DxComboBoxSettings.EditFormat Property
Specifies the format pattern applied to the value of the focused combo box editor.
Namespace: DevExpress.Blazor
Assembly:
DevExpress.Blazor.v24.1.dll
NuGet Package:
DevExpress.Blazor
Declaration
[DefaultValue(null)]
[Parameter]
public string EditFormat { get; set; }
Property Value
Type |
Default |
Description |
String |
null |
The format pattern.
|
Specify the DisplayFormat property to format the unfocused combo box editor’s display value. To format the focused editor’s edit value, use the EditFormat
property. If the EditFormat
property is unspecified, the TextFieldName property specifies the editor display text.
The EditFormat
property allows you to format edit values displayed in single-column and multi-column combo boxes. To change formatting at runtime, use the IComboBoxSettings.EditFormat property.
Note
A multi-column combo box applies the filter condition only to the columns displayed in the edit value.
The following code snippet applies the following formats to the combo box editor:
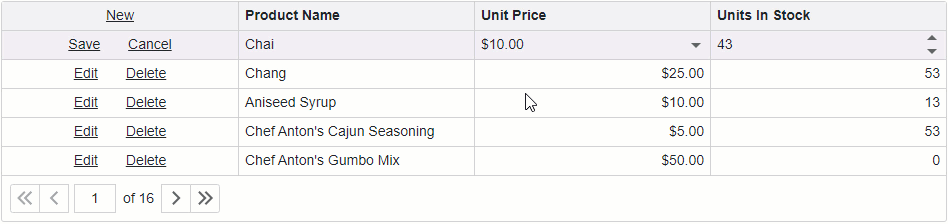
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
<DxGrid Data="Products"
EditMode="GridEditMode.EditRow">
<Columns>
<DxGridCommandColumn />
<DxGridDataColumn FieldName="ProductName" Width="25%" />
<DxGridDataColumn FieldName="UnitPrice">
<EditSettings>
<DxComboBoxSettings Data="Prices" DisplayFormat="C" EditFormat="F" />
</EditSettings>
</DxGridDataColumn>
<DxGridDataColumn FieldName="UnitsInStock" />
</Columns>
</DxGrid>
@code {
NorthwindContext Northwind { get; set; }
List<Product> Products { get; set; }
IEnumerable<decimal?> Prices = new List<decimal?>() { 5, 10, 25, 50, 100};
protected override async Task OnInitializedAsync() {
Northwind = NorthwindContextFactory.CreateDbContext();
Products = await Northwind.Products.ToListAsync();
}
public void Dispose() {
Northwind?.Dispose();
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Product> Products { get; set; }
// ...
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder) {
modelBuilder.HasAnnotation("Relational:Collation", "SQL_Latin1_General_CP1_CI_AS");
// ...
modelBuilder.Entity<Product>(entity => {
entity.HasIndex(e => e.CategoryId, "CategoriesProducts");
entity.HasIndex(e => e.CategoryId, "CategoryID");
entity.HasIndex(e => e.ProductName, "ProductName");
entity.HasIndex(e => e.SupplierId, "SupplierID");
entity.HasIndex(e => e.SupplierId, "SuppliersProducts");
entity.Property(e => e.ProductId).HasColumnName("ProductID");
entity.Property(e => e.CategoryId).HasColumnName("CategoryID");
entity.Property(e => e.ProductName)
.IsRequired()
.HasMaxLength(40);
entity.Property(e => e.QuantityPerUnit).HasMaxLength(20);
entity.Property(e => e.ReorderLevel).HasDefaultValueSql("((0))");
entity.Property(e => e.SupplierId).HasColumnName("SupplierID");
entity.Property(e => e.UnitPrice)
.HasColumnType("money")
.HasDefaultValueSql("((0))");
entity.Property(e => e.UnitsInStock).HasDefaultValueSql("((0))");
entity.Property(e => e.UnitsOnOrder).HasDefaultValueSql("((0))");
entity.HasOne(d => d.Category)
.WithMany(p => p.Products)
.HasForeignKey(d => d.CategoryId)
.HasConstraintName("FK_Products_Categories");
});
// ...
