ASPxClientCustomizeExportOptionsEventArgs Class
Allows you to customize the export panel and export options.
Declaration
declare class ASPxClientCustomizeExportOptionsEventArgs extends ASPxClientEventArgs
ASPxClientCustomizeExportOptionsEventArgs objects are automatically created, initialized and passed to the following event handler and callback functions:
constructor(options)
Initializes a new instance of the ASPxClientCustomizeExportOptionsEventArgs
class with the specified export options.
Declaration
constructor(
options: any
)
Parameters
Name |
Type |
Description |
options |
any |
An object that stores export options.
|
Methods
Returns the export options model for the specified export format.
GetExportOptionsModel(
format: any
): any
Type |
Description |
any |
An export options model.
|
You can use an export options model to specify the default export options. The following code snippet, for example, specifies the ‘|” symbol as a separator for CSV data:
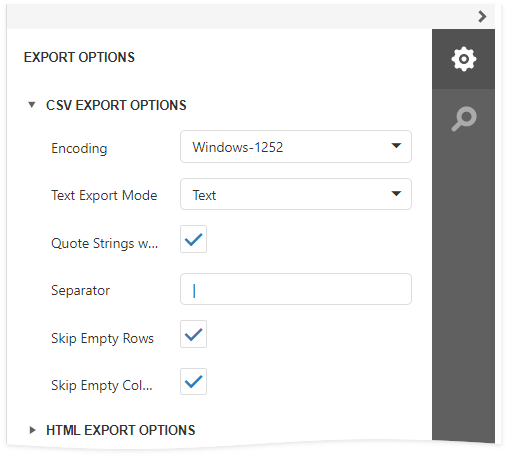
<script type="text/javascript">
function onCustomizeExportOptions(s, e) {
var model = e.GetExportOptionsModel(DevExpress.Reporting.Viewer.ExportFormatID.CSV);
model.separator = '|';
}
</script>
@Html.DevExpress().WebDocumentViewer(settings =>
{
settings.Name = "WebDocumentViewer1";
settings.ClientSideEvents.CustomizeExportOptions = "onCustomizeExportOptions";
}).Bind("XtraReport1").GetHtml()
<script type="text/javascript">
function onCustomizeExportOptions(s, e) {
var model = e.GetExportOptionsModel(DevExpress.Reporting.Viewer.ExportFormatID.CSV);
model.separator = '|';
}
</script>
@{
var viewerRender = Html.DevExpress().WebDocumentViewer("DocumentViewer")
.ClientSideEvents(configure =>
{
configure.CustomizeExportOptions("onCustomizeExportOptions");
})
.Height("1000px")
.Bind("XtraReport1");
@viewerRender.RenderHtml()
}
report-viewer.html
<div>
<dx-report-viewer [reportUrl]="reportUrl" height="800px">
<dxrv-request-options [invokeAction]="invokeAction" [host]="hostUrl">
</dxrv-request-options>
<dxrv-callbacks (CustomizeExportOptions)="OnCustomizeExportOptions($event)">
</dxrv-callbacks>
</dx-report-viewer>
</div>
report-viewer.ts
import { ExportFormatID } from 'devexpress-reporting/dx-webdocumentviewer'
import { Component, Inject, ViewEncapsulation, ViewChild } from '@angular/core';
import { DxReportViewerComponent } from 'devexpress-reporting-angular';
@Component({
// ...
})
export class ReportViewerComponent {
@ViewChild(DxReportViewerComponent, { static: false }) viewer: DxReportViewerComponent;
reportUrl: string = "TestReport";
invokeAction: string = '/DXXRDV';
OnCustomizeExportOptions(event) {
var model = event.args.GetExportOptionsModel(ExportFormatID.CSV);
model.separator = '|';
}
constructor(@Inject('BASE_URL') public hostUrl: string) { }
}
'use client';
import ReportViewer, { RequestOptions, Callbacks } from 'devexpress-reporting-react/dx-report-viewer';
import { ExportFormatID } from 'devexpress-reporting/dx-webdocumentviewer'
function App() {
const onCustomizeExportOptions = ({ args }: { args: any }): void => {
var model = args.GetExportOptionsModel(ExportFormatID.CSV);
model.separator = '|';
args.HideProperties(ExportFormatID.XLS, "ExportMode", "PageRange");
args.HideProperties(ExportFormatID.XLSX);
};
return (
<ReportViewer reportUrl="TestReport">
<RequestOptions host="http://localhost:5000/" invokeAction="DXXRDV" />
<Callbacks CustomizeExportOptions={onCustomizeExportOptions} />
</ReportViewer>
)
}
export default App
import { ExportFormatID } from 'devexpress-reporting/dx-webdocumentviewer'
import ko from "knockout";
var componentData = {
name: "WebDocumentViewer",
mounted() {
// ...
