
</GroupSummary>
</DxGrid>
@code {
[Parameter]
public Customer Customer { get; set; }
object DetailGridData { get; set; }
protected override async Task OnInitializedAsync() {
var invoices = await NwindDataService.GetInvoicesAsync();
DetailGridData = invoices
.Where(i => i.CustomerId == Customer.CustomerId)
.ToArray();
}
}
using System;
using System.Collections.Generic;
using System.Runtime.InteropServices;
namespace BlazorDemo.Data.Northwind {
public partial class Customer {
public Customer() {
Orders = new HashSet<Order>();
}
public string CustomerId { get; set; }
public string CompanyName { get; set; }
public string ContactName { get; set; }
public string ContactTitle { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string Phone { get; set; }
public string Fax { get; set; }
public virtual ICollection<Order> Orders { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.Runtime.InteropServices;
namespace BlazorDemo.Data.Northwind {
public class Invoice {
public string ShipName { get; set; }
public string ShipAddress { get; set; }
public string ShipCity { get; set; }
public string ShipRegion { get; set; }
public string ShipPostalCode { get; set; }
public string ShipCountry { get; set; }
public string CustomerId { get; set; }
public string CustomerName { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string Salesperson { get; set; }
public int OrderId { get; set; }
public DateTime? OrderDate { get; set; }
public DateTime? RequiredDate { get; set; }
public DateTime? ShippedDate { get; set; }
public string ShipperName { get; set; }
public int ProductId { get; set; }
public string ProductName { get; set; }
public decimal UnitPrice { get; set; }
public short Quantity { get; set; }
public float Discount { get; set; }
public decimal? ExtendedPrice { get; set; }
public decimal? Freight { get; set; }
}
}
// ...
builder.Services.AddScoped<NwindDataService>();
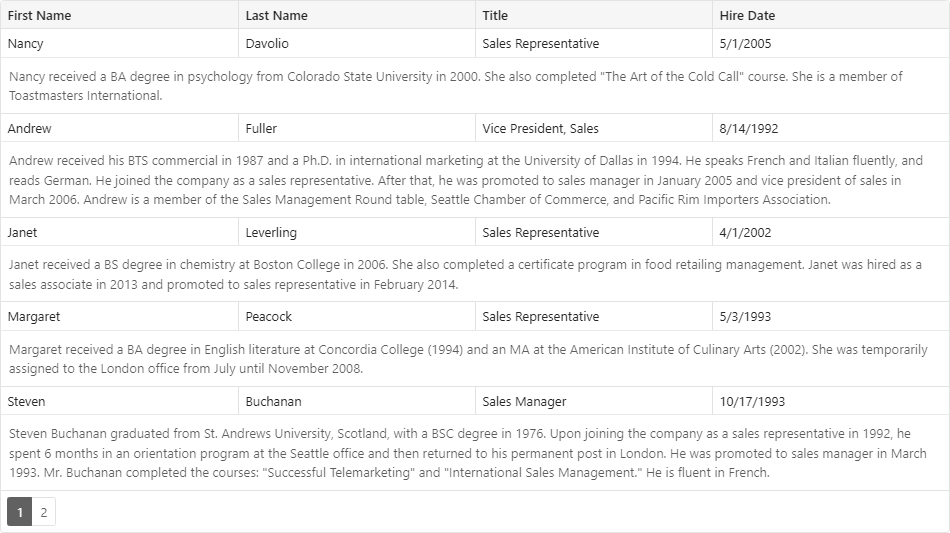
Run Demo: Grid Master-Detail View - Row Preview
See Also