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
public partial class Product {
public Product() {
OrderDetails = new HashSet<OrderDetail>();
}
public int ProductId { get; set; }
public string ProductName { get; set; }
public int? SupplierId { get; set; }
public int? CategoryId { get; set; }
public string QuantityPerUnit { get; set; }
public decimal? UnitPrice { get; set; }
public short? UnitsInStock { get; set; }
public short? UnitsOnOrder { get; set; }
public short? ReorderLevel { get; set; }
public bool Discontinued { get; set; }
public virtual Category Category { get; set; }
}
The following code snippet adds three columns to the combo box editor and applies the {1} {2}
format pattern to the edit value. This format specifies that the editor should display column values with listed visible indexes:
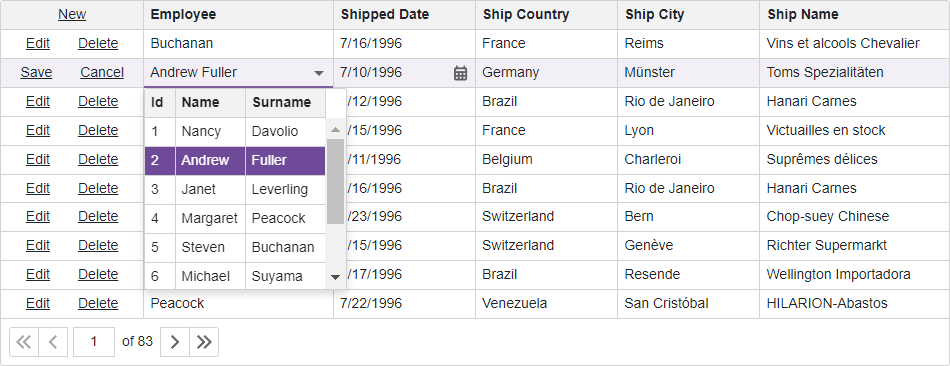
@inject IDbContextFactory<NorthwindContext> NorthwindContextFactory
<DxGrid Data="Orders"
EditMode="GridEditMode.EditRow">
<Columns>
<DxGridCommandColumn />
<DxGridDataColumn FieldName="EmployeeId" Caption="Employee" Width="20%">
<EditSettings>
<DxComboBoxSettings Data="Employees"
TextFieldName="LastName"
ValueFieldName="EmployeeId"
EditFormat="{1} {2}" >
<Columns>
<DxListEditorColumn FieldName="EmployeeId" Caption="Id" Width="30px" />
<DxListEditorColumn FieldName="FirstName" Caption="Name" />
<DxListEditorColumn FieldName="LastName" Caption="Surname" />
</Columns>
</DxComboBoxSettings>
</EditSettings>
</DxGridDataColumn>
<DxGridDataColumn FieldName="ShippedDate" />
<DxGridDataColumn FieldName="ShipCountry" />
<DxGridDataColumn FieldName="ShipCity" />
<DxGridDataColumn FieldName="ShipName" Width="20%" />
</Columns>
</DxGrid>
@code {
NorthwindContext Northwind { get; set; }
List<Order> Orders { get; set; }
List<Employee> Employees { get; set; }
protected override async Task OnInitializedAsync() {
Northwind = NorthwindContextFactory.CreateDbContext();
Orders = await Northwind.Orders.ToListAsync();
Employees = await Northwind.Employees.ToListAsync();
}
public void Dispose() {
Northwind?.Dispose();
}
}
using Microsoft.EntityFrameworkCore;
#nullable disable
namespace Grid.Northwind {
public partial class NorthwindContext : DbContext {
public NorthwindContext(DbContextOptions<NorthwindContext> options)
: base(options) {
}
// ...
public virtual DbSet<Order> Orders { get; set; }
public virtual DbSet<Employee> Employees { get; set; }
// ...
