The WinUIMessageBoxService implements the IMessageBoxService interface and uses the WinUIMessageBox control to display messages.
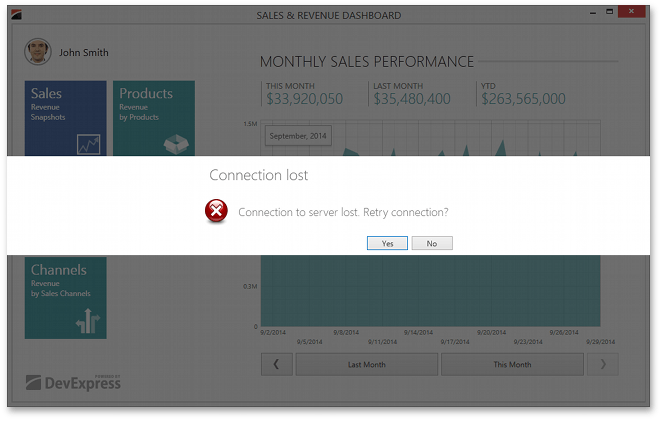
Note
The message box stretches to fit the window’s width when you use the WinUIMessageBoxService.
Example
You can use the WinUIMessageBoxService instead of the DXMessageBoxService. The following example demonstrates how to use the WinUIMessageBoxService:
Imports DevExpress.Mvvm
Imports System.Windows.Input
Namespace Example.ViewModel
Public Class MainViewModel
Protected Overridable ReadOnly Property MessageBoxService() As IMessageBoxService
Get
Return Nothing
End Get
End Property
Public Sub ShowMessage()
MessageBoxService.Show("This is MainView!")
End Sub
End Class
End Namespace
Imports DevExpress.Mvvm
Imports DevExpress.Mvvm.DataAnnotations
Namespace Example.ViewModel
Public Class ChildViewModel
<ServiceProperty(SearchMode:=ServiceSearchMode.PreferParents)> _
Protected Overridable ReadOnly Property MessageBoxService() As IMessageBoxService
Get
Return Nothing
End Get
End Property
Public Sub ShowMessage()
MessageBoxService.Show("This is ChildView")
End Sub
End Class
End Namespace
<UserControl x:Class="Example.View.ChildView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:ViewModel="clr-namespace:Example.ViewModel"
xmlns:dxmvvm="http://schemas.devexpress.com/winfx/2008/xaml/mvvm"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300"
DataContext="{dxmvvm:ViewModelSource ViewModel:ChildViewModel}">
<Grid>
<Button Content="ChildView: Show Message" Command="{Binding ShowMessageCommand}"/>
</Grid>
</UserControl>
<UserControl x:Class="Example.View.MainView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:ViewModel="clr-namespace:Example.ViewModel"
xmlns:View="clr-namespace:Example.View"
xmlns:dxmvvm="http://schemas.devexpress.com/winfx/2008/xaml/mvvm"
xmlns:dx="http://schemas.devexpress.com/winfx/2008/xaml/core"
xmlns:dxwui="http://schemas.devexpress.com/winfx/2008/xaml/windowsui"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" d:DesignHeight="500" d:DesignWidth="600"
DataContext="{dxmvvm:ViewModelSource ViewModel:MainViewModel}">
<dxmvvm:Interaction.Behaviors>
<dxwui:WinUIMessageBoxService/>
</dxmvvm:Interaction.Behaviors>
<Grid x:Name="LayoutRoot" Background="White">
<StackPanel Orientation="Vertical">
<StackPanel Orientation="Horizontal">
<TextBlock Text="MainView: "/>
<Button Content="Show Message" Command="{Binding ShowMessageCommand}"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<TextBlock Text="ChildView: " />
<View:ChildView dxmvvm:ViewModelExtensions.ParentViewModel="{Binding DataContext, ElementName=LayoutRoot}"/>
</StackPanel>
</StackPanel>
</Grid>
</UserControl>
using DevExpress.Mvvm;
using DevExpress.Mvvm.DataAnnotations;
namespace Example.ViewModel {
public class ChildViewModel {
[ServiceProperty(SearchMode=ServiceSearchMode.PreferParents)]
protected virtual IMessageBoxService MessageBoxService { get { return null; } }
public void ShowMessage() {
MessageBoxService.Show("This is ChildView");
}
}
}
using DevExpress.Mvvm;
using System.Windows.Input;
namespace Example.ViewModel {
public class MainViewModel {
protected virtual IMessageBoxService MessageBoxService { get { return null; } }
public void ShowMessage() {
MessageBoxService.Show("This is MainView!");
}
}
}