var callbacks = {
CustomizeExportOptions: function(s, e){
var model = e.GetExportOptionsModel(ExportFormatID.CSV);
model.separator = '|';
}
};
ko.applyBindings({
// ..
callbacks: callbacks
}, this.$refs.viewer);
},
// ...
};
export default componentData;
To specify a document format in the method’s parameter, use the ExportFormatID enumeration value.
HideExportOptionsPanel Method
Hides the Export Options panel in the Web Document Viewer.
Declaration
HideExportOptionsPanel(): void
The following code snippet hides the Export Options panel in the Web Document Viewer:
<script type="text/javascript">
function onCustomizeExportOptions(s, e) {
e.HideExportOptionsPanel();
}
</script>
@Html.DevExpress().WebDocumentViewer(settings =>
{
settings.Name = "WebDocumentViewer1";
settings.ClientSideEvents.CustomizeExportOptions = "onCustomizeExportOptions";
}).Bind("XtraReport1").GetHtml()
<script type="text/javascript">
function onCustomizeExportOptions(s, e) {
e.HideExportOptionsPanel();
}
</script>
@{
var viewerRender = Html.DevExpress().WebDocumentViewer("DocumentViewer")
.ClientSideEvents(configure =>
{
configure.CustomizeExportOptions("onCustomizeExportOptions");
})
.Height("1000px")
.Bind("XtraReport1");
@viewerRender.RenderHtml()
}
report-viewer.html
<div>
<dx-report-viewer [reportUrl]="reportUrl" height="800px">
<dxrv-request-options [invokeAction]="invokeAction" [host]="hostUrl">
</dxrv-request-options>
<dxrv-callbacks (CustomizeExportOptions)="OnCustomizeExportOptions($event)">
</dxrv-callbacks>
</dx-report-viewer>
</div>
report-viewer.ts
import { Component, Inject, ViewEncapsulation, ViewChild } from '@angular/core';
import { DxReportViewerComponent } from 'devexpress-reporting-angular';
@Component({
// ...
})
export class ReportViewerComponent {
@ViewChild(DxReportViewerComponent, { static: false }) viewer: DxReportViewerComponent;
reportUrl: string = "TestReport";
invokeAction: string = '/DXXRDV';
OnCustomizeExportOptions(event) {
e.HideExportOptionsPanel();
}
constructor(@Inject('BASE_URL') public hostUrl: string) { }
}
'use client';
import React from 'react';
import ReportViewer, { Callbacks, DxReportViewerRef, RequestOptions } from 'devexpress-reporting-react/dx-report-viewer';
import { ExportFormatID, AsyncExportApproach } from 'devexpress-reporting/dx-webdocumentviewer'
function App() {
const viewerRef = React.useRef<DxReportViewerRef>();
const onCustomizeExportOptions = (event: any): void => {
event.args.HideExportOptionsPanel();
var model = event.args.GetExportOptionsModel(ExportFormatID.XLSX);
// Encrypt the file. Encryption is performed in asynchronous mode.