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) {
if(!optionsBuilder.IsConfigured) {
optionsBuilder.UseSqlServer("Server=.\\sqlexpress;Database=Northwind;Integrated Security=true");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder) {
modelBuilder.HasAnnotation("Relational:Collation", "SQL_Latin1_General_CP1_CI_AS");
// ...
modelBuilder.Entity<Order>(entity => {
entity.HasIndex(e => e.CustomerId, "CustomerID");
entity.HasIndex(e => e.CustomerId, "CustomersOrders");
entity.HasIndex(e => e.EmployeeId, "EmployeeID");
entity.HasIndex(e => e.EmployeeId, "EmployeesOrders");
entity.HasIndex(e => e.OrderDate, "OrderDate");
entity.HasIndex(e => e.ShipPostalCode, "ShipPostalCode");
entity.HasIndex(e => e.ShippedDate, "ShippedDate");
entity.HasIndex(e => e.ShipVia, "ShippersOrders");
entity.Property(e => e.OrderId).HasColumnName("OrderID");
entity.Property(e => e.CustomerId)
.HasMaxLength(5)
.HasColumnName("CustomerID")
.IsFixedLength(true);
entity.Property(e => e.EmployeeId).HasColumnName("EmployeeID");
entity.Property(e => e.Freight)
.HasColumnType("money")
.HasDefaultValueSql("((0))");
entity.Property(e => e.OrderDate).HasColumnType("datetime");
entity.Property(e => e.RequiredDate).HasColumnType("datetime");
entity.Property(e => e.ShipAddress).HasMaxLength(60);
entity.Property(e => e.ShipCity).HasMaxLength(15);
entity.Property(e => e.ShipCountry).HasMaxLength(15);
entity.Property(e => e.ShipName).HasMaxLength(40);
entity.Property(e => e.ShipPostalCode).HasMaxLength(10);
entity.Property(e => e.ShipRegion).HasMaxLength(15);
entity.Property(e => e.ShippedDate).HasColumnType("datetime");
});
modelBuilder.Entity<Employee>(entity => {
entity.HasIndex(e => e.EmployeeId, "EmployeeId");
entity.HasIndex(e => e.LastName, "LastName");
entity.HasIndex(e => e.FirstName, "FirstName");
entity.HasIndex(e => e.Title, "Title");
entity.HasIndex(e => e.BirthDate, "BirthDate");
entity.HasIndex(e => e.HireDate, "HireDate");
entity.HasIndex(e => e.Notes, "Notes");
});
// ...
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
public partial class Order {
public Order() {
OrderDetails = new HashSet<OrderDetail>();
}
public int OrderId { get; set; }
public string CustomerId { get; set; }
public int? EmployeeId { get; set; }
public DateTime? OrderDate { get; set; }
public DateTime? RequiredDate { get; set; }
public DateTime? ShippedDate { get; set; }
public int? ShipVia { get; set; }
public decimal? Freight { get; set; }
public string ShipName { get; set; }
public string ShipAddress { get; set; }
public string ShipCity { get; set; }
public string ShipRegion { get; set; }
public string ShipPostalCode { get; set; }
public string ShipCountry { get; set; }
}
public partial class Employee {
public int EmployeeId { get; set; }
public string LastName { get; set; }
public string FirstName { get; set; }
public string Title { get; set; }
public string TitleOfCourtesy { get; set; }
public Nullable<System.DateTime> BirthDate { get; set; }
public Nullable<System.DateTime> HireDate { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string Region { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string HomePhone { get; set; }
public string Extension { get; set; }
public byte[] Photo { get; set; }
public string Notes { get; set; }
public Nullable<int> ReportsTo { get; set; }
public string PhotoPath { get; set; }
public virtual ICollection<Order> Orders { get; set; }
public string Text => $"{FirstName} {LastName} ({Title})";
public string ImageFileName => $"Employees/{EmployeeId}.jpg";
}
See Also