This tutorial shows how to add the DashboardEFDataSource to an in-memory data source storage, and make it available to users. The MDF database is used as a sample.
In your application, add the NWind.mdf database to the App_Data folder from the C:\Users\Public\Documents\DevExpress Demos 23.2\Components\Data directory.
In Web.config, specify a connection string to the database.
<configuration>
<connectionStrings>
<add name="NWindConnectionString" connectionString="data source=(localdb)\mssqllocaldb;attachdbfilename=|DataDirectory|\NWind.mdf;integrated security=True;connect timeout=120" providerName="System.Data.SqlClient" />
</connectionStrings>
</configuration>
- Right-click the project and select Add | ADO.NET Entity Data Model. Set OrdersContext as a model name and select the Code First from database model type in the invoked wizard.
- Select the added NWindConnectionString as a data connection for the created model.
On the next page, specify which tables and views to include in your model. In this tutorial, the data model is based on the OrderDetail table. The code below shows the generated data model:
namespace MvcDashboardDataSources {
using System.Data.Entity;
public partial class OrderContext : DbContext {
public OrderContext()
: base("name=OrderContext") {
}
public virtual DbSet<OrderDetails> OrderDetails { get; set; }
protected override void OnModelCreating(DbModelBuilder modelBuilder) {
modelBuilder.Entity<OrderDetails>()
.Property(e => e.UnitPrice)
.HasPrecision(10, 4);
}
}
}
namespace MvcDashboardDataSources {
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
public partial class OrderDetails {
[Key]
[Column(Order = 0)]
[DatabaseGenerated(DatabaseGeneratedOption.None)]
public int OrderID { get; set; }
[Key]
[Column(Order = 1)]
[DatabaseGenerated(DatabaseGeneratedOption.None)]
public short Quantity { get; set; }
[Key]
[Column(Order = 2, TypeName = "smallmoney")]
public decimal UnitPrice { get; set; }
[Key]
[Column(Order = 3)]
public float Discount { get; set; }
[Key]
[Column(Order = 4)]
[StringLength(40)]
public string ProductName { get; set; }
[StringLength(217)]
public string Supplier { get; set; }
}
}
Imports Microsoft.VisualBasic
Imports System.Data.Entity
Namespace MvcDashboardDataSources
Partial Public Class OrderContext
Inherits DbContext
Public Sub New()
MyBase.New("name=OrderContext")
End Sub
Private privateOrderDetails As DbSet(Of OrderDetails)
Public Overridable Property OrderDetails() As DbSet(Of OrderDetails)
Get
Return privateOrderDetails
End Get
Set(ByVal value As DbSet(Of OrderDetails))
privateOrderDetails = value
End Set
End Property
Protected Overrides Sub OnModelCreating(ByVal modelBuilder As DbModelBuilder)
modelBuilder.Entity(Of OrderDetails)().Property(Function(e) e.UnitPrice).HasPrecision(10, 4)
End Sub
End Class
End Namespace
Imports Microsoft.VisualBasic
Imports System
Imports System.Collections.Generic
Imports System.ComponentModel.DataAnnotations
Imports System.ComponentModel.DataAnnotations.Schema
Imports System.Data.Entity.Spatial
Namespace MvcDashboardDataSources
Partial Public Class OrderDetails
Private privateOrderID As Integer
<Key, Column(Order := 0), DatabaseGenerated(DatabaseGeneratedOption.None)> _
Public Property OrderID() As Integer
Get
Return privateOrderID
End Get
Set(ByVal value As Integer)
privateOrderID = value
End Set
End Property
Private privateQuantity As Short
<Key, Column(Order := 1), DatabaseGenerated(DatabaseGeneratedOption.None)> _
Public Property Quantity() As Short
Get
Return privateQuantity
End Get
Set(ByVal value As Short)
privateQuantity = value
End Set
End Property
Private privateUnitPrice As Decimal
<Key, Column(Order := 2, TypeName := "smallmoney")> _
Public Property UnitPrice() As Decimal
Get
Return privateUnitPrice
End Get
Set(ByVal value As Decimal)
privateUnitPrice = value
End Set
End Property
Private privateDiscount As Single
<Key, Column(Order := 3)> _
Public Property Discount() As Single
Get
Return privateDiscount
End Get
Set(ByVal value As Single)
privateDiscount = value
End Set
End Property
Private privateProductName As String
<Key, Column(Order := 4), StringLength(40)> _
Public Property ProductName() As String
Get
Return privateProductName
End Get
Set(ByVal value As String)
privateProductName = value
End Set
End Property
Private privateSupplier As String
<StringLength(217)> _
Public Property Supplier() As String
Get
Return privateSupplier
End Get
Set(ByVal value As String)
privateSupplier = value
End Set
End Property
End Class
End Namespace
In the dashboard configuration file (for example, DashboardConfig.cs / DashboardConfig.vb), create a public method that returns the configured dashboard’s data source storage (DataSourceInMemoryStorage) and define the EF data source.