//model.encryptionOptions.password = "1234";
model.documentOptions.author = "Me";
};
const onBeforeRender = (event: any): void => {
AsyncExportApproach(true);
};
const exportDocument = () => viewerRef.current?.instance().ExportTo('xlsx');
return (
<>
<button onClick={exportDocument}>Export to XLSX</button>
<ReportViewer ref={viewerRef} reportUrl="TestReport">
<RequestOptions host="http://localhost:5000/" invokeAction="/DXXRDV" />
<Callbacks CustomizeExportOptions={onCustomizeExportOptions} BeforeRender={onBeforeRender} />
</ReportViewer>
</>
)
}
export default App
<script>
import ko from "knockout";
var componentData = {
name: "WebDocumentViewer",
mounted() {
// ...
var callbacks = {
CustomizeExportOptions: function(s, e){
e.HideExportOptionsPanel();
}
};
ko.applyBindings({
// ..
callbacks: callbacks
}, this.$refs.viewer);
},
// ...
};
export default componentData;
</script>
Removes the specified export format from the Export To drop-down list and from the Export Options panel.
HideFormat(
format: any
): void
Name |
Type |
Description |
format |
any |
An ExportFormatID enumeration value that specifies the export format to hide.
|
The following code snippet removes the XLS format from the Export To drop-down list and from the Export Options panel:
<script type="text/javascript">
function onCustomizeExportOptions(s, e) {
e.HideFormat(DevExpress.Reporting.Viewer.ExportFormatID.XLS);
}
</script>
@Html.DevExpress().WebDocumentViewer(settings =>
{
settings.Name = "WebDocumentViewer1";
settings.ClientSideEvents.CustomizeExportOptions = "onCustomizeExportOptions";
}).Bind("XtraReport1").GetHtml()
<script type="text/javascript">
function onCustomizeExportOptions(s, e) {
e.HideFormat(DevExpress.Reporting.Viewer.ExportFormatID.XLS);
}
</script>
@{
var viewerRender = Html.DevExpress().WebDocumentViewer("DocumentViewer")
.ClientSideEvents(configure =>
{
configure.CustomizeExportOptions("onCustomizeExportOptions");
})
.Height("1000px")
.Bind("XtraReport1");
@viewerRender.RenderHtml()
}
report-viewer.html
<div>
<dx-report-viewer [reportUrl]="reportUrl" height="800px">
<dxrv-request-options [invokeAction]="invokeAction" [host]="hostUrl">
</dxrv-request-options>
<dxrv-callbacks (CustomizeExportOptions)="OnCustomizeExportOptions($event)">
</dxrv-callbacks>
</dx-report-viewer>
</div>
report-viewer.ts
import { ExportFormatID } from 'devexpress-reporting/dx-webdocumentviewer'
import { Component, Inject, ViewEncapsulation, ViewChild } from '@angular/core';
import { DxReportViewerComponent } from 'devexpress-reporting-angular';
@Component({
// ...
})
export class ReportViewerComponent {
@ViewChild(DxReportViewerComponent, { static: false }) viewer: DxReportViewerComponent;
reportUrl: string = "TestReport";
invokeAction: string = '/DXXRDV';
OnCustomizeExportOptions(event) {
e.HideFormat(ExportFormatID.XLS);
}
constructor(@Inject('BASE_URL') public hostUrl: string) { }
}
'use client';
import ReportViewer, { RequestOptions, Callbacks } from 'devexpress-reporting-react/dx-report-viewer';
import { ExportFormatID } from 'devexpress-reporting/dx-webdocumentviewer';
function App() {
const onCustomizeExportOptions = ({ args }: { args: any }): void => {
args.HideFormat(ExportFormatID.XLS);
};
return (
<ReportViewer reportUrl="TestExportReport">
<RequestOptions host="http://localhost:5000/" invokeAction="DXXRDV" />
<Callbacks CustomizeExportOptions={onCustomizeExportOptions} />
</ReportViewer>
)
}
export default App
<script>
import ko from "knockout";
import { ExportFormatID } from 'devexpress-reporting/dx-webdocumentviewer'
var componentData = {
name: "WebDocumentViewer",
mounted() {
// ...
var callbacks = {
CustomizeExportOptions: function(s, e){
e.HideFormat(ExportFormatID.XLS);
}
};
ko.applyBindings({
// ..
callbacks: callbacks
}, this.$refs.viewer);
},
// ...
};
export default componentData;
</script>
Hides the specified options for the designated export format from the Export Options panel in the Document Viewer.
HideProperties(
format: any,
... properties: any[]
): void
Name |
Type |
Description |
format |
any |
An ExportFormatID enumeration value that specifies the export format whose options should be hidden.
|
properties |
any[] |
An array of properties to hide. If this parameter is omitted, all export options for the specified document format are hidden.
|
The code snippet below hides the following export options in the Document Viewer’s Export Options panel:
- ExportMode option for the XLS format
- PageRange option for the XLS format
- all options for the XLSX format.
<script type="text/javascript">
function onCustomizeExportOptions(s, e) {
e.HideProperties(DevExpress.Reporting.Viewer.ExportFormatID.XLS, "ExportMode", "PageRange");
e.HideProperties(DevExpress.Reporting.Viewer.ExportFormatID.XLSX);
}
</script>
@Html.DevExpress().WebDocumentViewer(settings =>
{
settings.Name = "WebDocumentViewer1";
settings.ClientSideEvents.CustomizeExportOptions = "onCustomizeExportOptions";
}).Bind("XtraReport1").GetHtml()
<script type="text/javascript">
function onCustomizeExportOptions(s, e) {
e.HideProperties(DevExpress.Reporting.Viewer.ExportFormatID.XLS, "ExportMode", "PageRange");
e.HideProperties(DevExpress.Reporting.Viewer.ExportFormatID.XLSX);
}
</script>
@{
var viewerRender = Html.DevExpress().WebDocumentViewer("DocumentViewer")
.ClientSideEvents(configure =>
{
configure.CustomizeExportOptions("onCustomizeExportOptions");
})
.Height("1000px")
.Bind("XtraReport1");
@viewerRender.RenderHtml()
}
report-viewer.html
<div>
<dx-report-viewer [reportUrl]="reportUrl" height="800px">
<dxrv-request-options [invokeAction]="invokeAction" [host]="hostUrl">
</dxrv-request-options>
<dxrv-callbacks (CustomizeExportOptions)="OnCustomizeExportOptions($event)">
</dxrv-callbacks>
</dx-report-viewer>
</div>
report-viewer.ts
import { Component, Inject, ViewEncapsulation, ViewChild } from '@angular/core';
import { DxReportViewerComponent } from 'devexpress-reporting-angular';
import { ExportFormatID } from 'devexpress-reporting/dx-webdocumentviewer'
@Component({
// ...
})
export class ReportViewerComponent {
@ViewChild(DxReportViewerComponent, { static: false }) viewer: DxReportViewerComponent;
reportUrl: string = "TestReport";
invokeAction: string = '/DXXRDV';
OnCustomizeExportOptions(event) {
e.HideProperties(ExportFormatID.XLS, "ExportMode", "PageRange");
e.HideProperties(ExportFormatID.XLSX);
}
constructor(@Inject('BASE_URL') public hostUrl: string) { }
}
'use client';
import ReportViewer, { RequestOptions, Callbacks } from 'devexpress-reporting-react/dx-report-viewer';
import { ExportFormatID } from 'devexpress-reporting/dx-webdocumentviewer'
function App() {
const onCustomizeExportOptions = ({ args }: { args: any }): void => {
var model = args.GetExportOptionsModel(ExportFormatID.CSV);
model.separator = '|';
args.HideProperties(ExportFormatID.XLS, "ExportMode", "PageRange");
args.HideProperties(ExportFormatID.XLSX);
};
return (
<ReportViewer reportUrl="TestReport">
<RequestOptions host="http://localhost:5000/" invokeAction="DXXRDV" />
<Callbacks CustomizeExportOptions={onCustomizeExportOptions} />
</ReportViewer>
)
}
export default App
<script>
import ko from "knockout";
import { ExportFormatID } from 'devexpress-reporting/dx-webdocumentviewer'
var componentData = {
name: "WebDocumentViewer",
mounted() {
// ...
var callbacks = {
CustomizeExportOptions: function(s, e){
e.HideProperties(ExportFormatID.XLS, "ExportMode", "PageRange");
e.HideProperties(ExportFormatID.XLSX);
}
};
ko.applyBindings({
// ..
callbacks: callbacks
}, this.$refs.viewer);
},
// ...
};
export default componentData;
</script>