using System;
using DevExpress.DashboardCommon;
using DevExpress.DashboardWeb;
using DevExpress.DashboardWeb.Mvc;
using DevExpress.DataAccess.EntityFramework;
public static DataSourceInMemoryStorage CreateDataSourceStorage()
DataSourceInMemoryStorage dataSourceStorage = new DataSourceInMemoryStorage();
DashboardEFDataSource efDataSource = new DashboardEFDataSource("EF Data Source");
efDataSource.ConnectionParameters = new EFConnectionParameters(typeof(OrdersContext));
dataSourceStorage.RegisterDataSource("efDataSource", efDataSource.SaveToXml());
return dataSourceStorage;
}
Imports System
Imports DevExpress.DashboardCommon
Imports DevExpress.DashboardWeb
Imports DevExpress.DashboardWeb.Mvc
Imports DevExpress.DataAccess.EntityFramework
Public Shared Function CreateDataSourceStorage() As DataSourceInMemoryStorage
Dim dataSourceStorage As New DataSourceInMemoryStorage()
Dim efDataSource As New DashboardEFDataSource("EF Data Source")
efDataSource.ConnectionParameters = New EFConnectionParameters(GetType(OrdersContext))
dataSourceStorage.RegisterDataSource("efDataSource", efDataSource.SaveToXml())
Return dataSourceStorage
End Function
Call the DashboardConfigurator.SetDataSourceStorage method to configure the data source storage. Use the created CreateDataSourceStorage method as the SetDataSourceStorage parameter.
using System;
using DevExpress.DashboardWeb;
using DevExpress.DataAccess.Json;
public static void RegisterService(RouteCollection routes) {
routes.MapDashboardRoute("dashboardControl", "DefaultDashboard");
// ...
DashboardConfigurator.Default.SetDataSourceStorage(CreateDataSourceStorage());
}
Imports System
Imports DevExpress.DashboardWeb
Imports DevExpress.DataAccess.Json
Public Shared Sub RegisterService(ByVal routes As RouteCollection)
routes.MapDashboardRoute("dashboardControl", "DefaultDashboard")
' ...
DashboardConfigurator.Default.SetDataSourceStorage(CreateDataSourceStorage())
End Sub
The EF Data Source is now available in the Web Dashboard:
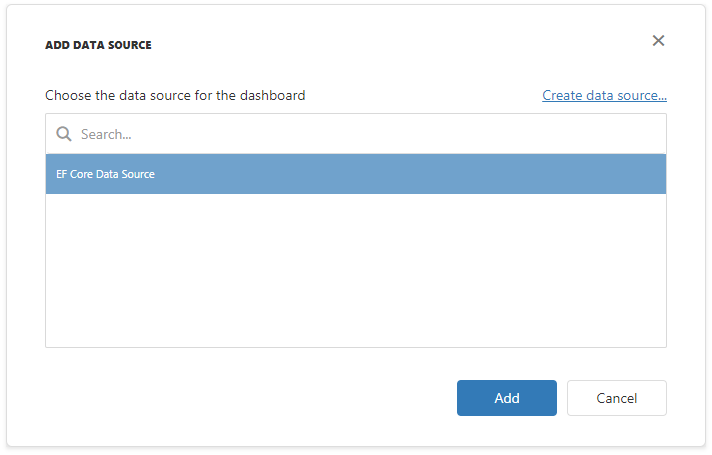
Users can now bind dashboard items to data in the Web Dashboard’s UI.
Example
The example shows how to make a set of data sources available for users in the Web Dashboard application.
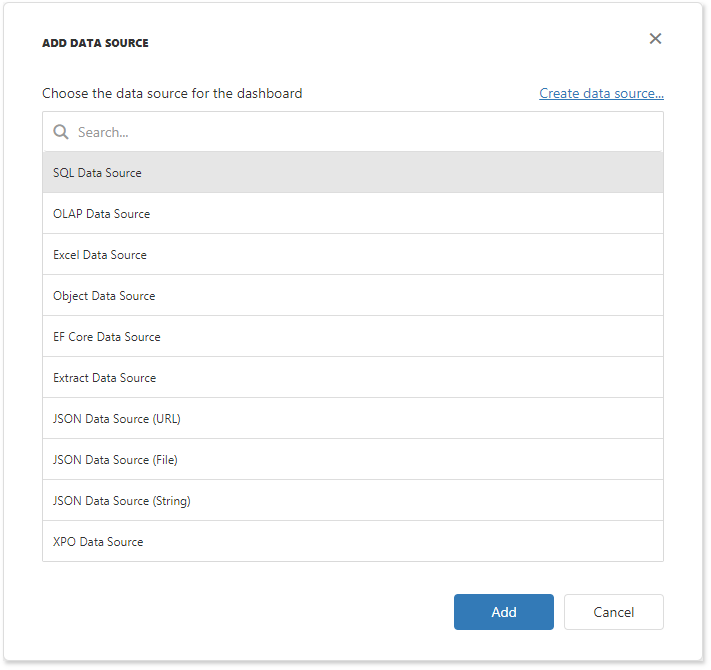
View Example: How to Register Data Sources for the ASP.NET MVC Dashboard Extension